ude using namespace std; struct node { public: int data; node* left; node* right; };
**Print the order of insertion to the screen for the code below**
#include<iostream>
using namespace std;
struct node
{
public:
int data;
node* left;
node* right;
};
class BST
{
public:
node* insert(node* , int);
void Preorder(node*);
void Inorder(node*);
void Postorder(node*);
};
node* get_new_node(int n)
{
node* temp = new node;
temp->data = n;
temp->left = NULL;
temp->right = NULL;
return temp;
}
node* BST::insert(node* root , int data)
{
if(root == NULL)
{
root = get_new_node(data);
}
else if (data <= root->data)
{
root->left = insert(root->left , data);
}
else
{
root->right = insert(root->right , data);
}
return root;
}
void BST::Preorder(node* root)
{
if(root == NULL){
return ;
}
else
{
cout << root -> data << " ";
Preorder(root -> left);
Preorder(root -> right);
}
}
void BST::Inorder(node* root)
{
if(root == NULL){
return ;
}
else
{
Inorder(root -> left);
cout<<root -> data << " ";
Inorder(root -> right);
}
}
void BST::Postorder(node* root)
{
if(root == NULL){
return;
}
else
{
Postorder(root -> left);
Postorder(root -> right);
cout << root -> data << " ";
}
}
int main()
{
int ch = 1;
BST bt;
node* root = NULL;
while(ch){
cout << "Please select an operation (enter 1 , 2 , 3 or 4; to quit enter 0)" << endl;
cout << "1. Insert element" << endl;
cout << "2. Pre-Order traversal" << endl;
cout << "3. In-Order Traversal" << endl;
cout << "4. Post-order Traversal" << endl;
cin >> ch;
int ele = 0;
switch(ch){
case 0 : exit(0);
break;
case 1 : cout << "Enter the element to insert: ";
cin >> ele;
root = bt.insert(root , ele);
break;
case 2 : if(root == NULL){
cout << "Tree is empty" << endl;
break;
}
bt.Preorder(root);
cout << "\n\n";
break;
case 3 : if(root == NULL){
cout << "Tree is empty" << endl;
break;
}
bt.Inorder(root);
cout << "\n\n";
break;
case 4 : if(root == NULL){
cout << "Tree is empty" << endl;
break;
}
bt.Postorder(root);
cout << "\n\n";
break;
default: cout << "Wrong choice" << endl;
break;
}
}
return 0;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

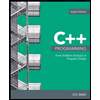
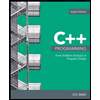