fix the getIndex function
Not sure how to fix the getIndex function please help
---------------------------
#include <iostream>
#include <cassert>
using namespace std;
class SomeObj{
public:
SomeObj(int d ): id(d){}
int getId() const;
void output();
private:
int id;
};
int SomeObj::getId() const{
return id;
}
void SomeObj::output(){
cout<<id<<endl;
}
template<typename T>
class MyArray {
public:
MyArray();
MyArray(int c);
T& operator[](int index);
void push_back(T e);
int getSize() const;
int getCapacity() const;
int getIndex(T a);
void erase();
private:
void grow();
T *data;
int capacity;
int size;
};
template <typename T>
MyArray<T>::MyArray(): MyArray(1) {
}
template <typename T>
MyArray<T>::MyArray(int c) {
assert(c>0);
size = 0;
capacity = c;
data = new T[capacity];
}
template <typename T>
T& MyArray<T>::operator[](int index) {
assert(index >=0 && index < size);
return data[index];
}
template <typename T>
int MyArray<T>::getSize() const {
return size;
}
template <typename T>
int MyArray<T>::getCapacity() const {
return capacity;
}
template <typename T>
void MyArray<T>::grow(){
int* new_a = new int[(capacity*2)-1];
for (int i = 0; i < size; i++){
new_a[i] = *data[i];
}
capacity = (capacity*2)-1;
data = &new_a;
}
template <typename T>
int MyArray<T>::getIndex(T a){
for(int i = 0; i < size; i++){
if(data[i] == a){
return i;
}
else return -1;
}
return 0;
}
template <typename T>
void MyArray<T>::push_back(T e) {
if(size>=capacity){
grow();
}
else
assert(size < capacity);
data[size++] = e;
}
template <typename T>
void MyArray<T>::erase(){
for (int i = size; i < capacity; i++){
data[size] = data[size + 1];
data[capacity - 1] = data[size];
}
}
template <typename T>
void printHeap(MyArray<T> &m) {
for(int i=0; i<m.getSize();i++) {
cout << *(m[i]) << " ";
}
cout << endl;
}
int main() {
cout << endl;
MyArray<int*> Mnums(10);
for(int i=0; i<Mnums.getCapacity(); i++) {
int *nums = new int(i+1);
Mnums.push_back(nums);
}
printHeap(Mnums);
cout << endl;
return 0;
}



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

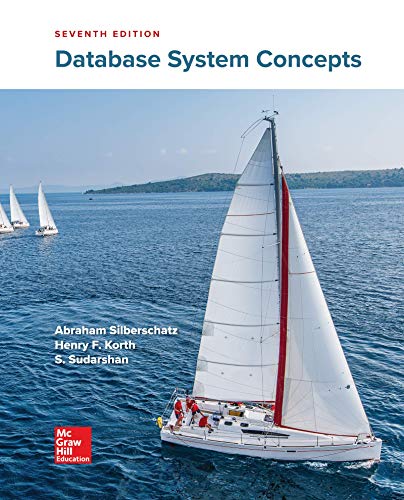
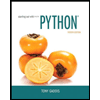
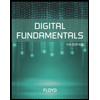
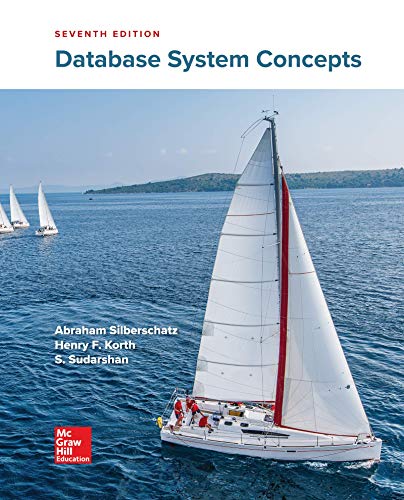
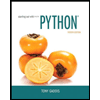
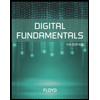
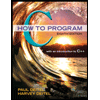
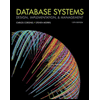
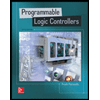