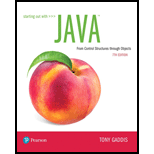
Concept explainers
// Superclass
public class Vehicle
{
private double cost;
(Other methods …)
}
// Subclass
public class Car extends Vehicle
{
public Car (double c)
{
cost = c;
}
}

Trending nowThis is a popular solution!

Chapter 10 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Degarmo's Materials And Processes In Manufacturing
Mechanics of Materials (10th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Code: interface Bicycle{ //interface for bicycle void changeGear(int val); //abstract methods void changeSpeed(int inc); void applyBrakes(int dec); void ringBell(int count); } class NewCycle implements Bicycle{ //class for a new cycle int speed=0; //stores the speed value int gear=0; //stores the gear value @Override public void changeGear(int val) { //method to change the gear gear=val; } @Override public void changeSpeed(int inc) { //method to change the speed speed=speed+inc; } @Override public void applyBrakes(int dec) { //method to apply brakes speed=speed-dec; } @Override public void ringBell(int count) { //method to ring the bell for(int i=0;i<count;i++){ System.out.print("Clang!!"+" "); } System.out.println(""); } public void printState(){ //method to print the states System.out.println("Ring bell, Speed, Gear,…arrow_forwardConver the class To UML diagram class Clint { private String accNumber; private String holderName; private long currBalance; public Clint() { currBalance = 0; } public Clint(String acc, String name, long bal) { accNumber = acc; holderName = name; currBalance = bal; } Scanner sc = new Scanner(System.in); // deposit money into the bank account by given amount amt public void deposit(long amt) { currBalance += amt; } // if amount is not given ask the user for the amount public void deposit() { System.out.print("Enter Amount(to deposit): $"); long amt = sc.nextLong(); currBalance += amt; } // withdraw money from the bank account public void withdraw(long amt) { if (currBalance >= amt) { currBalance -= amt; } else { System.out.println("Insufficient funds!"); } } // if amt is not given ask the user for the amount public…arrow_forwardWhat is the output of the following code? Convert the abstract class into an interface and modify the codes in all classes that will maintain the same output. (Rewrite the code)arrow_forward
- C# List the differences between CommissionEmployee class and BasePlusCommissionEmployee class public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; private decimal commissionRate; private decimal baseSalary; public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; CommissionRate = commissionRate; BaseSalary = baseSalary; } public decimal GrossSales { get { return grossSales; } set { if (value < 0) // validation { throw new ArgumentOutOfRangeException(nameof(value),…arrow_forwardTrue or False A subclass can override methods from its superclass.arrow_forwardinterface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forward
- public class FoodItem { private String name; private double fat; private double carbs; private double protein; // TODO: Define default constructor // TODO: Define second constructor with parameters to initialize private fields (name, fat, carbs, protein) public String getName() { return name; } public double getFat() { return fat; } public double getCarbs() { return carbs; } public double getProtein() { return protein; } public double getCalories(double numServings) { // Calorie formula double calories = ((fat * 9) + (carbs * 4) + (protein * 4)) * numServings; return calories; } public void printInfo() { System.out.println("Nutritional information per serving of " + name + ":"); System.out.printf(" Fat: %.2f g\n", fat); System.out.printf(" Carbohydrates: %.2f g\n", carbs); System.out.printf(" Protein: %.2f g\n", protein); }} import java.util.Scanner; public class NutritionalInfo {…arrow_forwardNumber 8arrow_forwardPublic Class SavingsAccount { float interest; float FixedDeposit; SeniorAccount(float interest, float FixedDeposit) { this. interest = interest; this.fixedDeposit= FixedDeposit; } float calculateInterest(); { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*interest/100); } } Public Class SeniorAccount extends SavingAccount { float seniorInterest; SeniorAccount(float interest, float FixedDeposit) { this.seniorInterest=interest; super(interest, FixedDeposit) } float calculateInterest() { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*seniorinterest/10); } } Public static void main(String args[]) { SavingsAccount saving = new SavingsAccount(6,100000); System.out.println(saving.calculateinterest()); SeniorAccount senior=new seniorAccount(10,100000); System.out.println(Senior.calculateInterest()); } Correct the syntax and logical errorarrow_forward
- Public Class SavingsAccount { float interest; float FixedDeposit; SeniorAccount(float interest, float FixedDeposit) { this. interest = interest; this.fixedDeposit= FixedDeposit; } float calculateInterest(); { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*interest/100); } } Public Class SeniorAccount extends SavingAccount { float seniorInterest; SeniorAccount(float interest, float FixedDeposit) { this.seniorInterest=interest; super(interest, FixedDeposit) } float calculateInterest() { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*seniorinterest/10); } } Public static void main(String args[]) { SavingsAccount saving = new SavingsAccount(6,100000); System.out.println(saving.calculateinterest()); SeniorAccount senior=new seniorAccount(10,100000); System.out.println(Senior.calculateInterest()); } Rubric: Correct the syntax and logical errors Proper working codearrow_forwardQUESTION 15 public class numClass { private int a; private static int y = 10; public numClass(int newX) {a=newx; } public void set(int newx) { a=newx; y+=a; } public void setY(int newy) { y=newY; } public static int getY() { return y; } }// end of class public class output { public static void main(String[] args) { numClass one = new numClass(10); numClass two = new numClass (2); try{ one. sety(30); two.set(4); if(one.getY()==30) throw new Exception ("30"); one.setY(40); } catch (Exception e) { two.sety (50); } System.out.println(two.getY()); }// end of main } // end of classarrow_forwardpublic class Person { private String personID; private String firstName; private String lastName; private String birthDate; private String address; public Person(){ personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = ""; } public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add); } public void setPerson(String id, String first, String last, String birth, String add){ personID = id; firstName = first; lastName = last; birthDate = birth; address = add; } public String getFirstName(){ return firstName; } public String getLastName(){ return lastName; } public String getBirthdate(){ return birthDate; } public String getAddress(){ return address; } public void print(){ System.out.print("\nPerson ID = " + personID); System.out.print("\nFirst Name = " +firstName); System.out.print("\nLast Name = " +lastName); System.out.print("\nBirth Date = " +birthDate); System.out.print("\nAddress = " +address); } public String…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
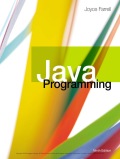
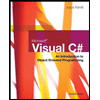
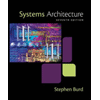