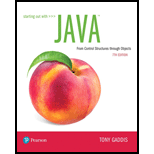
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 10.4, Problem 10.15CP
Why is it easy to give package access to a class member by accident?
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Q 3: Different packages and access modifiers
package package_02;
public class ClassB {
public int x = 1;
int y = 2;
private int z = 3;
public void myMethod() {
System.out.print(x);
System.out.print(y);
System.out.print(z);
}
}
package package_01%;
import package_02.ClassB;
public class ClassA {
public static void main(String] args) {
ClassB cb = new ClassB();
cb.myMethod();
}
}
3.1- Which statement(s) are true? Choose all that apply.
a. This code prints "123" to the output.
b. If you remove the "public" modifier from the ClassB, the code writes
"123" to the output.
c. If you remove the "public" modifier from the method myMethod, the
code prints "123" to the output.
d. This code does not compile
3.2- What do you expect if you remove the public keyword from the ClassB
(public class ClassB{..})?
PLZ help with the following IN JAVA
When defining an inner class to be a helper class for an outer class, the inner classes access should be marked as:
Public
Private
Protected
Package access
Q:-Write a statement in ClassA to check out the accessibility of your variable.
package package_02;
public class ClassB {
public int x = 1;
int y = 2;
private int z =3;
}
package package_01;
import package_02.ClassB;
public class ClassA {
public static void main(String[] args) {
ClassB cb = new ClassB();
// System.out.print(cb.x); /* 1 */
// System.out.print(cb.y); /* 2 */
// System.out.print(cb.z); /* 3 */
}
}
Chapter 10 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 10.1 - Here is the first line of a class declaration....Ch. 10.1 - Look at the following class declarations and...Ch. 10.1 - Class B extends class A. (Class A is the...Ch. 10.2 - Prob. 10.4CPCh. 10.2 - Look at the following classes: public class Ground...Ch. 10.3 - Under what circumstances would a subclass need to...Ch. 10.3 - How can a subclass method call an overridden...Ch. 10.3 - If a method in a subclass has the same signature...Ch. 10.3 - If a method in a subclass has the same name as a...Ch. 10.3 - Prob. 10.10CP
Ch. 10.4 - When a class member is declared as protected, what...Ch. 10.4 - What is the difference between private members and...Ch. 10.4 - Why should you avoid making class members...Ch. 10.4 - Prob. 10.14CPCh. 10.4 - Why is it easy to give package access to a class...Ch. 10.6 - Look at the following class definition: public...Ch. 10.6 - When you create a class, it automatically has a...Ch. 10.7 - Recall the Rectangle and Cube classes discussed...Ch. 10.8 - Prob. 10.19CPCh. 10.8 - If a subclass extends a superclass with an...Ch. 10.8 - What is the purpose of an abstract class?Ch. 10.8 - If a class is defined as abstract, what can you...Ch. 10.9 - Prob. 10.23CPCh. 10.9 - Prob. 10.24CPCh. 10.9 - Prob. 10.25CPCh. 10.9 - Prob. 10.26CPCh. 10.9 - Prob. 10.27CPCh. 10.9 - Prob. 10.28CPCh. 10 - In an inheritance relationship, this is the...Ch. 10 - In an inheritance relationship, this is the...Ch. 10 - This key word indicates that a class inherits from...Ch. 10 - A subclass does not have access to these...Ch. 10 - This key word refers to an objects superclass. a....Ch. 10 - In a subclass constructor, a call to the...Ch. 10 - The following is an explicit call to the...Ch. 10 - A method in a subclass that has the same signature...Ch. 10 - A method in a subclass having the same name as a...Ch. 10 - These superclass members are accessible to...Ch. 10 - Prob. 11MCCh. 10 - With this type of binding, the Java Virtual...Ch. 10 - This operator can be used to determine whether a...Ch. 10 - When a class implements an interface, it must...Ch. 10 - Prob. 15MCCh. 10 - Prob. 16MCCh. 10 - Abstract classes cannot ___________. a. be used as...Ch. 10 - You use the __________ operator to define an...Ch. 10 - Prob. 19MCCh. 10 - Prob. 20MCCh. 10 - You can use a lambda expression to instantiate an...Ch. 10 - True or False: Constructors are not inherited.Ch. 10 - True or False: in a subclass, a call to the...Ch. 10 - True or False: If a subclass constructor does not...Ch. 10 - True or False: An object of a superclass can...Ch. 10 - True or False: The superclass constructor always...Ch. 10 - True or False: When a method is declared with the...Ch. 10 - True or False: A superclass has a member with...Ch. 10 - True or False: A superclass reference variable can...Ch. 10 - True or False: A subclass reference variable can...Ch. 10 - True or False: When a class contains an abstract...Ch. 10 - True or False: A class may only implement one...Ch. 10 - True or False: By default all members of an...Ch. 10 - // Superclass public class Vehicle { (Member...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { public...Ch. 10 - Write the first line of the definition for a...Ch. 10 - Look at the following code, which is the first...Ch. 10 - Write the declaration for class B. The classs...Ch. 10 - Write the statement that calls a superclass...Ch. 10 - A superclass has the following method: public void...Ch. 10 - A superclass has the following abstract method:...Ch. 10 - Prob. 7AWCh. 10 - Prob. 8AWCh. 10 - Look at the following interface: public interface...Ch. 10 - Prob. 1SACh. 10 - A program uses two classes: Animal and Dog. Which...Ch. 10 - What is the superclass and what is the subclass in...Ch. 10 - What is the difference between a protected class...Ch. 10 - Can a subclass ever directly access the private...Ch. 10 - Which constructor is called first, that of the...Ch. 10 - What is the difference between overriding a...Ch. 10 - Prob. 8SACh. 10 - Prob. 9SACh. 10 - Prob. 10SACh. 10 - What is an. abstract class?Ch. 10 - Prob. 12SACh. 10 - When you instantiate an anonymous inner class, the...Ch. 10 - Prob. 14SACh. 10 - Prob. 15SACh. 10 - Employee and ProductionWorker Classes Design a...Ch. 10 - ShiftSupervisor Class In a particular factory, a...Ch. 10 - TeamLeader Class In a particular factory, a team...Ch. 10 - Essay Class Design an Essay class that extends the...Ch. 10 - Course Grades In a course, a teacher gives the...Ch. 10 - Analyzable Interface Modify the CourseGrades class...Ch. 10 - Person and Customer Classes Design a class named...Ch. 10 - PreferredCustomer Class A retail store has a...Ch. 10 - BankAccount and SavingsAccount Classes Design an...Ch. 10 - Ship, CruiseShip, and CargoShip Classes Design a...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Write a program to compute the interest due, total amount due, and the minimum payment for a revolving credit a...
Problem Solving with C++ (10th Edition)
Write an SQL query to list each customer who bought at least one product that belongs to product line Basic in ...
Modern Database Management
A 10-mm-diameter rod has a modulus of elasticity of E = 100 GPa. If it is 4 m long and subjected to an axial te...
Mechanics of Materials (10th Edition)
int a, x = 23; a = x % 2; System.out.println(x + \n + a);
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Show that for circular motion, force = mass * velocity squared/radius.
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
A loop that is inside another is called a(n) _______ loop.
Starting Out with C++ from Control Structures to Objects (9th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Take a look at the class declaration below: package p1; class Test8{ package p2; import p1.Test8; package p3; import p1.Test8; import p2.Test9; int i; class Test9 extends Test8 { private int j; protected int k; public int I; } class TestDemo3{ public static void main(String args[]){ Test8 t8 = new Test8(); Test9 t9 = new Test9(); } Out of these 4 variables declared in Test8, which are accessible in Test9 directly and by creating Test8 reference? In TestDemo3 main function, which variables can be used by each of the references t8 and t9?arrow_forward- Simple factory is not considered a design pattern because it does not decouple the client from concrete classes Select one: True False 2- structural patterns provide guidance on the way in which classes interact to distribute responsibility Select one: True False 3- The Simple factory is used when you want to limit the instantiation of classes to one implementation Select one: True False 4- When a class A is composing a reference to another class B, then we say that class A has-a class B relationship Select one: True Falsearrow_forwardTrue or False A class can implement more than one interface.arrow_forward
- Object Oriented Programming in JAVA You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is…arrow_forwardJava prgm basedarrow_forwardjava programming language You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is tested – create…arrow_forward
- Java A class always has a constructor that does not take any parameters even if there are other constructors in the class that take parameters. Choose one of the options:TrueFalsearrow_forwardAssume that a programmer wants mutual exclusion for some part of the code. the critical section is testval = testval + 1; The following code example illustrates the use of semaphore: public class Increment { private int testval = 0; Semaphore sem =new Semaphore (3); public int nextValue(){ sem.acquire(); testval = testval + 1; sem.release(); return testval; } } Will there will be race condition between threads/process in the CRITICAL SECTION? Explain your answer and correct the code above.arrow_forwardUsing c++ Design a Wacky class that can be used to generate a message based on the information passed to it. The class should have a single integer private member variable: int num; Design the complete class so that if: an object is created with no parameters; num is initialized to 10. an object is created with 1 parameter that is an integer; num is initialized to the integer that is passed *2 an object is created with 1 parameter that is a double; num is initialized to the double *4 an object is created with 2 parameters, a char and a string; it prints the message "That's WACKY!"arrow_forward
- Problem C • -3: method consonants() had more than one loop • -3: method initials () had more than one loop • -3: at least one method used nested loopsarrow_forwardSimple factory is not considered a design pattern because it does not decouple the client from concrete classes Select one: True Falsearrow_forwardHi i'm trying to learn access modifiers and i'm having trouble understanding some of them. Could you create classes and packages to test the modfiers when there's no error and when you get error. I only need help with protected and no modifier.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
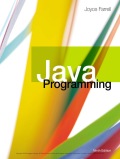
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
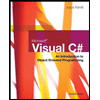
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY