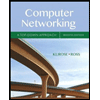
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
![**4. Consider the following classes:**
```java
public class Animal {
public Animal(String itsName) { name = itsName; }
public void setName(String itsName) { name = itsName; }
public String getName() { return name; }
private String name;
}
public class Dog extends Animal {
public Dog(String itsName) {
super(itsName + "Dog");
sound = "Bark!";
}
public void setSound(String itsSound) { sound = itsSound; }
public String getSound() { return (getName() + sound); }
public String getName(int choice) {
if(choice > 0) return super.getName();
else return "AnyDog";
}
public String getName() { return super.getName() + "OK"; }
private String sound;
}
```
**In addition, the following lines of code have been placed in a main class:**
```java
public static void main(String[] args) {
Animal a1 = new Animal("Tweety");
Animal a2 = new Dog("Rover");
Dog d1 = new Dog("Spot");
...
}
```
**Give the values of the following expressions. If an expression is erroneous, state why.**
a) `a1.getName()`
- **Output:** `Tweety`
b) `a2.getName()`
- **Output:** `RoverDogOK`
c) `a2.getName(0)`
- **Error:** Method `getName(int)` is not available in `Animal`
d) `(new Animal()).getName()`
- **Error:** Constructor `Animal` requires a `String` argument
e) `d1.getName()`
- **Output:** `SpotDogOK`
f) `d1.getName(1)`
- **Output:** `SpotDog`
g) `((Animal)d1).getName()`
- **Output:** `SpotDogOK`
h) `((Dog)a1).getName()`
- **Error:** `Animal` cannot be cast to `Dog`
i) `d1 instanceof Animal`
- **Output:** `true`
j) `a1 instanceof Dog`
- **Output:** `false`
k) `a1.getSound()`
- **Error:** Method `getSound()` is not available in `Animal`
l) `a2.get](https://content.bartleby.com/qna-images/question/b4deeaa2-280e-4afd-b08f-ce2df53e06a4/2fb714bb-3808-4232-8bb7-368df53bd141/x5swgch_thumbnail.png)
Transcribed Image Text:**4. Consider the following classes:**
```java
public class Animal {
public Animal(String itsName) { name = itsName; }
public void setName(String itsName) { name = itsName; }
public String getName() { return name; }
private String name;
}
public class Dog extends Animal {
public Dog(String itsName) {
super(itsName + "Dog");
sound = "Bark!";
}
public void setSound(String itsSound) { sound = itsSound; }
public String getSound() { return (getName() + sound); }
public String getName(int choice) {
if(choice > 0) return super.getName();
else return "AnyDog";
}
public String getName() { return super.getName() + "OK"; }
private String sound;
}
```
**In addition, the following lines of code have been placed in a main class:**
```java
public static void main(String[] args) {
Animal a1 = new Animal("Tweety");
Animal a2 = new Dog("Rover");
Dog d1 = new Dog("Spot");
...
}
```
**Give the values of the following expressions. If an expression is erroneous, state why.**
a) `a1.getName()`
- **Output:** `Tweety`
b) `a2.getName()`
- **Output:** `RoverDogOK`
c) `a2.getName(0)`
- **Error:** Method `getName(int)` is not available in `Animal`
d) `(new Animal()).getName()`
- **Error:** Constructor `Animal` requires a `String` argument
e) `d1.getName()`
- **Output:** `SpotDogOK`
f) `d1.getName(1)`
- **Output:** `SpotDog`
g) `((Animal)d1).getName()`
- **Output:** `SpotDogOK`
h) `((Dog)a1).getName()`
- **Error:** `Animal` cannot be cast to `Dog`
i) `d1 instanceof Animal`
- **Output:** `true`
j) `a1 instanceof Dog`
- **Output:** `false`
k) `a1.getSound()`
- **Error:** Method `getSound()` is not available in `Animal`
l) `a2.get
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- class smart { public: void print() const; void set(int, int); int sum(); smart(); smart(int, int); private: int x; int y; int secret(); }; class superSmart: public smart { public: void print() const; void set(int, int, int); int manipulate(); superSmart(); superSmart(int, int, int); private: int z; }; Now answer the following questions: a. Which private members, if any, of smart are public members of superSmart? b. Which members, functions, and/or data of the class smart are directly accessible in class superSmart?arrow_forwardQuestion p .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forwardFind the error(s) in the following class definition: public class TestClass private int value1; private int value2; public void TestClass(int num1, int num2) { value1= num1; value2 = num2; }arrow_forward
- Incorrect Question 4 Consider public class Species { } // fields private String name; private int population; private double growthRate; // constructor public Species () { } name = "No Name Yet"; population = 0; growthRate = 33.3; Which of the following is the best setter method for the population instance variable? public boolean setPopulation(int newPopulation) { } if (newPopulation>= 0) { } population = newPopulation; return false; } return true; public public void setPopulation (int newPopulation) if (newPopulation>= 0) { } population newPopulation; public void setPopulation (int newPopulation) { population } = newPopulation;arrow_forwardpublic class Cat extends Pet { private String breed; public void setBreed(String userBreed) { breed = userBreed; } public String getBreed() { return breed; }} public class Pet { protected String name; protected int age; public void setName(String userName) { name = userName; } public String getName() { return name; } public void setAge(int userAge) { age = userAge; } public int getAge() { return age; } public void printInfo() { System.out.println("Pet Information: "); System.out.println(" Name: " + name); System.out.println(" Age: " + age); } } import java.util.Scanner; public class PetInformation { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); // create a generic Pet and a Cat Pet myPet = new Pet(); Cat myCat = new Cat(); // declare variables for pet and cat info String petName, catName, catBreed; int petAge,…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
- Consider the following class definition: class circle { public: void print() const; void setRadius(double); void setCenter(double, double); void getCenter(double&, double&); double getRadius(); double area(); circle(); circle(double, double, double); double, double); private: double xCoordinate; double yCoordinate; double radius; } class cylinder: public circle { public: void print() const; void setHeight(double); double getHeight(); double volume(); double area(); cylinder(); cylinder(double, double, private: double height; } Suppose that you have the declaration: cylinder newCylinder; Write the definitions of the member functions of the classes circle and cylinder. Identify the member functions of the class cylinder that overrides the member functions of the class circlearrow_forwardpublic abstract class Shape { public Shape() { } public abstract double area() ; public String who() { return "Shape" ; } } public class Circle extends Shape { private int radius ; public Circle( int r ) { radius = r ; } @Override public double area() { return Math.PI * radius * radius ; } } public class Square extends Shape { private int _sideLength ; public Square( int sideLength ) { /* TODO question 45 */ } @Override public double area() { return _sideLength * _sideLength ; } } Implement the Square constructor, and make sure it throws an exception when the input parameter is not a valid length. The input parameter must be positive to be considered valid.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
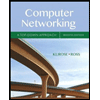
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
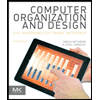
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
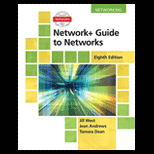
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
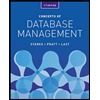
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
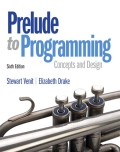
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
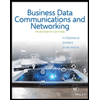
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY