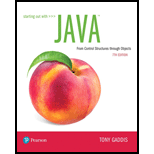
Person and Customer Classes
Design a class named Person with fields for holding a person’s name, address, and telephone number. Write one or more constructors and the appropriate mutator and accessor methods for the class’s fields.
Next, design a class named Customer, which extends the Person class. The Customer class should have a field for a customer number and a boolean field indicating whether the customer wishes to be on a mailing list. Write one or more constructors and the appropriate mutator and accessor methods for the class’s fields. Demonstrate an object of the Customer class in a simple program.

Trending nowThis is a popular solution!
Learn your wayIncludes step-by-step video

Chapter 10 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Electric Circuits. (11th Edition)
Database Concepts (8th Edition)
Concepts Of Programming Languages
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- java programming language You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is tested – create…arrow_forwardObject Oriented Programming in JAVA You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is…arrow_forward2. Car Class Write a class named car that has the following fields: ▪ yearModel. The year Model field is an int that holds the car's year model. ▪ make. The make field is a String object that holds the make of the car, such as "Ford", "Chevrolet", "Honda", etc. ▪ speed. The speed field is an int that holds the car's current speed. In addition, the class should have the following methods. ■ Constructor. The constructor should accept the car's year model and make as arguments. These values should be assigned to the object's year Model and make fields. The constructor should also assign 0 to the speed field.arrow_forward
- Design a Ship class that the following members: A field for the name of the ship (a string). A field for the year that the ship was built (a string). A constructor and appropriate accessors and mutators. A toString method that displays the ship’s name and the year it was built. Design a CruiseShip class that extends the Ship class. The CruiseShip class should have the following members: A field for the maximum number of passengers (an int). A constructor and appropriate accessors and mutators. A toString method that overrides the toString method in the base class. The CruiseShip class’s toString method should display only the ship’s name and the maximum number of passengers. Design a CargoShip class that extends the Ship class. The CargoShip class should have the following members: A field for the cargo capacity in tonnage (an int). A constructor and appropriate accessors and mutators. A toString method that overrides the toString method in the base class. The…arrow_forwardAssignment 10: Chapter 10 Complete Programming Challenge 10 at the end of chapter 10 10. Ship, CruiseShip, and CargoShip Classes Design a Ship class that the following members: A field for the name of the ship (a string) - A field for the year that the ship was built (a string). A constructor and appropriate accessors and mutators. A toString method that displays the ship's name and the year it was built. Design a CruiseShip class that extends the Ship class. The CruiseShip class should have the following members: A field for the maximum number of passengers (an int). A constructor and appropriate accessors and mutators. A toString method that overrides the toString method in the base class. The CruiseShip class's toString method should display only the ship's name and the maximum number of passengers. Design a CargoShip class that extends the Ship class. The CargoShip class should have the following members: A field for the cargo capacity in tonnage (an int). A constructor and…arrow_forwardin C# languageDesign a Book class that holds the title, author’s name, and price of the book. Books’s constructor shouldinitialize all of these data members except the price which is set to 500/-. Create a display method thatdisplays all fields.All Books are priced at 500/- unless they are PopularBooks. The PopularBooks subclass replaces theBookprice and sets each Book’s price to 50,000/- through PopularBooks construcor. Override the displaymethod to display all fields.Write a Main () method that declares an array of five Book objects. Ask the user to enter the title andauthor for each of the 5 Books. Consider the Book to be a PopularBook if the author is one of the following:Khaled Hosseini, Oscar Wilde, or Rembrandt. Display the five Books’ details.arrow_forward
- Temp Class - Create Temp class whos job is to hold a temp in degrees F and gives methods to get the temp in F, Celsius, and Kelvin. The class should follow the instance variable (field): ftemp (double type that holds F temp)Follow these methods with the class.. Constructor --Will accept Fahrenheit temperature as a double to store it in the ftemp field. setFahrenheit - The setFahrenheit method accepts a Ftemperature as a doubleto stores it in the ftemp field. getFahrenheit - Returns the value of the ftemp field, as a Fahrenheit temperature (no conversion required). getCelsius - Returns the value of the ftemp field converted to Celsius. [C e l s i u s = ( 5.0 / 9.0 ) × ( F a h r e n h e i t − 32 )] getKelvin - Returns the value of the ftemp field converted to Kelvin. [K e l v i n = ( ( 5.0 / 9.0 ) × ( F a h r e n h e i t − 32 ) ) + 273] Exemplify the temp class by writing a (test) program that is separate and promotes users for an F Fahrenheit temp. The program must create an…arrow_forwardT/F: Instance variables are shared by all the instances of the class. T/F: The scope of instance and static variables is the entire class. They can be declared anywhere inside a class. T/F: To declare static variables, constants, and methods, use the static modifier.arrow_forwardDesign a class named Pet, which should have the following fields: • name. The name field holds the name of a pet. • animal. The animal field holds the type of animal that a pet is. Example values are “Dog”, “Cat”, and “Bird”. • age. The age field holds the pet’s age. The Pet class should also have the following methods: • setName. The setName method stores a value in the name field. • setAnimal. The setAnimal method stores a value in the animal field. • setAge. The setAge method stores a value in the age field. • getName. The getName method returns the value of the name field. • getAnimal. The getAnimal method returns the value of the animal field. • getAge. The getAge method returns the value of the age field. Write the Java code for the Pet class and demonstrate it.arrow_forward
- True or false: A class may have a constructor with no parameter list, and an overloaded constructor whose parameters all take default arguments.arrow_forwardMethod,Field can be accessed from the same class to which they belong.arrow_forwardChild Class: Vegetable Write a child class called Vegetable. A vegetable is described by a name, the number of grams of sugar (as a whole number), the number of grams of sodium (as a whole number), and whether or not the vegetable is a starch. Core Class Components For the Vegetable class, write: the complete class header the instance data variables a constructor that sets the instance data variables based on parameters getters and setters; use instance data variables where appropriate a toString method that returns a text representation of a Vegetable object that includes all four characteristics of the vegetableJavaarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
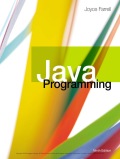
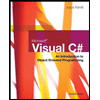
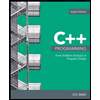