#include #include using namespace std; void PrintSize(vector numsList) { cout << numsList.size() <<< items" << endl; } int main() { } int currval; vector intList (2); PrintSize(intList); cin >> currval; while (currval >= 0) { intList.push_back(currval); cin >> currval; } PrintSize(intList); intList.clear(); PrintSize(intList); return 0; Input 12345-1 Output
#include #include using namespace std; void PrintSize(vector numsList) { cout << numsList.size() <<< items" << endl; } int main() { } int currval; vector intList (2); PrintSize(intList); cin >> currval; while (currval >= 0) { intList.push_back(currval); cin >> currval; } PrintSize(intList); intList.clear(); PrintSize(intList); return 0; Input 12345-1 Output
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Check
1
ALLLEURE
#include <iostream>
#include <vector>
using namespace std;
void PrintSize(vector<int> numsList) {
cout << numsList.size() << "items" << endl;
int main() {
Next
int currVal;
vector<int> intList (2);
PrintSize(intList);
cin >> currval;
while (currVal >= 0) {
}
Type the program's output
intList.push_back(currval);
cin >> currval;
PrintSize(intList);
intList.clear();
PrintSize(intList);
return 0;
CS Scanned with Calin canner
Janviantars
Input
12345-1
Output
Feedback?
口口。
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
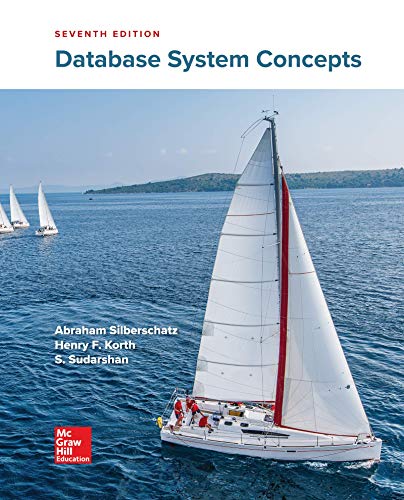
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
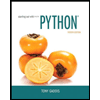
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
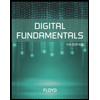
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
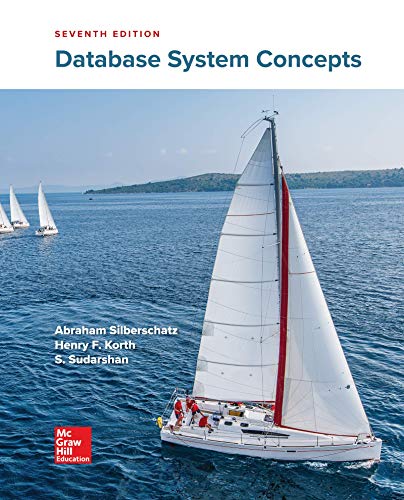
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
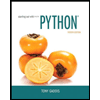
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
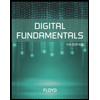
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
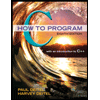
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
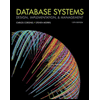
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
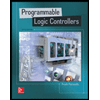
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education