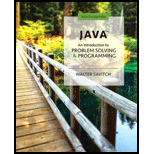
Tax computation
Program plan:
- • Define the class “TaxComputer”.
- ○ Declare the necessary constant variables.
- ○ Define the method “changeBasicRateTo()”.
- ■ Assign “newRate” into the variable “basicRate”.
- ○ Define the method “changeLuxuryRateTo()”.
- ■ Assign “newRate” into the variable “luxuryRate”.
- ○ Define the method “computeCostBasic()”.
- ■ Calculate “tax”, and “price”.
- ■ Return the value.
- ○ Define the method “computeCostLuxury()”.
- ■ Calculate “tax”, and “price”.
- ■ Return the value.
- ○ Define the method “roundToNearestPenny()”.
- ■ Calculate the price.
- ■ Return the value.
- ○ Define the “main()” function.
- ■ Print the result by calling the appropriate methods.

Program to display the tax computation of the computer.
Explanation of Solution
Program:
//Define the class
public class TaxComputer
{
//Declare the necessary constant variables
private static double basicRate = 4.0;
private static double luxuryRate = 10.0;
//Define the function changeBasicRateTo()
public static void changeBasicRateTo(double newRate)
{
/*Assign "newRate" into the variable “basicRate”*/
basicRate = newRate;
}
//Define the function changeLuxuryRateTo()
public static void changeLuxuryRateTo(double newRate)
{
/*Assign "newRate" into the variable “luxuryRate”*/
luxuryRate = newRate;
}
//Define the function computeCostBasic()
public static double computeCostBasic(double price)
{
//Calculate the “tax”
double tax = price * basicRate / 100;
//Calculate the “price”
price = price + tax;
//Return the value
return roundToNearestPenny(price);
}
//Define the function computeCostLuxury()
public static double computeCostLuxury(double price)
{
//Calculate the "tax"
double tax = price * luxuryRate / 100;
//Calculate the "price"
price = price + tax;
//Return the value
return roundToNearestPenny(price);
}
//Define the function roundToNearestPenny()
private static double roundToNearestPenny(double price)
{
//Calculate the "price"
price = price * 100;
price = java.lang.Math.round(price);
//Return the value
return price/100;
}
//Define the main() function
public static void main(String[] args)
{
//Print the statement
System.out.println("Testing the basic rate computation.");
//Print the statement
System.out.println(" Item price no tax:10.00");
//Print the statement
System.out.println("cost with 4% tax: "+ TaxComputer.computeCostBasic(10.00));
//Print the statement
System.out.println("Testing the basic rate computation.");
//Call the function changeBasicRateTo()
TaxComputer.changeBasicRateTo(7.5);
//Print the statement
System.out.println(" Item price no tax: 10.00");
//Print the statement
System.out.println("cost with 7.5% tax: "+ TaxComputer.computeCostBasic(10.00));
//Print the statement
System.out.println("Testing the luxury rate computation.");
//Print the statement
System.out.println(" Item price no tax: 2019.25");
//Print the statement
System.out.println("cost with 10% tax: "+ TaxComputer.computeCostLuxury(2019.25));
//Print the statement
System.out.println("Testing the luxury rate computation.");
//Call the function changeLuxuryRateTo()
TaxComputer.changeLuxuryRateTo(20.0);
//Print the statement
System.out.println(" Item price no tax: 2019.25");
//Print the statement
System.out.println("cost with 20% tax: "+ TaxComputer.computeCostLuxury(2019.25));
//Print the statement
System.out.println("Testing the basic rate again, should still be 7.5%.");
//Print the statement
System.out.println(" Item price no tax: 210.99");
//Print the statement
System.out.println("cost with 7.5% tax: "+ TaxComputer.computeCostBasic(210.99));
}
}
Output:
Testing the basic rate computation.
Item price no tax: 10.00
cost with 4% tax: 10.4
Testing the basic rate computation.
Item price no tax: 10.00
cost with 7.5% tax: 10.75
Testing the luxury rate computation.
Item price no tax: 2019.25
cost with 10% tax: 2221.18
Testing the luxury rate computation.
Item price no tax: 2019.25
cost with 20% tax: 2423.1
Testing the basic rate again, should still be 7.5%.
Item price no tax: 210.99
cost with 7.5% tax: 226.81
Want to see more full solutions like this?
Chapter 6 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Code In Java NetBeans Q: Create an Inventory system program which has the following classes: A) . A Date class that includes three instance variables, a month, a day, and an year. Provide a constructor that initializes the three instance variables, validating the values. Provide a toString method that displays the day, month, year separated by the forward slash(/).arrow_forwardModify the Car class example and add the following: A default constructor that initializes strings to “N/A” and numbers to zero. An overloaded constructor that takes in initial values to set your fields (6 parameters). accel() method: a void method that takes a double variable as a parameter and increases the speed of the car by that amount. brake() method: a void method that sets the speed to zero. To test your class, you will need to instantiate at least two different Car objects in the main method in the CarDemo class. Each of your objects should use a different constructor. For the object that uses the default constructor, you will need to invoke the mutator methods to assign values to your fields. Invoke the displayFeatures() method for both objects and display the variables. Demonstrate the use of the accessor method getColor() by accessing the color of each car and printing it to the console. Use the method accel to increase the speed of the first car by 65 mph. Use…arrow_forwardSlide Type Slide • Exercise # 1: Define a class Point that represents a point in 2 – D plane. The point has x and y coordinates. Define the following: • A constructor to initialize the x, y coordinates. • A method translate(self, dx,dy) to translate the point object dx , and dy units in x and y directions, respectively. • A method distanceTo (self, point2) to return the distance between the point referenced by self and point2. • getX(self) to return the value of x coordinate. • getY(self) to return the value of y coordinate Test the above class by: • Creating 2 point objects; one with (3,5) as x,y coordinates; the second with (-10,30) as x,y coordinates. • Move the first point 5.5 units in x direction and -12.5 units in y direction using translate method • Find the distance between the 2 points in their current location using distance To method A Sample output resulting from running the above test class is shown below new coordinates of point1= (8.5 , -7.5) Coordinates of point 2 =…arrow_forward
- Create a Class Pet with the following data members Identification: String species : String (e.g. cat, dog, fish etc) breed: String Age (in days): int Weight: float Dead: boolean 1. Provide a constructor with parameters for all instance variables. 2. Provide getters for all and setters for only breed, and weight 3. Provide a method growOld() that increases the age of the pet by one day. A dead pet wont grow old 4. Provide a method growHealthy(float w) that increases the weight of the pet by the given amount w. A dead pet cannot grow healthy. 5. Provide a method fallSick(float w) that reduces the weight of the pet by the given amount. The least weight a pet can have is 0 which will mean that the pet has died. If the value of weigh is 10 kg and the method is called with an argument of 11 kg then you will set it to 0 and set the dead to an appropriate value to mark the death of the pet 6. Provide a toString method that shows an appropriate well formatted string…arrow_forwardCreate a class Animal with a constructor that takes no parameters and has an instance variable: private int energy; When an animal is "born," it has one unit of energy. It has the methods: public void eat(int amountToEat) - which increases the amount of energy the animal has by amountToEat public void move(int amountToMove) - which decreases the energy the animal has by amountToMove. public int getEnergy() - which returns the amount of energy left Notice there is no setEnergy method. Energy is only changed by eating or moving. You should also create a subclass BetterAnimal which has a cap on the amount of energy an animal can have. The constructor takes a parameter that specifies a maximum for energy. You will need to save this in another instance variable. Override the eat and move methods In the eat method: If the amount the BetterAnimal eats would set its energy above the max, the energy level is only increased to the max. Also energy is only changed if the amount > 0 In…arrow_forwardJava Programming problem Create a checking account class with three attributes: account number, owner’s name, and balance. Create constructor, getters and setters. If one is to set the initial balance of an account as a negative value in a setter or a constructor, remind the user about the error and set it as 0. Create a CheckingAccountDemo class, and create a main method in which Ask for the name, account number, and initial balance for one account from the keyboard input. With these input values as arguments in a call to the constructor, create a CheckingAccount object. Print the summary of this account that includes information on the name, account number, and balance by calling the getters.arrow_forward
- Write a method toString() for the Cow class which displays information about a Cow object. The method should consider the following: • If the Cow's stomach value is 100, the output should say that the Cow is full • If the Cow's stomach value is below 100 but above 50, no information about the Cow's stomach should be given • If the Cow's stomach value is below 50 but above 10, the output should mention that the cow is hungry • If the Cow's stomach value is below 10, the output should mention that the cow is starving. Example output: First Cow is full. Second Cow Third Cow is hungry. Fourth Cow is starving!arrow_forwardJava - Constructors Create a class named Book that has the following attributes: Publisher - String Year Published - Integer Price - Double Title - String Create a constructor for the class Book that has the parameters from the attributes of the class. After, create a method for the class that displays all attributes. Ask the user for inputs for the attributes and use the constructor to create the object. Lastly, print the values of the attributes. Inputs 1. Publisher 2. Year Published 3. Price 4. Book Title XYZ Publishing 2020 1000.00 How to Win? Sample Output Published: ABC Publishing Year Published: 2022 Price: 150.00 Book Title: Stooping too low Stooping too low is published by ABC Publishing on 2022. It sells at the price of Php150.00.arrow_forwardZOOM + Press Esc to exit full screen Create a new java project named CH46EX 1. Create a class named Student 1. 2 private instance data fields – student name, student id 2. 1 final and static constant for a COLLEGE, set to "Fullerton" Create one programmer written constructor that accepts parameters for two instance data fields during instantiation Create a static method named demoStaticMethod ()-see output 3. | - print message, print college 4. Create a non-static method named demoObjectMethod ()– see output - print message, college, student name, student id 2. Create an application named StudentDemo 1. Create/instantiate 1 student object 2. Call each method correctly This demo is a static method Fullerton 3. Expected Output > This demo is a non-static method Fullerton Name: Wyle Coyote ID: 456arrow_forward
- In java language on netbeans design a console application that will print the game sales for a particular game in 2021. Use an abstract class named Games that contains variables to store the game name, game genre and the total sales. Create a constructor that accepts the game name, game genre and the total game sales as parameters. In this class also create get methods for the variables. The Games class must implement an iGames interface that contains the following: public interface iGames { public void printSales(); public String getGame(); public String getGameGenre(); public int getGameSales(); } Create a subclass called Game Sales that extends the Games class. The Games Sales class must contain a constructor to accept the game name, game genre and total game sales as parameters. Then, write code for the Print Sales method, which prints the game sales report for 2021. Finally, write a Use Games class to instantiate the Games Sales class.arrow_forward1. Write an app that computes student average2. Create a class called Student3. Use the init () function to collect the student information: name, math, science, and English grade4. Create a method (class function) that computes the average5. Create another method that outputs (similarly to) the ff when calledName: John Math: 90Science: 90English: 90Average: 90 (Passed)6. Instantiate a Student object and utilize the defined methods***Let the user input that student infoarrow_forwardCreate a RightTriangle class given the following design: RightTriangle variables: base, height methods: 2 constructors, 1 that set default values and another that accepts to parameters. setBase - changes the base. Requires one parameter for base. setHeight - changes the height. Requires one parameter for height. getBase - returns the triangle base. getHeight - returns the triangle height. area - returns the area of the triangle (1/2bh) given the current base and heightarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
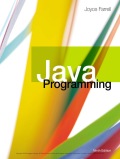