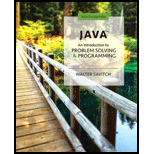
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 6, Problem 4E
Write a constructor for the class ScienceFairProjectRating, as described in Exercise 10 of the previous chapter. Give this constructor three parameters corresponding to the first three attributes that the exercise describes. The constructor should give default values to the other attributes.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I would like to know about the following concepts:
1. defragmentation
2. disk management
3. hardware RAID
Node.js, Express, and Nunjucks Templates?
CIT244 Program Project 3 Assignment
As with any assigned program, do not wait until the last minute to start. Start early in the week
the program is due so you can ask questions if you get stuck
Node.js and Express and Nunjucks Templates
We have gotten to the good stuff. There is a program similar to this assignment
given as the last example in the lecture notes for the week that discusses node
static files. This program will take more time that previous assignments.
There are several examples you should study first, particularly the pizza order
example program available in the examples programs folder for the week
discussing static files. You should study and run the pizza order program before
trying this program.
The pseudo-company is called Sun or Fun, which offers cheap flights from
Louisville to either Miami or Vegas.
Here's a video of how it should work.
NOTE: You will hear or see references to Handlebars in this video. We used to
use Handlebars, but it will be Nunjucks that we…
Chapter 6 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 6.1 - If a class is named Student, what name can you use...Ch. 6.1 - When defining a constructor, what do you specify...Ch. 6.1 - What is a default constructor?Ch. 6.1 - Does every class in Java automatically have a...Ch. 6.1 - In the program PetDemo shown in Listing 6 2, you...Ch. 6.2 - Prob. 6STQCh. 6.2 - Can a class contain both instance variables and...Ch. 6.2 - Can you reference a static variable by name within...Ch. 6.2 - Can you reference an instance variable by name...Ch. 6.2 - Can you reference a static variable by name within...
Ch. 6.2 - Can you reference an instance variable by name...Ch. 6.2 - Is the following valid, given the class...Ch. 6.2 - Prob. 13STQCh. 6.2 - Prob. 14STQCh. 6.2 - Prob. 15STQCh. 6.2 - Is the following valid, given the class...Ch. 6.2 - What values are returned by each of the following?...Ch. 6.2 - Suppose that speed is a variable of type double...Ch. 6.2 - Repeat the previous question, but instead assign...Ch. 6.2 - Suppose that nl is of type int and n2 is of type...Ch. 6.2 - Define a class CircleCalculator that hat only two...Ch. 6.2 - Which of the following statements are legal?...Ch. 6.2 - Write a Java expression to convert the number in...Ch. 6.2 - Consider the variable 5 of type String that...Ch. 6.2 - Repeat the previous question, but accommodate a...Ch. 6.2 - Write Java code to display the largest and...Ch. 6.3 - Prob. 27STQCh. 6.3 - Consider the variable allCents in the method...Ch. 6.3 - What is wrong with a program that starts as...Ch. 6.3 - Prob. 30STQCh. 6.3 - In your definition of the class OutputFormat. In...Ch. 6.4 - Prob. 32STQCh. 6.4 - Prob. 33STQCh. 6.4 - Prob. 34STQCh. 6.4 - Consider the class Species in Listing 5.19 of...Ch. 6.4 - Repeat the previous question for a method...Ch. 6.4 - Still considering the class Species in Listing...Ch. 6.4 - Rewrite the method add in Listing 6.16 so that it...Ch. 6.4 - In Listing 6.16, the set method that has a String...Ch. 6.5 - Give the definitions of three accessor methods...Ch. 6.6 - If cardSuit is an instance of Suit and is assigned...Ch. 6.7 - Suppose you want to use classes in the package...Ch. 6.7 - Prob. 43STQCh. 6.7 - Can a package have any name you might want, or are...Ch. 6.7 - On your system, place the class Pet (Listing 6.1)...Ch. 6.8 - The previous section showed you how to change the...Ch. 6 - Prob. 1ECh. 6 - Prob. 2ECh. 6 - Write a default constructor and a second...Ch. 6 - Write a constructor for the class...Ch. 6 - Consider a class characteristic that will be used...Ch. 6 - Create a class RoomOccupancy that can be used to...Ch. 6 - Write a program that tests the class RoomOccupancy...Ch. 6 - Sometimes we would like a class that has just a...Ch. 6 - Create a program that tests the class Merlin...Ch. 6 - In the previous chapter, Self-Test Question 16...Ch. 6 - Create a class Android whose objects have unique...Ch. 6 - Prob. 12ECh. 6 - Modify the definition of the class Species in...Ch. 6 - Prob. 2PCh. 6 - Using the class Pet from Listing 6.1, write a...Ch. 6 - Do Practice Program 4 from Chapter 5 except define...Ch. 6 - The following class displays a disclaimer every...Ch. 6 - Do Practice Program 5 from Chapter 5 but add a...Ch. 6 - We can improve the Beer class from the previous...Ch. 6 - Define a utility class for displaying values of...Ch. 6 - Write a new class TruncatedDollarFormat that is...Ch. 6 - Complete and fully test the class Time that...Ch. 6 - Complete and fully test the class Characteristic...Ch. 6 - Write a Java enumeration LetterGrade that...Ch. 6 - Complete and fully test the class Per n that...Ch. 6 - Write a Temperature class that represents...Ch. 6 - Repeat Programming Project 8 of the previous...Ch. 6 - Write and fully test a class that represents...Ch. 6 - Write a program that will record the votes for one...Ch. 6 - Repeat Programming Project 10 from Chapter 5, but...Ch. 6 - Create a JavaFX application that displays a button...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Porter’s competitive forces model: The model is used to provide a general view about the firms, the competitors...
Management Information Systems: Managing The Digital Firm (16th Edition)
1.1 List 10 uses. for surveying in areas other than land
sunreying-
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Why is it critical that accumulator variables are properly initialized?
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
In Exercises 41 through 46, identify the errors. Dim9WAsDouble9W=2*9WIstoutput.Items.Add(9W)
Introduction To Programming Using Visual Basic (11th Edition)
Use the following tables for your answers to questions 3.7 through 3.51 : PET_OWNER (OwnerID, OwnerLasst Name, ...
Database Concepts (8th Edition)
The acrylic plastic rod is 200 mm long and 15 mm in diameter. If an axial load of 300 N is applied to it, deter...
Mechanics of Materials (10th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please answer both Exercise 1 and2(these questions are not GRADED)arrow_forwardDiscussion 1. Comment on your results. 2. Compare between the practical and theoretical results. 3. Find VB, Vc on the figure below: 3V V₁₁ R₁ B IR, R, IR, R www ΙΚΩ www www I 1.5KQ 18₁ 82002 R₁ 3.3KQ R₂ 2.2KQ E Darrow_forwardAgile1. a. Describe it and how it differs from other SDLC approachesb. List and describe the two primary terms for the agile processc. What are the three activities in the Construction phasearrow_forward
- how are youarrow_forwardneed help with thi Next, you are going to combine everything you've learned about HTML and CSS to make a static site portfolio piece. The page should first introduce yourself. The content is up to you, but should include a variety of HTML elements, not just text. This should be followed by an online (HTML-ified) version of your CV (Resume). The following is a minimum list of requirements you should have across all your content: Both pages should start with a CSS reset (imported into your CSS, not included in your HTML) Semantic use of HTML5 sectioning elements for page structure A variety other semantic HTML elements Meaningful use of Grid, Flexbox and the Box Model as appropriate for different layout components A table An image Good use of CSS Custom Properties (variables) Non-trivial use of CSS animation Use of pseudeo elements An accessible colour palette Use of media queries The focus of this course is development, not design. However, being able to replicate a provided design…arrow_forwardUsing the notationarrow_forward
- you can select multipy optionsarrow_forwardFor each of the following, decide whether the claim is True or False and select the True ones: Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would mean that all problems in NP can also be solved in cubic time. If a problem can be solved using Dynamic Programming, then it is not NP-complete. Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time reduction from X to Y and also one from Y to X.arrow_forwardMaximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
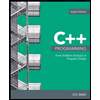
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
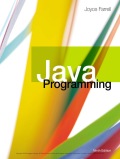
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
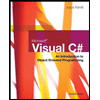
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
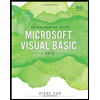
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
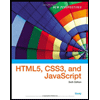
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY