/* Interface File: Movie.h Declaration of Movie Class (Variables - "Data Members" or "Attributes" AND Functions - "Member Functions" or "Methods") */ #ifndef MOVIE_H // Include Guard or Header Guard -If already defined ignore rest of code #define MOVIE_H // Otherwise, define MOVIE_H #include // Note: Not "using namespace std;" or even "using std::string" class Movie { private: std::string title = ""; // Explict scope used --> std::string int year = 0; public: Movie(std::string title = "", int year = 1888); // Declaring a Default Constructor ~Movie(); // A Destructor used for freeing up resources void set_title(std::string title_param); std::string get_title() const; // "const" safeguards class variable changes within function std::string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! #endif // MOVIE_H /* Implementation file: Movie.cpp Implements the functions declared in the interface file (Movie.h) */ #include #include "Movie.h" // This code only needs "using" to enable the use of "string". Other programs would have a longer list here! using std::string; Movie::Movie(string title, int year) // Constructor definition { set_title(title); set_year(year); } ~Movie::Movie() // Destructor definition { CODE FOR FREEING UP RESOURCES } void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } /* Main file: Movie_List.cpp Utilizes Class Movie */ #include #include #include #include #include "Movie.h" // You need to replace "using namespace std;" with "using" declarations (see Movie.cpp as an example) using namespace std; // Replace this with "using std::cout", etc. int main() { cout << "The Movie List program\n\n" << "Enter a movie...\n\n"; // get vector of Movie objects vector movies; char another = 'y'; while (tolower(another) == 'y') { Movie movie; // movie is initialized with the Default Constructor values string title; cout << "Title: "; getline(cin, title); movie.set_title(title); int year; cout << "Year: "; cin >> year; movie.set_year(year); movies.push_back(movie); cout << "\nEnter another movie? (y/n): "; cin >> another; cin.ignore(); cout << endl; } // display the movies const int w = 10; cout << left << setw(w * 3) << "TITLE" << setw(w) << "YEAR" << endl; for (Movie movie : movies) { cout << setw(w * 3) << movie.get_title() << setw(w) << movie.get_year() << endl; } cout << endl; // Output with titles in ALL CAPS for (Movie movie : movies) { cout << setw(w * 3) << movie.get_title_upper() << setw(w) << movie.get_year() << endl; } cout << endl; return 0; } Modify the code above to create a "Favorite Video Game List" program that is contained in three files: Game.h Game.cpp Game_List.cpp You will need do to these things as you modify the code and put it into the three files: Create a class called "Game" (instead of "Movie") that contains 3 private variables ("title", "year", and "rating") The "year" is when the game first came out. The "rating" should be from 1 to 5 (5 being the best). Add the appropriate member functions of the class (setters and getters). Continue to use the get_title_upper() function as part of the class, Game, and in the output within the main file, Game_List.cpp. Add the Default Constructor and Destructor for the class with the member functions of the class. Don't forget to add the "include guard" to the .h file Use the scope resolution operator correctly for each file. Don't use the "using namespace std;" for the main file, Game_List.cpp. Instead, use the "using" declaration using std::cout; using std::endl; using std::string; etc.
/*
Interface File: Movie.h
Declaration of Movie Class
(Variables - "Data Members" or "Attributes"
AND Functions - "Member Functions" or "Methods")
*/
#ifndef MOVIE_H // Include Guard or Header Guard -If already defined ignore rest of code
#define MOVIE_H // Otherwise, define MOVIE_H
#include<string>
// Note: Not "using namespace std;" or even "using std::string"
class Movie
{
private:
std::string title = ""; // Explict scope used --> std::string
int year = 0;
public:
Movie(std::string title = "", int year = 1888); // Declaring a Default Constructor
~Movie(); // A Destructor used for freeing up resources
void set_title(std::string title_param);
std::string get_title() const; // "const" safeguards class variable changes within function
std::string get_title_upper() const;
void set_year(int year_param);
int get_year() const;
}; // NOTICE: Class declaration ends with semicolon!
#endif // MOVIE_H
/*
Implementation file: Movie.cpp
Implements the functions declared in the interface file (Movie.h)
*/
#include <string>
#include "Movie.h"
// This code only needs "using" to enable the use of "string". Other programs would have a longer list here!
using std::string;
Movie::Movie(string title, int year) // Constructor definition
{
set_title(title);
set_year(year);
}
~Movie::Movie() // Destructor definition
{
CODE FOR FREEING UP RESOURCES
}
void Movie::set_title(string title_param)
{
title = title_param;
}
string Movie::get_title() const
{
return title;
}
string Movie::get_title_upper() const
{
string title_upper;
for (char c : title) {
title_upper.push_back(toupper(c));
}
return title_upper;
}
void Movie::set_year(int year_param)
{
year = year_param;
}
int Movie::get_year() const
{
return year;
}
/*
Main file: Movie_List.cpp
Utilizes Class Movie
*/
#include <iostream>
#include <iomanip>
#include <string>
#include <
#include "Movie.h"
// You need to replace "using namespace std;" with "using" declarations (see Movie.cpp as an example)
using namespace std; // Replace this with "using std::cout", etc.
int main()
{
cout << "The Movie List program\n\n"
<< "Enter a movie...\n\n";
// get vector of Movie objects
vector<Movie> movies;
char another = 'y';
while (tolower(another) == 'y')
{
Movie movie; // movie is initialized with the Default Constructor values
string title;
cout << "Title: ";
getline(cin, title);
movie.set_title(title);
int year;
cout << "Year: ";
cin >> year;
movie.set_year(year);
movies.push_back(movie);
cout << "\nEnter another movie? (y/n): ";
cin >> another;
cin.ignore();
cout << endl;
}
// display the movies
const int w = 10;
cout << left
<< setw(w * 3) << "TITLE"
<< setw(w) << "YEAR" << endl;
for (Movie movie : movies)
{
cout << setw(w * 3) << movie.get_title()
<< setw(w) << movie.get_year() << endl;
}
cout << endl;
// Output with titles in ALL CAPS
for (Movie movie : movies)
{
cout << setw(w * 3) << movie.get_title_upper()
<< setw(w) << movie.get_year() << endl;
}
cout << endl;
return 0;
}
Modify the code above to create a "Favorite Video Game List" program that is contained in three files:
- Game.h
- Game.cpp
- Game_List.cpp
You will need do to these things as you modify the code and put it into the three files:
- Create a class called "Game" (instead of "Movie") that contains 3 private variables ("title", "year", and "rating")
- The "year" is when the game first came out.
- The "rating" should be from 1 to 5 (5 being the best).
- Add the appropriate member functions of the class (setters and getters).
- Continue to use the get_title_upper() function as part of the class, Game, and in the output within the main file, Game_List.cpp.
-
- Add the Default Constructor and Destructor for the class with the member functions of the class.
- Don't forget to add the "include guard" to the .h file
- Use the scope resolution operator correctly for each file.
- Don't use the "using namespace std;" for the main file, Game_List.cpp.
- Instead, use the "using" declaration
- using std::cout;
- using std::endl;
- using std::string;
- etc.
- Instead, use the "using" declaration

Step by step
Solved in 4 steps with 6 images

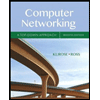
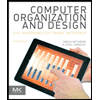
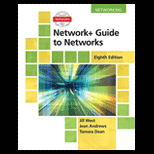
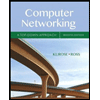
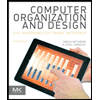
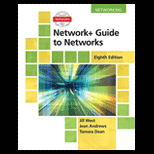
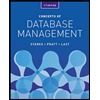
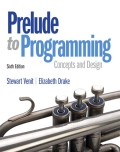
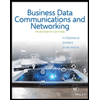