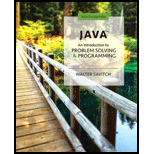
Concept explainers
Do Practice Program 5 from Chapter 5 but add a constructor that allows the user to initialize the name and alcoholic content of the beer.
Define a Beer class that contains the following instance variables with accessors/mutators:
String name; // The name of the beer
double alcohol; // The percent alcohol or the beer, e.g. // 0.05 for 5%
Add the following method:
// This method returns the number of drinks that a person
// of (weight) pounds can drink using the alcohol percentage
// in the beer, assuming a drink of 12 ounces. This is an
// estimate. The method assisted chat the legal limit is 0.08 blood
// alcohol.
public double intoxicated(double weight)
{
double numDrinks;
// This is a simplification of the Widmark formula
numDrinks = (0.08 + 0.015) + weight / (12 + 7.5 + alcohol);
return numDrinks;
}
Write code in a main method that creates two Beer objects with different alcohol percentages. Invoke the intoxicated method for a light individual and a heavy individual and output the estimated number of drinks to become legally intoxicated.

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Introduction To Programming Using Visual Basic (11th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- Java Programming problem Create a checking account class with three attributes: account number, owner’s name, and balance. Create constructor, getters and setters. If one is to set the initial balance of an account as a negative value in a setter or a constructor, remind the user about the error and set it as 0. Create a CheckingAccountDemo class, and create a main method in which Ask for the name, account number, and initial balance for one account from the keyboard input. With these input values as arguments in a call to the constructor, create a CheckingAccount object. Print the summary of this account that includes information on the name, account number, and balance by calling the getters.arrow_forwardDesign a class that holds the following personal data: name address age phone numberWrite appropriate accessor and mutator methods. Also, create a program that creates three instances of the class. One instance should hold yourinformation, and the other two should hold your friends’ or family members’information.arrow_forwardTrue or False A static method can reference an instance variable.arrow_forward
- At most, a class can contain ____________ method(S). 0 1 2 any number ofarrow_forwardIt is possible for a class to have more than one constructor.A) This is correct. B) The answer is False.arrow_forwardObject Oriented Programming in JAVA Define and use a class for the student record. The class should have instance variables for the quizzes, midterm, final, overall numeric score for the course, and final letter grade. The overall numeric score is a number in the range 0 to 100, which represents the weighted average of the student’s work. The class should have methods to compute the overall numeric grade and the final letter grade. These last methods should be void methods that set the appropriate instance variables. Your class should have a reasonable set of accessor and mutator methods, an equals method, and a toString method.arrow_forward
- Cargo Ships This question involves cargo ships that carry a specific number of cargo containers. Each ship has a maximum number of cargo containers that it can hold and a current number of cargo containers that it is holding. You can see more details in the Ship class that is provided for you. Part A For part A, you will be completing the nextToUnload() method. This takes no input and returns a Ship from the ships instance variable that has the highest percentage of its cargo hold filled (as measured by current hold / capacity). If two ships have the same percentage, then it should return the first ship with that percentage. If all of the ships in the ships array are empty (ie. no cargo), the method should return null. Part B For part B, you will complete the two unloadShip methods. The first method takes a Ship object and unloads all of the cargo from that ship. The second method takes a Ship and an int and unloads the specified number of containers from the ship. If the number of…arrow_forward2019 AP® COMPUTER SCIENCE A FREE-RESPONSE QUESTIONS 2. This question involves the implementation of a fitness tracking system that is represented by the StepTracker class. A StepTracker object is created with a parameter that defines the minimum number of steps that must be taken for a day to be considered active. The StepTracker class provides a constructor and the following methods. addDailySteps, which accumulates information about steps, in readings taken once per day activeDays, which returns the number of active days averageSteps, which returns the average number of steps per day, calculated by dividing the total number of steps taken by the number of days tracked The following table contains a sample code execution sequence and the corresponding results. Statements and Expressions Value Returned Comment (blank if no value) StepTracker tr StepTracker(10000); Days with at least 10,000 steps are considered active. Assume that the parameter is positive. new tr.activeDays () ; No…arrow_forwardThis is the tester of two classesarrow_forward
- 1- Write a Java class that models a Vehicle. There are three types of vehicles (Sedan, SUV, Truck). Every vehicle object should also contain an instance variable that can be used to store the time at which the vehicle has entered the car parking building (Hint: use the java.util.Date class to represent the time). In addition to the constructors, you should provide the following methods: public double getDuration(Date exitTime) this method will return the duration (in minutes) from the time the vehicle entered the parking until the given exitTime. public String getType() this method will return either Sedan or SUV or Truck based on the vehicle type. public void setType(String type) this method will set the type of the vehicle to either Sedan or SUV or Truck. public void setEntryTime(Date entryTime) this method will set the time at which the vehicle has entered the parking.arrow_forward• must require .extra instance variable • calculated volume again inside method getSnowVolume () • calculated snow volume again inside method getSnowCost()arrow_forwardCreate an Employee Class that will have Two instance variable: name and workingPeriod A class method named employeeByJoiningYear(): • To create an Employee object by joining year for calculating the working period o it will have two Parameter name and year • A static method experienceCheck() to check if an Employee is experienced or not o It will take working period and gender as parameter o If an employee's working period is less than 3, he or she is not experienced [You are not allowed to change the code below] # Write your code here employee1 = Employee('Dororo', 3) employee2 = Employee.employeeByJoiningYear('Harry', 2016) 6 print(employee1.workingPeriod) print(employee2.workingPeriod) print(employee1.name) print(employee2.name) print(Employee.experienceCheck(2, "male")) print(Employee.experienceCheck(3, "female")) Оutput 3 Dororo Harry He is not experienced She is experiencedarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
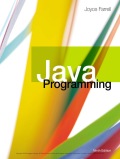
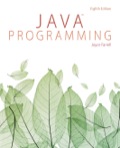
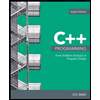
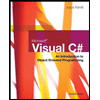