Implement the following class MyPhoneBook. You can add additional private member variables and functions. The class has the following private member variables: 1- names and phones: Dynamic arrays of strings. 2- phoneBookSize: An int that holds size of phonebook. The Class has the following public member functions: 1- Parametrized Constructor that takes the size of PhoneBook and allocate dynamic arrays of names and phones and a copy constructor to initialize a PhoneBook using another PhoneBook. 2- A function addEntry that adds a name and phone number at the first empty space at corresponding arrays, and returns true if entry is added, false otherwise. An entry is added if there's space in the array and if phone number if valid. It checks for the validity of the phone number by ensuring that it has 11 digits, and doesn't include any alphabets or special characters. 3- A function displayEntryAtIndex(int) that displays a name and phone number at the specified index. It returns true if the index is in range, false otherwise. 4- A function displayEntryAtIndices(int*) that receives an array of zeros and ones and has the same size of the class arrays. The function displays the name and phone number of an entry in the array only if the corresponding integer in the parameter array is one.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
object oriented
class decleration:
class MyPhoneBook
{
string* names;
string* phones;
int phoneBookSize;
public:
MyPhoneBook(int); //Takes size
MyPhoneBook(const MyPhoneBook&); //Copy Constructor
bool addEntry(string ,string);
bool displayEntryAtIndex(int);
void displayEntryAtIndices(int*);
void displayAll();
int* findByName(string);
int* findByPhone(string);
bool updateNameAt(string, int);
bool updatePhoneAt(string, int);
~MyPhoneBook();
};



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

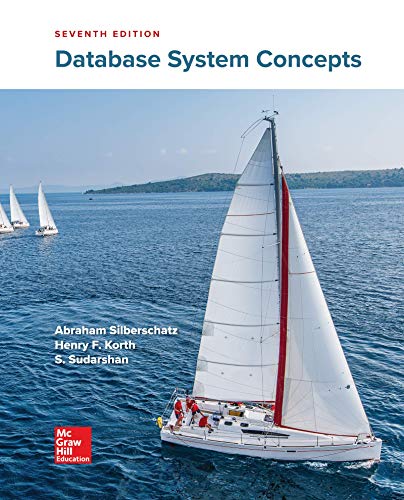
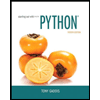
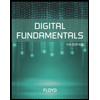
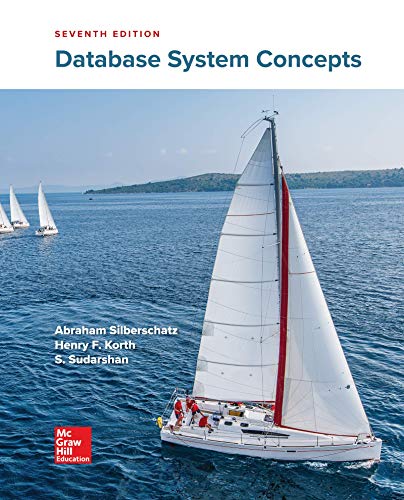
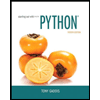
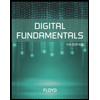
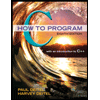
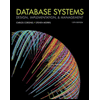
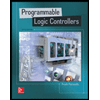