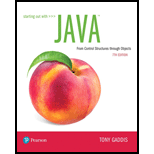
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 10, Problem 9AW
Look at the following interface:
public interface Computable
{
double compute(double x);
}
Write a statement that uses a lambda expression to create an object that implements the Computable interface. The object’s name should he half. The half object’s compute method should return the value of the x parameter divided by 2.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Refer to page 75 for graph-related problems.
Instructions:
• Implement a greedy graph coloring algorithm for the given graph.
• Demonstrate the steps to assign colors while minimizing the chromatic number.
•
Analyze the time complexity and limitations of the approach.
Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]
Refer to page 150 for problems on socket programming.
Instructions:
• Develop a client-server application using sockets to exchange messages.
•
Implement both TCP and UDP communication and highlight their differences.
• Test the program under different network conditions and analyze results.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]
Refer to page 80 for problems on white-box testing.
Instructions:
•
Perform control flow testing for the given program, drawing the control flow graph (CFG).
• Design test cases to achieve statement, branch, and path coverage.
• Justify the adequacy of your test cases using the CFG.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]
Chapter 10 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 10.1 - Here is the first line of a class declaration....Ch. 10.1 - Look at the following class declarations and...Ch. 10.1 - Class B extends class A. (Class A is the...Ch. 10.2 - Prob. 10.4CPCh. 10.2 - Look at the following classes: public class Ground...Ch. 10.3 - Under what circumstances would a subclass need to...Ch. 10.3 - How can a subclass method call an overridden...Ch. 10.3 - If a method in a subclass has the same signature...Ch. 10.3 - If a method in a subclass has the same name as a...Ch. 10.3 - Prob. 10.10CP
Ch. 10.4 - When a class member is declared as protected, what...Ch. 10.4 - What is the difference between private members and...Ch. 10.4 - Why should you avoid making class members...Ch. 10.4 - Prob. 10.14CPCh. 10.4 - Why is it easy to give package access to a class...Ch. 10.6 - Look at the following class definition: public...Ch. 10.6 - When you create a class, it automatically has a...Ch. 10.7 - Recall the Rectangle and Cube classes discussed...Ch. 10.8 - Prob. 10.19CPCh. 10.8 - If a subclass extends a superclass with an...Ch. 10.8 - What is the purpose of an abstract class?Ch. 10.8 - If a class is defined as abstract, what can you...Ch. 10.9 - Prob. 10.23CPCh. 10.9 - Prob. 10.24CPCh. 10.9 - Prob. 10.25CPCh. 10.9 - Prob. 10.26CPCh. 10.9 - Prob. 10.27CPCh. 10.9 - Prob. 10.28CPCh. 10 - In an inheritance relationship, this is the...Ch. 10 - In an inheritance relationship, this is the...Ch. 10 - This key word indicates that a class inherits from...Ch. 10 - A subclass does not have access to these...Ch. 10 - This key word refers to an objects superclass. a....Ch. 10 - In a subclass constructor, a call to the...Ch. 10 - The following is an explicit call to the...Ch. 10 - A method in a subclass that has the same signature...Ch. 10 - A method in a subclass having the same name as a...Ch. 10 - These superclass members are accessible to...Ch. 10 - Prob. 11MCCh. 10 - With this type of binding, the Java Virtual...Ch. 10 - This operator can be used to determine whether a...Ch. 10 - When a class implements an interface, it must...Ch. 10 - Prob. 15MCCh. 10 - Prob. 16MCCh. 10 - Abstract classes cannot ___________. a. be used as...Ch. 10 - You use the __________ operator to define an...Ch. 10 - Prob. 19MCCh. 10 - Prob. 20MCCh. 10 - You can use a lambda expression to instantiate an...Ch. 10 - True or False: Constructors are not inherited.Ch. 10 - True or False: in a subclass, a call to the...Ch. 10 - True or False: If a subclass constructor does not...Ch. 10 - True or False: An object of a superclass can...Ch. 10 - True or False: The superclass constructor always...Ch. 10 - True or False: When a method is declared with the...Ch. 10 - True or False: A superclass has a member with...Ch. 10 - True or False: A superclass reference variable can...Ch. 10 - True or False: A subclass reference variable can...Ch. 10 - True or False: When a class contains an abstract...Ch. 10 - True or False: A class may only implement one...Ch. 10 - True or False: By default all members of an...Ch. 10 - // Superclass public class Vehicle { (Member...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { public...Ch. 10 - Write the first line of the definition for a...Ch. 10 - Look at the following code, which is the first...Ch. 10 - Write the declaration for class B. The classs...Ch. 10 - Write the statement that calls a superclass...Ch. 10 - A superclass has the following method: public void...Ch. 10 - A superclass has the following abstract method:...Ch. 10 - Prob. 7AWCh. 10 - Prob. 8AWCh. 10 - Look at the following interface: public interface...Ch. 10 - Prob. 1SACh. 10 - A program uses two classes: Animal and Dog. Which...Ch. 10 - What is the superclass and what is the subclass in...Ch. 10 - What is the difference between a protected class...Ch. 10 - Can a subclass ever directly access the private...Ch. 10 - Which constructor is called first, that of the...Ch. 10 - What is the difference between overriding a...Ch. 10 - Prob. 8SACh. 10 - Prob. 9SACh. 10 - Prob. 10SACh. 10 - What is an. abstract class?Ch. 10 - Prob. 12SACh. 10 - When you instantiate an anonymous inner class, the...Ch. 10 - Prob. 14SACh. 10 - Prob. 15SACh. 10 - Employee and ProductionWorker Classes Design a...Ch. 10 - ShiftSupervisor Class In a particular factory, a...Ch. 10 - TeamLeader Class In a particular factory, a team...Ch. 10 - Essay Class Design an Essay class that extends the...Ch. 10 - Course Grades In a course, a teacher gives the...Ch. 10 - Analyzable Interface Modify the CourseGrades class...Ch. 10 - Person and Customer Classes Design a class named...Ch. 10 - PreferredCustomer Class A retail store has a...Ch. 10 - BankAccount and SavingsAccount Classes Design an...Ch. 10 - Ship, CruiseShip, and CargoShip Classes Design a...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
What is denormalization?
Database Concepts (8th Edition)
Assume a telephone signal travels through a cable at two-thirds the speed of light. How long does it take the s...
Electric Circuits. (11th Edition)
Write a program in a class characterFrequency that counts the number of times a digit appears in a telephone nu...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Write Java statements to accomplish each of the following tasks: Use one statement to assign the sum of x and y...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Create a flowchart that shows the necessary steps for making the cookies in the following recipe: Ingredients: ...
Starting Out With Visual Basic (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Refer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward6.3A-3. Multiple Access protocols (3). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access wireless nodes at times t=0.1, 1.4, 1.8, 3.2, 3.3, 4.1. Each transmission requires exactly one time unit. 1 t=0.0 2 3 45 t=1.0 t-2.0 t-3.0 6 t=4.0 t-5.0 For the CSMA protocol (without collision detection), indicate which packets are successfully transmitted. You should assume that it takes .2 time units for a signal to propagate from one node to each of the other nodes. You can assume that if a packet experiences a collision or senses the channel busy, then that node will not attempt a retransmission of that packet until sometime after t=5. Hint: consider propagation times carefully here. (Note: You can find more examples of problems similar to this here B.] ☐ U ப 5 - 3 1 4 6 2arrow_forward
- Just wanted to know, if you had a scene graph, how do you get multiple components from a specific scene node within a scene graph? Like if I wanted to get a component from wheel from the scene graph, does that require traversing still? Like if a physics component requires a transform component and these two component are part of the same scene node. How does the physics component knows how to get the scene object's transform it is attached to, this being in a scene graph?arrow_forwardHow to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward
- 14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
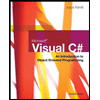
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
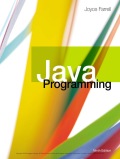
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY