C++ Given Code #include #include #include using namespace std; // The puzzle will always have exactly 20 columns const int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logic void searchPuzzle(const char puzzle[][numCols], const string wordBank[], vector &discovered, int numRows, int numWords); // Printer function that outputs a vector void printVector(const vector &v); // Example of one potential helper function. // bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word, // int rowStart, int colStart) int main() { int numRows, numWords; // grab the array row dimension and amount of words cin >> numRows >> numWords; // declare a 2D array char puzzle[numRows][numCols]; // TODO: fill the 2D array via input // read the puzzle in from the input file using cin // create a 1D array for wods string wordBank[numWords]; // TODO: fill the wordBank through input using cin // set up discovery vector vector discovered; // Search for Words searchPuzzle(puzzle, wordBank, discovered, numRows, numWords); // Sort the results sort(discovered.begin(), discovered.end()); // Print vector of discovered words printVector(discovered); return 0; } // TODO: implement searchPuzzle and any helper functions you want void searchPuzzle(const char puzzle[][numCols], const string wordBank[], vector &discovered, int numRows, int numWords) { } // TODO: implement printVector void printVector(const vector &v) { }
C++ Given Code
#include <
#include <iostream>
#include <algorithm>
using namespace std;
// The puzzle will always have exactly 20 columns
const int numCols = 20;
// Searches the entire puzzle, but may use helper functions to implement logic
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords);
// Printer function that outputs a vector
void printVector(const vector <string> &v);
// Example of one potential helper function.
// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,
// int rowStart, int colStart)
int main()
{
int numRows, numWords;
// grab the array row dimension and amount of words
cin >> numRows >> numWords;
// declare a 2D array
char puzzle[numRows][numCols];
// TODO: fill the 2D array via input
// read the puzzle in from the input file using cin
// create a 1D array for wods
string wordBank[numWords];
// TODO: fill the wordBank through input using cin
// set up discovery vector
vector <string> discovered;
// Search for Words
searchPuzzle(puzzle, wordBank, discovered, numRows, numWords);
// Sort the results
sort(discovered.begin(), discovered.end());
// Print vector of discovered words
printVector(discovered);
return 0;
}
// TODO: implement searchPuzzle and any helper functions you want
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords)
{
}
// TODO: implement printVector
void printVector(const vector <string> &v)
{
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

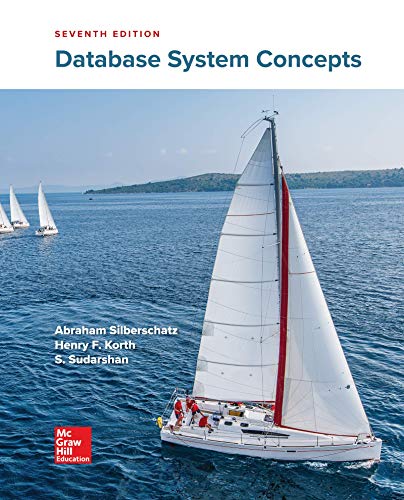
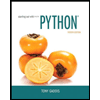
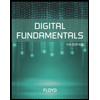
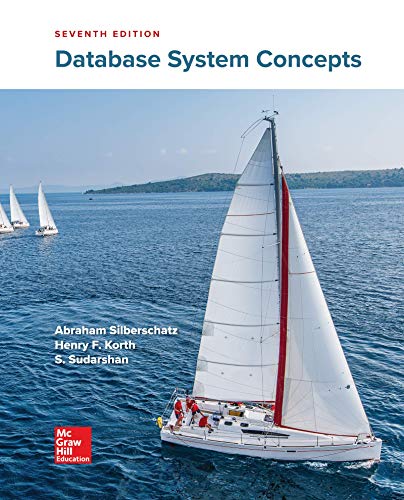
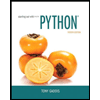
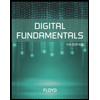
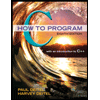
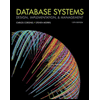
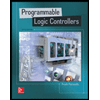