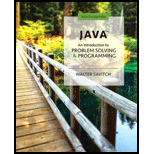
Write a Temperature class that represents temperatures in degrees in both Celsius and Fahrenheit. Use a floating-point number for the temperature and a character for the scale: either ‘C’ for Celsius or ‘F’ for Fahrenheit. The class should have
- Four constructors one for the number of decrees, one for the scale, one for both the decrees and the scale, and a default constructor. For each of these constructors, assume zero degrees if no value is specified and Celsius if no scale is given.
- Two accessory methods one to return the temperature in degrees Celsius, the other to return it in degrees Fahrenheit. Use me formulas from Practice Program 5 of Chapter 3 and round to the nearest tenth of a degree.
- Three set methods: one to set the number of degrees, one to set the scale, and one to set both.
- Three comparison methods: one to test whether two temperatures are equal, one to test whether one temperature is greater than another, and one to test whether one temperature is a less man another.
Write a driver program that tests all the methods. Be sure to invoke each of the constructors, to include at least one true and one false case for each comparison method, and to test at least the following three temperature pairs for equality; 0.0 degrees C and 32.0 degrees F, –40.0 degrees C and –40.0 degrees F, and 100.0 degrees C and 212 0 degrees F.

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
C++ How to Program (10th Edition)
Digital Fundamentals (11th Edition)
Modern Database Management (12th Edition)
Modern Database Management
- 7. Write a Temperature class that represents temperatures in degrees in both Celsius and Fahrenheit. Use a floating-point number for the temperature and a character for the scale: either 'C' for Celsius or 'F' for Fahrenheit. The class should have · Four constructors: one for the number of de- grees, one for the scale, one for both the de- grees and the scale, and a default construc- tor. For each of these constructors, assume zero degrees if no value is specified and Cel- sius if no scale is given. · Two accessor methods: one to return the temperature in degrees Celsius, the other to return it in degrees Fahrenheit. Use the for- mulas from Practice Program 5 of Chapter 3 and round to the nearest tenth of a degree. • Three set methods: one to set the number of degrees, one to set the scale, and one to set both. • Three comparison methods: one to test whether two temperatures are equal, one to test whether one temperature is greater than another, and one to test whether one temper-…arrow_forwardThis is the question - Create a class named Rock that acts as a superclass for rock samples collected and catalogued by a natural history museum. The Rock class contains the following fields: sampleNumber - of type int description - A description of the type of rock (of type String) weight - The weight of the rock in grams (of type double) Include a constructor that accepts parameters for the sample number and weight. The Rock constructor sets the description value to "Unclassified". Include get methods for each field. Create three child classes named IgneousRock, SedimentaryRock, and MetamorphicRock. The constructors for these classes require parameters for the sample number and weight. Search the Internet for a brief description of each rock type and assign it to the description field using a method named setDescription inside of the constructor. This is the code I have, the program does not like at all what I have - import java.util.*; public class DemoRock { public static…arrow_forwardDesign a Ship class that has the following members:a. A field for the name of the ship (a string).b. A field for the year that the ship was built (a string).c. A constructor and appropriate accessors and mutators.d. A toString method that displays the ship's name and the year it was built.Design a CruiseShip class that extends the Ship class. The CruiseShip class should have the following members:a. A field for the maximum number of passengers (an int).b. A constructor and appropriate accessors and mutators.c. A toString method that overrides the toString method in the base class. The CruiseShip class's toString method should display only the ship's name and the maximumnumber of passengers.Design a CargoShip class that extends the Ship class. The CargoShip class should have the following members:a. A field for the cargo capacity in tonnage (an int).b. A constructor and appropriate accessors and mutators.c. A toString method that overrides the toString method in the base class. The…arrow_forward
- This is the question - Create a class named Rock that acts as a superclass for rock samples collected and catalogued by a natural history museum. The Rock class contains the following fields: sampleNumber - of type int description - A description of the type of rock (of type String) weight - The weight of the rock in grams (of type double) Include a constructor that accepts parameters for the sample number and weight. The Rock constructor sets the description value to "Unclassified". Include get methods for each field. Create three child classes named IgneousRock, SedimentaryRock, and MetamorphicRock. The constructors for these classes require parameters for the sample number and weight. Search the Internet for a brief description of each rock type and assign it to the description field using a method named setDescription inside of the constructor. I am only missing two checks and they seem to be with the rock, here is my code then I will copy one check - import java.util.*;…arrow_forwardFor your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forwardSammy's Seashore Supplies rents beach equipment such as kayaks, canoes, beach chairs, and umbrellas to tourists. In Chapter 3, you created a Rental class for the company. The Rental class contains two public final static fields that hold the number of minutes in an hour and the hourly rental rate ($40), and four private fields that hold a contract number, number of hours for the rental, number of minutes over an hour, and the price. It also contains two public set methods and four public get methods. Now, modify the Rental class to contain two overloaded constructors. One constructor accepts a contract number and number of minutes as parameters. Pass these values to the setContractNumber() and setHoursAndMinutes() methods, respectively. The setHoursAndMinutes() method will automatically calculate the hours, extra minutes, and price. The other constructor is a default constructor that passes "A000" and 0 to the two-parameter constructor. Save the file as Rental.java. b. In Chapter…arrow_forward
- Create an Investment class that contains fields to hold the initial value of an investment, the current value, the profit (calculated as the difference between current value and initial value), and the percent profit (the profit divided by the initial value). Include a constructor with two arguments: initial, and current values and then calculate profit, and percent profit, and a display function that displays all fields. Create a House class that includes fields for street address and square feet, a constructor that requires values for both fields, and a display function. Create a HouseThatIsAnInvestment class that inherits from Investment and House. It includes a constructor (in order to pass arguments to its parent constructors) and a display function that calls the display functions of the parents. Write a main() function that declares a HouseThatIsAnInvestment and displays its values.arrow_forwardThis is the question - Write an application that uses an abstract Insurance class and Health and Life subclasses to display different types of insurance policies and the cost per month. The Insurance class contains a String representing the type of insurance and a double that holds the monthly price. The Insurance class constructor requires a String argument indicating the type of insurance, but the Life and Health class constructors require no arguments. The Insurance class contains a get method for each field; it also contains two abstract methods named setCost() and display(). The Life class setCost() method sets the monthly fee to $36, and the Health class sets the monthly fee to $196. Code I was given - import java.util.*; public class Health extends Insurance { public Health() { // write your code here } public void setCost() { // write your code here } public void display() { // write your code here } } public…arrow_forwardAn artist's discography includes several albums. Each album includes several songs. We want to model the Discography, Album and Song classes as follows • a discography has a single artist attribute and a total_time method that returns the total minutes corresponding to the artist's entire discography • an album has a single name attribute and a total_time method that returns the total minutes for the songs on the album a song has two attributes: title and duration and a duration method that returns the duration in minutes of the song • we want to take advantage of the composite pattern so that the duration method delivers the total number of minutes of a song, album or the entire discography of the artist depending on the object in question a) Draw a UML class diagram showing the solution b) Write the Ruby code that implements itarrow_forward
- in C#, Visual Basic Create a Car class that has at least the following properties:• Year• Make• Price• Passengers-holds the number of seat belts available in the car (presumably how many passengers the car can hold). This field must be private since users presumably can’t change this value. Have two constructors: one that initializes everything to some default value for the base price of a car and one that also accepts values for luxury upgrades of: heated seats, sun-roof, and satellite radio. The class has two methods: 1) Get Paint Color() which accepts a standard base color option but allows the user to purchase a pricier customized color 2) Upgrade Costs() which computes the cost of user-selected luxury items and adds it to the price. Create three car objects: Ford (make Explorer), Lexus (make ES) and a Clown Car which overrides the passenger value to allow 20 clowns in the car. The Get Paint Color() and Upgrade Costs() functions needs to be called for each object and data for all…arrow_forwardPYTHON: Define the Artist class with a constructor to initialize an artist's information and a print_info() method. The constructor should by default initialize the artist's name to "None" and the years of birth and death to 0. print_info() should display Artist Name, born XXXX if the year of death is -1 or Artist Name (XXXX-YYYY) otherwise. Define the Artwork class with a constructor to initialize an artwork's information and a print_info() method. The constructor should by default initialize the title to "None", the year created to 0, and the artist to use the Artist default constructor parameter values. Ex: If the input is: Pablo Picasso 1881 1973 Three Musicians 1921 the output is: Artist: Pablo Picasso (1881-1973) Title: Three Musicians, 1921 If the input is: Brice Marden 1938 -1 Distant Muses 2000 the output is: Artist: Brice Marden, born 1938 Title: Distant Muses, 2000 I have the code but I am getting errors that I can't seem to get rid of any suggestions would be appreciatedarrow_forwardYou are required to build a mini FunTime application for the kids to play with their electronic toys in a virtual world. Follow the given steps Build a class Toy having the following data members Name(String) Color (String) Type (String) Price (float) batteryHealth (int) Provide constructor with arguments for name, color, type, and price. Initialize batteryHealth to 5 (which means full). Provide getter for each of these but no setters.Provide a function charge(String time). This function would increase the batteryHealth of the toy according to following rules Time Increase in battery health 15 mins 20% charging (Increase health by 1) 30 mins 40% 45 mins 60% 1 hour 80% 1 hour 15 mins 100% If the toy is already fully charged, then no more charging should be done and appropriate message to be displayed. The charge function returns the new health of the battery of toy object. Provide another function play() that reduces the batteryHealth of the toy…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
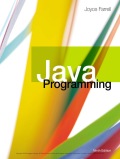
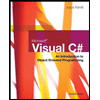
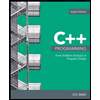