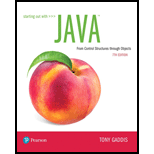
Concept explainers
Look at the following classes:
public class Ground
{
public Ground()
{
System.out.println(“You are on the ground.”);
}
public Ground(String groundColor)
{
System.out.println(“The ground is ” + groundColor);
}
}
public class Sky extends Ground
{
public Sky()
{
System.out.println(“You are in the sky.”);
}
public Sky(String skyColor)
{
super(“green”);
System.out.println(“The sky is “ + skyColor);
}
}
What will the following
public class Checkpoint
{
public static void main(String[] args)
{
Sky object = new Sky(“blue”);
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out with Java: Early Objects (6th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Database Concepts (7th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
C++ How to Program (10th Edition)
Database Concepts (8th Edition)
- public class First { public String name() { return "First"; } } public class Second extends First { public void whoRules() { System.out.print(super.name() + " rules"); System.out.printin(" but " + name() + " is even better"); } public String name() { return "Second"; } } public class Third extends Second { public String name() { return "Third"; } } Consider the following code segment. * SomeType1*/ varA = new Second(); * Some Type2*/ varB = new Third(); varA.whoRules(); varB.whoRules(); Which of the following could be used to replace /* SomeType1*/ and /* SomeType2*/ so that the code segment will compile without error?arrow_forward8. OOPS Theory #6 public class Car { public void horn() { System.out.println("Horn horn"); } } public class Ferrari extends Car { public void horn() { System.out.println("Ferarriiiiii"); } } public static void main(String[] args) { Ferrari ferrari = new Car(); ferrari.horn(); } Pick ONE option Horn horn Ferarriiii Cannot compile Runtime exception Clear Selection 9. OOPS Theory #7 In Java, we can achieve the abstraction by creating the blueprint of an object and only have abstract methods. What do we call this? Pick ONE option Interface Encapsulation Instance Protected Clear Selectionarrow_forwardpublic abstract class Shape { public Shape() { } public abstract double area() ; public String who() { return "Shape" ; } } public class Circle extends Shape { private int radius ; public Circle( int r ) { radius = r ; } @Override public double area() { return Math.PI * radius * radius ; } } public class Square extends Shape { private int _sideLength ; public Square( int sideLength ) { /* TODO question 45 */ } @Override public double area() { return _sideLength * _sideLength ; } } Implement the Square constructor, and make sure it throws an exception when the input parameter is not a valid length. The input parameter must be positive to be considered valid.arrow_forward
- public class Pet { protected String name; protected int age; public void setName(String userName) { name = userName; } public String getName() { return name; } public void setAge(int userAge) { age = userAge; } public int getAge() { return age; } public void printInfo() { System.out.println("Pet Information: "); System.out.println(" Name: " + name); System.out.println(" Age: " + age); } } import java.util.Scanner;public class PetInformation { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Pet myPet = new Pet(); Cat myCat = new Cat(); String petName, catName, catBreed; int petAge, catAge; petName = scnr.nextLine(); petAge = scnr.nextInt(); scnr.nextLine(); catName = scnr.nextLine(); catAge = scnr.nextInt(); scnr.nextLine(); catBreed = scnr.nextLine(); // TODO: Create generic pet (using petName, petAge) and then…arrow_forwardpackage debugging; public class BeanCounter {public static int beanCount = 0;} public class Bean {public Color beanColor;public String description;public Bean(Color beanColor, String description){this.beanColor = beanColor;this.description = description;}} public class Color {double[] rgb;double saturation;public Color(double[] rgb, double saturation){this.rgb = rgb;this.saturation = 0.0;}} public class Debugging { /*** @param args the command line arguments*/public static void main(String[] args) {BeanCounter bc = new BeanCounter();double[] redGreenBlue = {22.3d, 41.7d, 202.57d};Color c = new Color(rgb, 22.44+0.05, 15.78, 79.53, 252.04);++BeanCounter.beanCount;Bean b = new FavaBean(c); // New bean created!bc.beanCount++;}} 1. Remove the errors, warnings and mistakes that are in the source code. 2. Modify the code so that: •one bean is created •one bean is counted •there is only one line in the main methodarrow_forwardQuestion 13 What is outpout? public class Vehicle { public void drive(){ System.out.println("Driving vehicle"); } } public class Plane extends Vehicle { @Override public void drive(){ System.out.println("Flying plane"); } public static void main(String args[]) { Vehicle myVehicle= = new Plane(); myVehicle.drive(); } Driving vehicle syntax error Flying plane Driving vehicle Flying plane }arrow_forward
- Finish the constructor for the KickBall class. public class Ball { public double diameter; public Ball(double diam) { diameter = diam; public class KickBall extends Ball private Color ballColor; public KickBall(double diam, Color ballColor) {arrow_forwardpublic class Cylinder { public void smoothen() { } } } public abstract class Vessel implements Steerable { private Engine e; } System.out.println("Smoothening.."); public Engine getEngine() { return e; } public void setEngine (Engine e) { this.e = e; } public abstract void turnOn(); public class Engine { private Cylinder [] y; private int cyls = 1; static final int maxCylinders = 12; public void addCylinder (Cylinder y) { if (cylsarrow_forwardpublic class Secret private int x; private static int y; public static int count; public int z; public Secret () } X ?t = 2 { public Secret (int a) } { public Secret (int a, int b) } { public String toString() return ("x = " count = " + count); { public static void incrementY () { { 54. How many constructors are present in the class definition above? C. 2 d. 3 b. 1 55. What does the default constructor do in the class definition above? a. Sets the value of x to 0 c. Sets the value of x to 0 and the value of z to 1 d. There is no default constructor. b. Sets the value of z to 1 56. Based on the class definition above, which of the following statements is illegal? Secret.incrementY (); b. Secret.count++3B a. c. Secret. 2++; d. None of these 13arrow_forward// Simulates a simple car with operations to drive and check the odometer.public class SimpleCar { // Number of miles driven private int miles; public SimpleCar(){ miles = 0; } public void drive(int dist){ miles = miles + dist; } public void reverse(int dist){ miles = miles - dist; } public int getOdometer(){ return miles; } public void honkHorn(){ System.out.println("beep beep"); } public void report(){ System.out.println("Car has driven: " + miles + " miles"); } }arrow_forwardBased on Figure 3, create a class named Child that inherits the Father class. Declare an instance name location (String) for class Child. [3 marks]arrow_forwardpublic class Father { public String name; public int age; // customer name Father(){ name=”Hassan”; age=50;} public void display(){ System.out.println(“Name:”+name); System.out.println(“Age:”+age); } } 1. Based on Figure 3, create a class named Child that inherits the Father class. Declare an instance name location (String) for class Child. 2. Define a constructor in class Child and give an appropriate initial values for the instance (name, age, location). 3. Define a method display () in class Child, execute the display ( ) method in superclass using the keyword super. Method display ( ) in class Child should print the information of name, age and location 4. Create other class named Main for the main method and create 2 objects for the 2 classes (Father and Child). Then execute the display ( ) method for Father and Child. Example of the output as in Figure 4: Father's info: Name: Josh Age: 50 Child's info: Name: Christy Age: 20 Location:…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
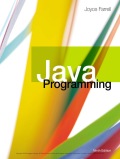