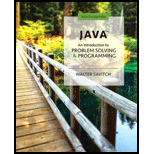
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 6.4, Problem 33STQ
Explanation of Solution
Program:
Given code:
//Define the method "countPeople()"
public static int countPeople(int numberOfCouples)
{
//Return the value
return 2 * numberOfCouples;
}
//Overload the method "countPeople()"
public static double countPeople(int numberOfCouples)
{
//Return the value
return 2...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
For the following four classes, choose the correct relationship between each pair.
public class Room (
private String m type;
private double m area;
// "Bedroom", "Dining room", etc.
// in square feet
public Room (String type, double area)
m type
type;
m area
= area;
public class Person {
private String m name;
private int m age;
public Person (String name, int age)
m name
= name;
m age = age;
public class LivingSSpace (
private String m address;
private Room[] m rooms;
private Person[] m occupants;
private int m countRooms;
private int m countoccupants;
public LivingSpace (String address, int numRooms, int
numoccupants)
m address =
address;
new int [numRooms];
= new int [numOceupants];
m rooms
%3D
D occupants
m countRooms = m countOccupants = 0;
public void addRoom (String type, double area)
//Todo write test cases for SimpleCalculator Class
// No need to implement the actual Calculator class just write Test cases as per TDD.
// you need to just write test cases no mocking // test should cover all methods from calculator and all scenarios, so a minimum of 5 test
// 1 for add, 1 for subtract, 1 for multiply, 2 for divide (1 for normal division, 1 for division by 0)
// make sure all these test cases fail
public class CalculatorTest {
//Declare variable here
private Calculator calculator;
//Add before each here
//write test cases here
}
public class PrintPrimes { private static boolean isDivisible (int i, int j) { if (j%i == 0) return true; else return false; } private static void printPrimes (int n) { int curPrime; // Value currently considered for primeness int numPrimes; // Number of primes found so far. boolean isPrime; // Is curPrime prime? int [] primes = new int [100]; // The list of prime numbers. // Initialize 2 into the list of primes. primes [0] = 2; numPrimes = 1; curPrime = 2; while (numPrimes < n) { curPrime++; // next number to consider ... isPrime = true; for (int i = 0; i <= numPrimes-1; i++) { // for each previous prime. if (isDivisible (primes[i], curPrime)) { // Found a divisor, curPrime is not prime. isPrime = false; break; // out of loop through primes. } } if (isPrime) { // save it! primes[numPrimes] = curPrime; numPrimes++; } } // End while // Print all the primes out. for (int i = 0; i <= numPrimes-1; i++) { System.out.println ("Prime: " + primes[i]); } } // end printPrimes public…
Chapter 6 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 6.1 - If a class is named Student, what name can you use...Ch. 6.1 - When defining a constructor, what do you specify...Ch. 6.1 - What is a default constructor?Ch. 6.1 - Does every class in Java automatically have a...Ch. 6.1 - In the program PetDemo shown in Listing 6 2, you...Ch. 6.2 - Prob. 6STQCh. 6.2 - Can a class contain both instance variables and...Ch. 6.2 - Can you reference a static variable by name within...Ch. 6.2 - Can you reference an instance variable by name...Ch. 6.2 - Can you reference a static variable by name within...
Ch. 6.2 - Can you reference an instance variable by name...Ch. 6.2 - Is the following valid, given the class...Ch. 6.2 - Prob. 13STQCh. 6.2 - Prob. 14STQCh. 6.2 - Prob. 15STQCh. 6.2 - Is the following valid, given the class...Ch. 6.2 - What values are returned by each of the following?...Ch. 6.2 - Suppose that speed is a variable of type double...Ch. 6.2 - Repeat the previous question, but instead assign...Ch. 6.2 - Suppose that nl is of type int and n2 is of type...Ch. 6.2 - Define a class CircleCalculator that hat only two...Ch. 6.2 - Which of the following statements are legal?...Ch. 6.2 - Write a Java expression to convert the number in...Ch. 6.2 - Consider the variable 5 of type String that...Ch. 6.2 - Repeat the previous question, but accommodate a...Ch. 6.2 - Write Java code to display the largest and...Ch. 6.3 - Prob. 27STQCh. 6.3 - Consider the variable allCents in the method...Ch. 6.3 - What is wrong with a program that starts as...Ch. 6.3 - Prob. 30STQCh. 6.3 - In your definition of the class OutputFormat. In...Ch. 6.4 - Prob. 32STQCh. 6.4 - Prob. 33STQCh. 6.4 - Prob. 34STQCh. 6.4 - Consider the class Species in Listing 5.19 of...Ch. 6.4 - Repeat the previous question for a method...Ch. 6.4 - Still considering the class Species in Listing...Ch. 6.4 - Rewrite the method add in Listing 6.16 so that it...Ch. 6.4 - In Listing 6.16, the set method that has a String...Ch. 6.5 - Give the definitions of three accessor methods...Ch. 6.6 - If cardSuit is an instance of Suit and is assigned...Ch. 6.7 - Suppose you want to use classes in the package...Ch. 6.7 - Prob. 43STQCh. 6.7 - Can a package have any name you might want, or are...Ch. 6.7 - On your system, place the class Pet (Listing 6.1)...Ch. 6.8 - The previous section showed you how to change the...Ch. 6 - Prob. 1ECh. 6 - Prob. 2ECh. 6 - Write a default constructor and a second...Ch. 6 - Write a constructor for the class...Ch. 6 - Consider a class characteristic that will be used...Ch. 6 - Create a class RoomOccupancy that can be used to...Ch. 6 - Write a program that tests the class RoomOccupancy...Ch. 6 - Sometimes we would like a class that has just a...Ch. 6 - Create a program that tests the class Merlin...Ch. 6 - In the previous chapter, Self-Test Question 16...Ch. 6 - Create a class Android whose objects have unique...Ch. 6 - Prob. 12ECh. 6 - Modify the definition of the class Species in...Ch. 6 - Prob. 2PCh. 6 - Using the class Pet from Listing 6.1, write a...Ch. 6 - Do Practice Program 4 from Chapter 5 except define...Ch. 6 - The following class displays a disclaimer every...Ch. 6 - Do Practice Program 5 from Chapter 5 but add a...Ch. 6 - We can improve the Beer class from the previous...Ch. 6 - Define a utility class for displaying values of...Ch. 6 - Write a new class TruncatedDollarFormat that is...Ch. 6 - Complete and fully test the class Time that...Ch. 6 - Complete and fully test the class Characteristic...Ch. 6 - Write a Java enumeration LetterGrade that...Ch. 6 - Complete and fully test the class Per n that...Ch. 6 - Write a Temperature class that represents...Ch. 6 - Repeat Programming Project 8 of the previous...Ch. 6 - Write and fully test a class that represents...Ch. 6 - Write a program that will record the votes for one...Ch. 6 - Repeat Programming Project 10 from Chapter 5, but...Ch. 6 - Create a JavaFX application that displays a button...
Knowledge Booster
Similar questions
- Method Overloading is a feature that allows a class to have two or more methods having same name, if their argument lists are different. Argument lists could differ in: 1. Number of parameters. 2. Data type of parameters. 3. Sequence of Data type of parameters. Write different version of method sum() that display the sum of the values received as parameter according to the following main method content: public static void main(String[] args) { sum ( 10, 10 ); sum ( 10, 10, 10 ); sum ( 10.0, 10.0 ); sum ( 10, 10.0 ); sum ( 10.0, 10); } You have to define five functions with the specified types. Then, demonstrate the Argument Promotion concept by reducing the number of method to two.arrow_forwardcomputer programmingarrow_forwardSHORT JAVA CODE only requested methods etc.arrow_forward
- True or False The versions of an overloaded method are distinguished by the number, type, and order of their parameters.arrow_forward// Sharissa Sullivan // COP 2250// Chapter 6 Method// Method with No permeters package sullivan4; import java.util.*; public class Sullivan4_2 { // Define a method for the flip of a coin - heads or tails class main { public static String toss() {// Create Random Number object for heads/tails toss Random randomNumber = new Random();// Generate a random number: heads = 0, tails = 1 int flip = randomNumber.nextInt(2);// If statement to flag flips with heads or tails if (flip == 0) return "heads"; else return "tails"; }// Define the main method public main(final String[] args) { // public static void main(String[] args)// Create Scanner object Scanner userinput = new Scanner(System.in);// Prompt the user to enter how many times they want the coin to be tossed System.out.println("How many times should I toss the coin ?");// Scan the value the user enters int scan =…arrow_forwardJAVA Write a class that calculates add, subtraction, and multiplication. The class should include the following methods: Constructor : initialize all values to 0 getAsk() : Ask users to input two integer numbers. This method calls Distribute() method. Distribute() : Ask users to choose which calculation he/she wants. This method calls a method that can add, subtract, or multiply based on the user's option. CalAdd() : add two numbers CalSub() : subtract two numbers CalProduct() : multiply two numbers toString() : toString method that display result of operation. The program should be designed to test the class developed. Your program might look like the following: public class CalculateNumTesting{ public static void main(String[] args){ CalculateNum calculation = new CalculateNum(); calculation.getAsk(); System.out.println(calculation); } }arrow_forward
- Ag 1- Random Prime Generator Add a new method to the Primes class called genRandPrime. It should take as input two int values: 1owerBound and upperBound. It should return a random prime number in between TowerBound (inclusive) and upperBound (exclusive) values. Test the functionality inside the main method. Implementation steps: a) Start by adding the method header. Remember to start with public static keywords, then the return type, method name, formal parameter list in parenthesis, and open brace. The return type will be int. We will have two formal parameters, so separate those by a comma. b) Now, add the method body code that generates a random prime number in the specified range. The simplest way to do this is to just keep trying different random numbers in the range, until we get one that is a prime. So, generate a random int using: int randNum = lowerBound + gen.nextInt(upperBound); Then enter put a while loop that will keep going while randNum is not a prime number - you can…arrow_forwardObject Oriented Programming: 213COMP, 214COMP (Feb-2022) Assignment- I [10 marks] Academic honesty: O Only pdf file accepted & student ID, will be your upload file. O Student who submit copied work will obtain a mark of zero. O Late work or attach file by email message not allowed. Q1: Write the signature for a method that has one parameter of type String, and does not return a value. Q2: Write the signature for a method that has two parameters, both of type Student, and returns an int value. Q3: Write the constructor's headers of the followings? new Student (202101156, “Ahmed"); new Address(51, "jazan university","CS&IT" ); new Grade(true, 505235600, 4.5); Q4: a) Write a class Student that define the following information: name, stid , age. b) Explain a mutators (setters) and accessors(getters) methods for each attributes(fields). c) Add three constructors: • with empty constructor. one parameter constructor (name of student) two parameters constructor (name and stid) d) Create two…arrow_forwardclass Param3 { public int x; private void increase(int p) { x = x*p; } public void calculateX(int y) { increase(y); } public int getX() { return x; } } // in another class Param3 q3 = new Param3(); q3.x = 5; q3.calculateX(7); System.out.println(q3.getX()); what would be the answer for the last two lines ? also above were x = x*p do both x in here are the fields? wouldn't that be cnofusing?arrow_forward
- java method ****just write the method ******* method heading public class VaccinationQueue { public static int prioritizeQueue(ArrayQueue<Integer> q1, ArrayQueue<Integer> q2) {arrow_forwardProblem: Construct a program that simulates the major Bank Transactions: Deposit, Withdraw and Inquire. Your program should use methods for the 3 transactions and another method for the login transaction. Below is the initial structure of the your code: public class BankTransaction { static void DisplayMenu() { /*this method displays the menu of the transactions*/ } static void withdraw(float amount) { /*this method should deduct the amount withdrawn from the current balance*/ } static void deposit(float amount) { /*this method should add the amount deposited to the current balance*/ } static float inquire() { /*this method should return the current balance*/ } static boolean CorrectPinNUmber() { /*this method should ask user to input the pin number. returns TRUE if the pin number is valid*/ It } public static void main(String a[]) { } } Design your main program to test these methods. You can design your own user interface.arrow_forwardCreate the Median class that has the method calculateMedian that calculates the median of the integers passed to the method. Use method overloading so that this method can accept anywhere from two to five parameters. CODE: import java.util.Arrays; //add class definitions below this line //add class definitions above this line public class Exercise4 { public static void main(String[] args) { //add code below this line //add code above this line }}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
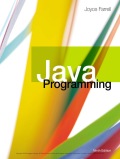
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage