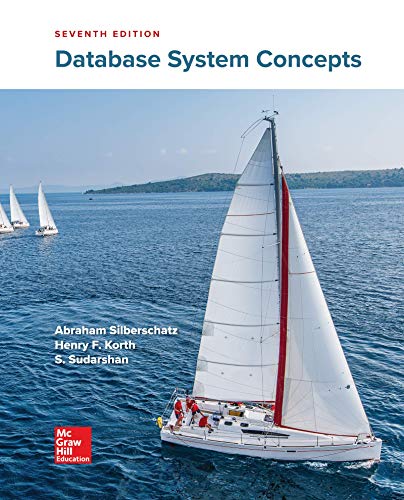
Concept explainers
#include <bits/stdc++.h>
#include<unordered_map>
#include <string>
using namespace std;
struct Student{
string firstName;
string lastName;
string studentId;
double gpa;
};
class MyClass{
public:
unordered_map<string, int>
classHoursInfo;
unordered_map<string, int> creditValue = {{"A", 4}, {"B", 3}, {"C", 2}, {"D", 1}, {"F", 0}};
void readStudentData(string filepath){
cout << "reading student data \n";
ifstream inpStream(filepath);
string text;
string tmp[4];
while (getline (inpStream, text)) {
string str = "", prevKey = ""; int j= 0;
unordered_map<string, int> curStudentClassInfo;
for(int i=0; i<text.length(); i++){
if(isspace(text[i])){
if(j>3){
if(prevKey.length()==0){
curStudentClassInfo[str] = 0;
prevKey = str;
str.clear();
}
else{
curStudentClassInfo[prevKey] = creditValue[str];
prevKey = "";
str.clear();
}
}
else{
tmp[j++] = str;
str.clear();
}
}
else{
str = str+text[i];
}
}
if(str.length() != 0){
curStudentClassInfo[prevKey] = creditValue[str];
}
double curGPA = gpacalculation(curStudentClassInfo, classHoursInfo);
students.push_back({tmp[0], tmp[1], tmp[2], curGPA});
}
inpStream.close();
}
};
double gpacalculation(unordered_map < string,int> studentData, unordered_map<string,int> creditData);{
cout << "calculating GPA of a student \n"
int sumOfCreditHours = 0;
int prod = 0;
for (auto &x : studentData) {
sumOfCreditHours += creditData[,first];
prod += ((x.second)*(creditData[x,first]));
}
return prod/(sumOfCreditHours+0.0);
}
void readCreditData(string filepath){
cout << "reading credit data \n";
ifstream inpStream(filepath);
string text;
string tmp[2];
while (getline (inpStream, text)) {
string str = ""; int j= 0;
for(int i=0; i<text.length(); i++){
if(isspace(text[i])){
tmp[j++] = str;
str.clear();
}
else{
str = str+text[i];
}
}
if(j!=2) tmp[1] = str;
classHoursInfo[tmp(0)] = stoi(tmp(1));
}
inpStream.close();
}
void writeToFile(string filepath){
cout << "writing students GPA to ouput file and printing in console \n";
ofstream outStream(filepath);
for(Student s: students){
string data = s.firstName+" "+s.lastName+" "+s.studentId+" "+to_string(s.gpa)+"\n";
outStream<<data;
cout<<data;
}
outStream.close();
}
};
int main() {
MyClass c;
c.readCreditData("Credit.txt");
c.readStudentData("Student.txt");
c.writeToFile("StudentsGPA.txt");
}
Above is the code i have written but i am having 2 error which i have posted the images for it
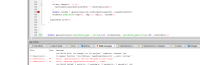

1st error: You close the class before the gpacalculation function so that’s why code shows the error.
2nd error: you close the class before int main() and also close the class before gpaclaculation() that’s why it shows this ‘{‘ error.
I have closed the class after writetofile() function this resolved both errors.
Step by stepSolved in 2 steps with 1 images

- namespace CS3358_FA2022_A04_sequenceOfNum{template <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;bool is_item();};} error: invalid use of template-name 'CS3358_FA2022_A04_sequenceOfNum::sequence' without an argument list 120 | sequence::size_type sequence<Item>::size() const { | ^~~~~~~~arrow_forwardstruct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward
- #ifndef LLCP_INT_H#define LLCP_INT_H #include <iostream> struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endifarrow_forward// FILE: DPQueue.h// CLASS PROVIDED: p_queue (priority queue ADT)//// TYPEDEFS and MEMBER CONSTANTS for the p_queue class:// typedef _____ value_type// p_queue::value_type is the data type of the items in// the p_queue. It may be any of the C++ built-in types// (int, char, etc.), or a class with a default constructor, a// copy constructor, an assignment operator, and a less-than// operator forming a strict weak ordering.//// typedef _____ size_type// p_queue::size_type is the data type considered best-suited// for any variable meant for counting and sizing (as well as// array-indexing) purposes; e.g.: it is the data type for a// variable representing how many items are in the p_queue.// It is also the data type of the priority associated with// each item in the p_queue//// static const size_type DEFAULT_CAPACITY = _____// p_queue::DEFAULT_CAPACITY is the default initial capacity of a// p_queue that is created by the default…arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forward
- #include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forwardC:/Users/r1821655/CLionProjects/untitled/sequence.cpp:48:5: error: return type specification for constructor invalidtemplate <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;};} 48 | void sequence<Item>::sequence() : used(0), current_index(0) { } | ^~~~ line 47 template<class Item> line 48 void sequence<Item>::sequence() : used(0), current_index(0) { }arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward
- Instructions: Turn all instances of classes into pointers. You will also need to combine the player and vector into one vector objects and fix all issues this causes. #ifndef ITEM_H #define ITEM_H class Item { public: Item() {}; enum class Type { sword, armor, shield, numTypes }; Item(Type classification, int bonusValue); Type getClassification() const; int getBonusValue() const; private: Type classification{ Type::numTypes }; int bonusValue{ 0 }; }; std::ostream& operator<< (std::ostream& o, const Item& src); bool operator< (const Item& srcL, const Item& srcR); int& operator+=(int& srcL, const Item& srcR); #endif // !ITEM_H #ifndef MONSTER_H #define MONSTER_H #include "Object.h" class Player; class Monster : public Object { public: Monster() {}; Monster(const Player& player); void update(Player& player, std::vector& monsters) override; int attack() const override; void defend(int damage) override; void print(std::ostream& o)…arrow_forward#ifndef NODES_LLOLL_H#define NODES_LLOLL_H #include <iostream> // for ostream namespace CS3358_SP2023_A5P2{ // child node struct CNode { int data; CNode* link; }; // parent node struct PNode { CNode* data; PNode* link; }; // toolkit functions for LLoLL based on above node definitions void Destroy_cList(CNode*& cListHead); void Destroy_pList(PNode*& pListHead); void ShowAll_DF(PNode* pListHead, std::ostream& outs); void ShowAll_BF(PNode* pListHead, std::ostream& outs);} #endif #include "nodes_LLoLL.h"#include "cnPtrQueue.h"#include <iostream>using namespace std; namespace CS3358_SP2023_A5P2{ // do breadth-first (level) traversal and print data void ShowAll_BF(PNode* pListHead, ostream& outs) { cnPtrQueue queue; CNode* currentNode; queue.push(lloLLPtr->getHead()); while (!queue.empty()) { currentNode = queue.front(); queue.pop(); if…arrow_forwardDesign a struct with following members: p_num_lots, int* type pa_lots, int [] type (dynamically allocated) Implement following methods: parameterized constructor which takes an int for num of lots copy constructor copy assignment operator destructorarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
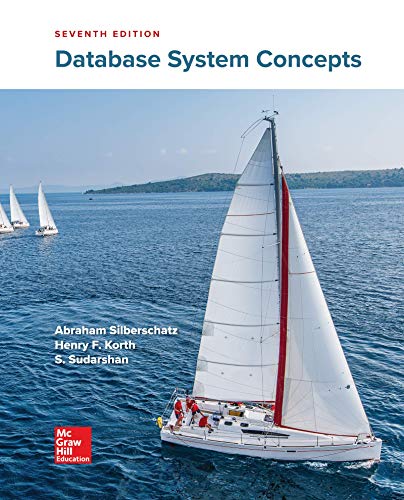
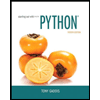
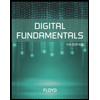
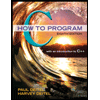
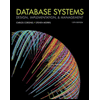
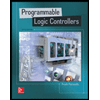