Code: interface Bicycle{ //interface for bicycle void changeGear(int val); //abstract methods void changeSpeed(int inc); void applyBrakes(int dec); void ringBell(int count);
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Code:
interface Bicycle{ //interface for bicycle
void changeGear(int val); //abstract methods
void changeSpeed(int inc);
void applyBrakes(int dec);
void ringBell(int count);
}
class NewCycle implements Bicycle{ //class for a new cycle
int speed=0; //stores the speed value
int gear=0; //stores the gear value
@Override
public void changeGear(int val) { //method to change the gear
gear=val;
}
@Override
public void changeSpeed(int inc) { //method to change the speed
speed=speed+inc;
}
@Override
public void applyBrakes(int dec) { //method to apply brakes
speed=speed-dec;
}
@Override
public void ringBell(int count) { //method to ring the bell
for(int i=0;i<count;i++){
System.out.print("Clang!!"+" ");
}
System.out.println("");
}
public void printState(){ //method to print the states
System.out.println("Ring bell, Speed, Gear, Brake, Ring Bell, Ring Bell");
}
}
public class BicycleDemo { //application class
public static void main(String args[]){ //main method
NewCycle obj=new NewCycle(); //object creation
obj.ringBell(1); //method call to ring the bell
obj.changeSpeed(3); //method call for changing the speed
System.out.println("Speed of bicycle: "+obj.speed);
obj.changeGear(2); //method call for changing the gear
System.out.println("Gear of bicycle: "+obj.gear);
obj.applyBrakes(1); //method call for applying brakes
System.out.println("Speed of bicycle: "+obj.speed);
obj.ringBell(2); //method call to ring the bell
obj.printState(); //method call for printing the states
}
}
Instructions:
Continue the above code(program):
Also:
- A subclasss of you bicycle class for a mountain bicycle, as described in the section: Classes
- Your brand comes with a water bottle (both the generic and mountain type). Add a new field that will keep the percentage of water remaining in the bottle (1 full, 0 emptty, 0.5 half empty), as described in the section: Declaring member variables. The water bottle is emptty by default.
- Declare a method to completely fill the water bottle. Declare a method to completely drink the water. No parameters needed. See: Defining methods.
- Declare a method that will specify how much to fill the water bottle, as a percentage. If the bottle has already that quantity, do nothing. The method must have the same name as above. A parameter is needed.
- Declare a method that will specify how much to drink from the water as a percentage. The method must have the same name as above. A parameter is needed.
- Create a new constructor that will specify the initial cadence, gear and water level. See: Providing Constructors for Your Classes
- Create another class, with a main method, in which you will take a trip aroound your house in your mountain bicycle, changing your bicycle attributes, starting and ending from a complete stop, and ringing the bell twice at start and once at the end. You must start with 3/4 filled water bottle, and dring some water once.
- Your trip must have at least 5 different parts in which you change your bicycle state and print its state (starting and ending from a rest state).

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

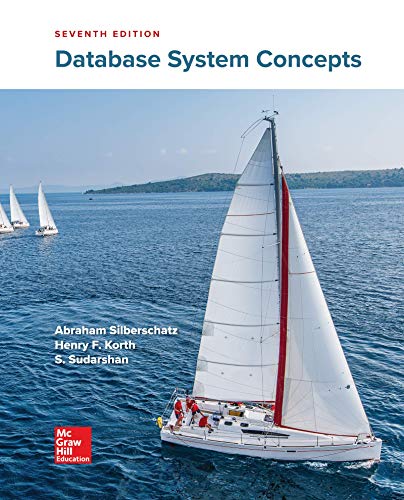
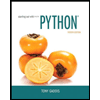
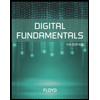
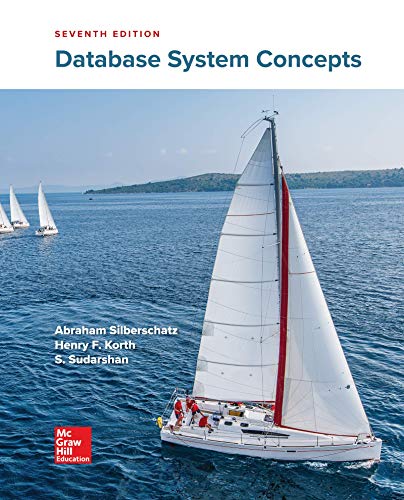
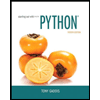
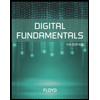
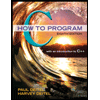
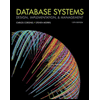
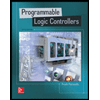