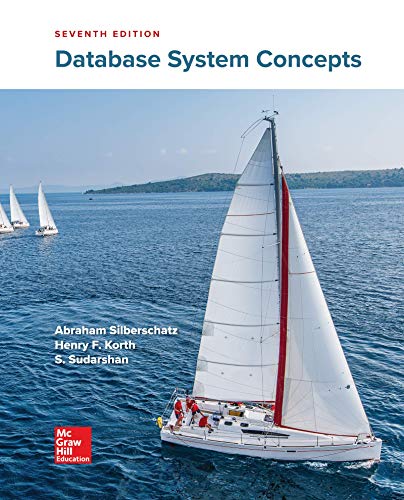
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
#include <iostream>
#include <string>
#include <cstdlib>
using namespace std;
template<class T>
class A
{
public:
A(){
cout<<"Created\n";
}
~A(){
cout<<"Destroyed\n";
}
};
int main(int argc, char const *argv[])
{
A <int>a;
return 0;
}
Give output for this code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- #include <iostream>#include <string>#include <cstdlib>using namespace std;template<class T>class A{public:A(){cout<<"Created\n";}~A(){cout<<"Destroyed\n";}}; int main(int argc, char const *argv[]){A <int>a1;A <char>a2;A <float>a3;return 0;} Give output for this codearrow_forward#include #include using std::cout; using std::vector; class StatSet { private: HCreate a vector of doubles that is called "numbers." public: //Nothing to do in the constructor. StatSet() } // Add a new number to the set. vòid add_num(double num) { } double mean() { //Sum up the values and return the average. double sum = 0; //Write a loop that would iterate through the vector. sum += numbers[i]; return sum / numbers.size(); } double median() //Before calculating the median, we must sort the //array.arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forward
- C++ Define an "Expression" class that manages expression info: operand1 (integer), operand2 (integer), and the expression operator (char). It must provide at least the following method:- toString() to return a string containing all the expression info such as 50 + 50 = 100 Write a command-driven program named "ListExpressions" that will accept the following commands:add, listall, listbyoperator, listsummary, exit "add" command will read the expression and save it away in the list "listall" command will display all the expressions "listbyoperator" command will read in the operator and display only expressions with that given operator "listsummary" command will display the total number of expressions, the number of expressions for each operator, the largest and smallest expression values "exit" command will exit the program Requirements:- It must be using the array of pointers (not vector) to Expression objects to manage the list of Expression…arrow_forward#include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forward
- C++ Given code #include <iostream>using namespace std; class Node {public:int data;Node *pNext;}; void displayNumberValues( Node *pHead){while( pHead != NULL) {cout << pHead->data << " ";pHead = pHead->pNext;}cout << endl;} //Option 1: Search the list// TODO: complete the function below to search for a given value in linked lsit// return true if value exists in the list, return false otherwise. ?? linkedlistSearch( ???){ } //Option 2: get sum of all values// TODO: complete the function below to return the sum of all elements in the linked list. ??? getSumOfAllNumbers( ???){ } int main(){int userInput;Node *pHead = NULL;Node *pTemp;cout<<"Enter list numbers separated by space, followed by -1: "; cin >> userInput;// Keep looping until end of input flag of -1 is givenwhile( userInput != -1) {// Store this number on the listpTemp = new Node;pTemp->data = userInput;pTemp->pNext = pHead;pHead = pTemp;cin >> userInput;}cout <<"…arrow_forward/* Interface File: Movie.h Declaration of Movie Class (Variables - "Data Members" or "Attributes" AND Functions - "Member Functions" or "Methods") */ #ifndef MOVIE_H // Include Guard or Header Guard -If already defined ignore rest of code #define MOVIE_H // Otherwise, define MOVIE_H #include<string> // Note: Not "using namespace std;" or even "using std::string" class Movie { private: std::string title = ""; // Explict scope used --> std::string int year = 0; public: Movie(std::string title = "", int year = 1888); // Declaring a Default Constructor ~Movie(); // A Destructor used for freeing up resources void set_title(std::string title_param); std::string get_title() const; // "const" safeguards class variable changes within function std::string get_title_upper() const; void set_year(int year_param); int get_year() const; }; //…arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> T func(T a) { cout<<a; return a; } template<class U> void func(U a) { cout<<a; } int main(int argc, char const *argv[]) { int a = 5; int b = func(a); return 0; } Give output for this code.arrow_forward
- C++ Define an "Expression" class that manages expression info: operand1 (integer), operand2 (integer), and the expression operator (char). It must provide at least the following method:- toString() to return a string containing all the expression info such as 50 + 50 = 100 Write a command-driven program named "ListExpressions" that will accept the following commands:add, listall, listbyoperator, listsummary, exit "add" command will read the expression and save it away in the list "listall" command will display all the expressions "listbyoperator" command will read in the operator and display only expressions with that given operator "listsummary" command will display the total number of expressions, the number of expressions for each operator, the largest and smallest expression values "exit" command will exit the program Requirements:- It must be using the array of pointers to Expression objects to manage the list of Expression objects. Please…arrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forwardgetting error please helparrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
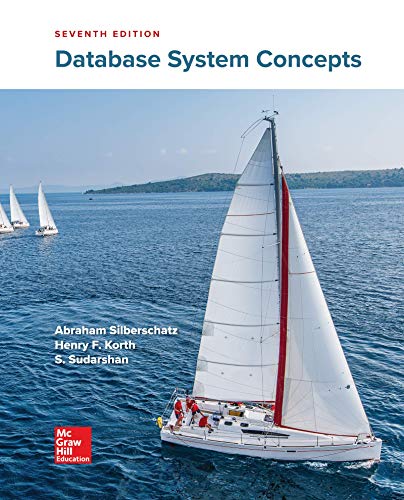
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
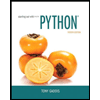
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
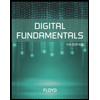
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
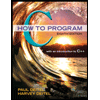
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
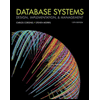
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
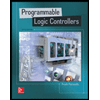
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education