What are OOPs?
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Alan Kay invented the term "Object-Oriented Programming" (OOP) while in graduate school in 1966 or 1967. The seminal Sketchpad application by Ivan Sutherland was an early source of inspiration for OOP. It was produced between 1961 and 1962 and published in 1963 as part of his Sketchpad Thesis.
Object-oriented programming (OOP) is a programming philosophy focused on the idea of "objects," which can hold data and code in the form of fields (also known as attributes or properties) and procedures (often known as methods).
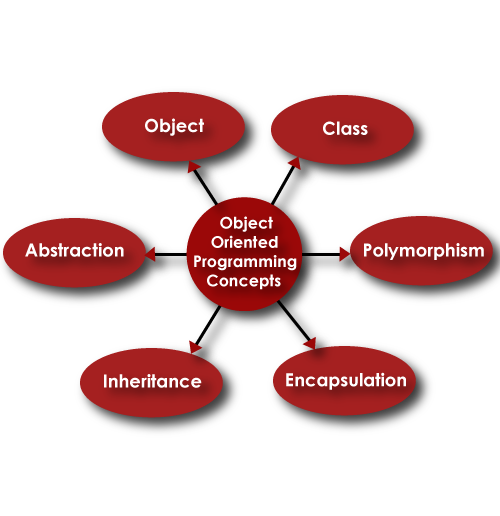
Classes & Objects
A real-world entity such as a pen, chair, table, machine, watch, etc. are referred to as an object. Object-Oriented Programming (OOP) is a programming technique or framework that uses classes and objects to construct a programme. It's used to break down a software programme into reusable code blueprints (called classes) that can be used to build individual instances of items. Object-oriented programming languages include JavaScript, C++, Java, and Python, to name a few. Kotlin is an object-oriented programming language just like Java.
Classes may also provide functions known as methods that are only applicable to objects of that type. These functions are specified within the class and perform some beneficial action to that particular object category. In an object-oriented programming language, the class is the blueprint for an object. In a programming language, the class members are accessed by creating an instance of the class.
A template description of methods and variables for a class (category of objects) that includes abstracted methods is known as an abstract class. All object-oriented programming (OOP) languages use abstract classes.
The following are some of the essential properties of a class:
- A user-defined data type is a class.
- Members of a class include data members and member functions.
- The variables of the class are the data members.
- The methods for manipulating data members are called member functions.
- The class's properties are defined by data members, while member functions define the class's behaviour.
A class may contain multiple objects, all of which share the same properties and behaviours.
Syntax
class class_name
{
data_type data_name;
return_type method_name(parameters);
}
A class's instance is an entity. It is a type of object that has properties and behaviors that are used in object-oriented programming. The entity that is generated to assign memory is known as an object. When a class is specified, it does not have its own memory chunk, which is allocated when objects are formed.
Syntax
class_name object_name;
Here's how a programmer might think about putting forward an OOP:
- Create a parent class as a blueprint of information and behaviours (methods)
- Create child classes to represent different subcategories under the generic parent blueprint.
- Add unique attributes and behaviours to the child classes to represent differences
- Create objects from the child class that represent within that subgroup
The principles of inheritance, encapsulation, abstraction, and polymorphism are all connected with object-oriented programming.
Inheritance
A class's ability to inherit or derive properties or attributes from other classes is known as Inheritance. It is very important in an object-oriented programme because it allows for reusability, i.e. using a method specified in another class through Inheritance. Child class or subclass is a class that inherits properties from another class, while base class is the class from which the properties are inherited is a base class or parent class.
“IS-A" relationship is based entirely on Inheritance, which can take two forms of Inheritance by class or Inheritance by the interface
Example The inheritance types provided by the C ++ programming language are as follows:
- Single Inheritance
- Multiple Inheritance
- Multi-level Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Abstraction
The idea of data abstraction, also known as data hiding, is to hide data and only reveal relevant data to the final user. Abstraction also has a significant security benefit. A driver only uses a few instruments, such as the gas pedal, brake, steering wheel, and blinker. The driver is not aware of the engineering. There are two ways to accomplish data abstraction in the C ++ programming language.
- Using class
- Using header file
Encapsulation
Encapsulation is the process of enclosing all important information within an object and only revealing a subset of it to the outside world. Code within the class prototype defines attributes and behaviours. Each program's various objects may attempt to interact with one another automatically. If a programmer wishes to avoid objects from communicating with one another, they must be divided into different classes. Encapsulation prevents classes from modifying or communicating with an object's unique variables and functions.
In object-oriented languages, access modifiers (or access specifiers) are keywords that govern the accessibility of classes, methods, and other members. Access modifiers are a form of programming language syntax that makes it easier to encapsulate components. Encapsulation is achieved using access modifiers, which allow you to organize the code into packages and classes and expose only the "official" public interface to the outside world while hiding the implementation information (which you want to do so that you can later change it without telling anyone).
A real-life example of encapsulation:
We have divisions for each course in our schools, such as computer science, information technology, electronics, etc. And of these departments has its own set of students and subjects to keep track of each course in our schools, such as computer science, information technology, electronics etc. These departments have their own set of students, information about the department's students, and the subjects will be taught. A department's rules are also a set of rules that students must obey throughout the course, such as timings, learning methods, etc. There are data and ways to manipulate data, and this is encapsulated in real life.
Encapsulation requires designating certain fields as private while others are made public.
- Private/ Internal interface: methods and properties, accessible from other methods of the same class.
- Public / External Interface: methods and properties, accessible also from outside the class.
Polymorphism
Polymorphism is characterized as having multiple forms. Polymorphism refers to the ability of object-oriented programming to perform tasks in various ways. The method's behaviour is determined by the form or circumstance under which it is invoked.
Let's look at an example from real life: an individual can exhibit several behaviours depending on the situation. as a mother, manager, and daughter, as a woman; as a result, her actions are described by all this. This is where the term "polymorphism" originated.
Polymorphism can be accomplished in two ways in the C++ programming language. They are operator overloading and function overloading.
- Overloading the operator when an operator is overloaded may have several behaviors in various situations.
- Overloading of functions of the same name that can perform a variety of tasks depending on a situation.
Common Mistakes
- Issues with naming,
- Exception handling,
- Deep hierarchies
- Overly optimistic development
Context & Applications
This topic is significant for both undergraduate and graduate courses, especially in Computer Science
- User interface design such as windows, menu.
- Real-Time Systems.
- Simulation and Modeling.
- Object-oriented databases.
- AI and Expert System.
- Neural Networks and parallel programming.
- Decision support and office automation systems etc.
Related Concepts
- Message Passing
- Generalization
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
OOPs Homework Questions from Fellow Students
Browse our recently answered OOPs homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.