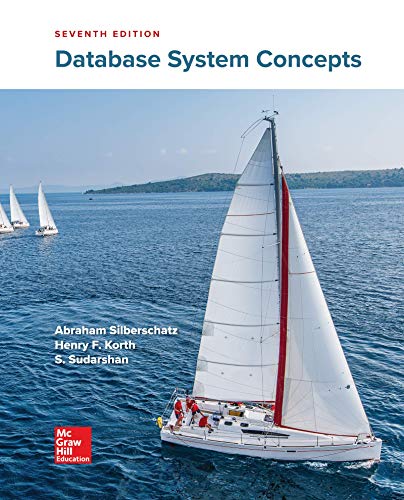
#include<iostream>
#include<string>
class Bicycle
{
friend std::ostream& operator<<(std::ostream& out_ref, const Bicycle& bike_ref);
public:
Bicycle(const std::string& name_ref, unsigned int gear, bool motorized, double range):
name_(name_ref), gear_(gear), motorized_(motorized), range_(range){current_gear_=1;};
Bicycle operator++() ;
Bicycle operator--(int) ;
double operator<(const Bicycle& rhs_ref) const;
double operator>(const Bicycle& rhs_ref) const;
double operator!() const;
std::string name() const { return name_;};
unsigned int current_gear() const { return current_gear_;};
unsigned int gear_max() const { return gear_max_;};
double range() const { return range_;}
bool motorized() const { return motorized_;};
private:
std::string name_;
unsigned int gear_max_;
unsigned int current_gear_;
double range_;
bool motorized_;
};
For the operator-- a member function should be used. The function shall decrement the current gear (current_gear_) if the current gear is higher then 1. Otherwise nothing shall happen.
For example the result of
std::cout << (bicycle1--).current_gear() << std::endl // consol output: 2
std::cout << bicycle1.current_gear() << std::endl;
to generate a solution
a solution
- C++ Find the errors in the code for Date Class and Source.cpp program and fix them. source.cpp include <iostream>#include "Date.h" // include definition of class Date from Date.h using namespace std; // function main begins program executionint main(){Date date(); // create a Date object for May 6, 1981 // display the values of the three Date data members cout << "Month: " << date.getMonth() << endl;cout « "Day: " << date.getDay() << endl;cout << "Year: " << date.getYear(2017) << endl; cout << "\nOriginal date:" << endl;date = displayDate(); // output the Date// modify the Date setMonth(13);setDay(1);setYear(2005); cout "\nNew date:" << endl;date.displayDate(); // output the modified date } // end main Date.h // class Data definition#include <iostream>using namespace std; //Data constructor that initializes the three data members;class Date{publicdate(m, d, y){setMonth();setDay();setYear();};// set monthvoid…arrow_forwardStatic analyzer write code c++ create your static analyzer tool thatreads your code as a text. Then, it analyzes it based on the below checklist. The checklist: - Do the attributes (e.g., data type and size) of each parameter match theattributes of each corresponding argument?arrow_forward} BMI(struct Person* person) { person->BMI = person->weight / (person->height person->height); return person->BMI; } //Q10: Why is the return type char*? char* determineWeightCategory(struct Person* person) {//assumes BMI is known const double UNDERWEIGHT = 18.5; const double NORMALWEIGHT = 24.9; const double OVERWEIGHT = 29.9; } else { //Q11: If the value in person->BMI is equal to 18.5 and person is athletic, what will be //stored in p- //Q12: The first if statement validates against certain conditions. //What are the conditions it is validating against? //HINT: Think about what causes BMI to be less than 0. if (person->BMI weightCategory, "ERROR"); } else if (person->BMI weightCategory, "Underweight"); } else if (person->BMI weightCategory, "Normalweight"); } else if (person->BMI weightCategory, "Overweight"); strcpy(person->weightCategory, "Obese"); return person->weightCategory; //Q13: Why do we return person->weightCategory? } //Q14: Why is provider passed by value?arrow_forward
- #ifndef INT_SET_H#define INT_SET_H #include <iostream> class IntSet{public: static const int DEFAULT_CAPACITY = 1; IntSet(int initial_capacity = DEFAULT_CAPACITY); IntSet(const IntSet& src); ~IntSet(); IntSet& operator=(const IntSet& rhs); int size() const; bool isEmpty() const; bool contains(int anInt) const; bool isSubsetOf(const IntSet& otherIntSet) const; void DumpData(std::ostream& out) const; IntSet unionWith(const IntSet& otherIntSet) const; IntSet intersect(const IntSet& otherIntSet) const; IntSet subtract(const IntSet& otherIntSet) const; void reset(); bool add(int anInt); bool remove(int anInt); private: int* data; int capacity; int used; void resize(int new_capacity);}; bool operator==(const IntSet& is1, const IntSet& is2); #endifarrow_forwardC++ Student Data Write a program that uses two structures Name and Student to store the following information for multiple students: Create a nameType structure that consists of First Name, Middle Initial, and Last Name Create a studentType structure that contains student information (Include the nameType structure within the Student information structure): Name ID email GPA Program (an enum type named programType containing programs such as CSCI, DBMS, INFM, SDEV) Suppose that a class has at most 20 students. Use an array of 20 components of type studentType. You will read in the names, id, and email from classroster.txt. The first line of the file will tell you how many students are in the class. To get the the GPA and program for each student you will need to read from studentlist.txt. The second file will have the id, program, and GPA for all of the students in the department. That means that not every student from studentlist.txt will be in classroster.txt. You will…arrow_forward// CLASS PROVIDED: sequence (part of the namespace CS3358_FA2022)//// TYPEDEFS and MEMBER CONSTANTS for the sequence class:// typedef ____ value_type// sequence::value_type is the data type of the items in the sequence.// It may be any of the C++ built-in types (int, char, etc.), or a// class with a default constructor, an assignment operator, and a// copy constructor.//// typedef ____ size_type// sequence::size_type is the data type of any variable that keeps// track of how many items are in a sequence.//// static const size_type DEFAULT_CAPACITY = _____// sequence::DEFAULT_CAPACITY is the default initial capacity of a// sequence that is created by the default constructor.//// CONSTRUCTOR for the sequence class:// sequence(size_type initial_capacity = DEFAULT_CAPACITY)// Pre: initial_capacity > 0// Post: The sequence has been initialized as an empty sequence.// The insert/attach functions will work efficiently (without// allocating…arrow_forward
- C++ OOParrow_forwardC++ coding project just need some help getting the code thank you!arrow_forwardint num=10, k=2; int *ptr1, *ptr2, *ptr3; San c++ language ptrl = ptrl ptrl = ptrl ptr3 = ptr2 ptr3 = 2; k; %3D ptrl; = (int *) (ptr2 ptrl) ptr3 ptr3 = ptr2 ptrl k; (int *) (ptr2 Valid ptr1) + k; ptrl ptrl + 2; Or ptrl = ptrl + k; ptr3 = ptrl + ptr2; ptr3 = Not valid (int *) (ptrl + ptr2) = ptrl + ptr2 + k; (int *) (ptrl + ptr2) + k; ptr3 ptrl = ptrl * 2; ptrl = ptrl * k; ptr3 = ptr2 * ptrl; ptrl = ptrl / 2 ptrl = ptrl / k; ptr2 / ptr%; ptr3 =arrow_forward
- computer programming (C++ language)arrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardParaphrase this code plz #include<iostream>using namespace std;struct studmodule{char modulename[30];int totalmarks;}; struct Student{char name[30];int age;int studid;struct studmodule module[4];float avgmarks;}stud[5];float avg;float classavg;int highmark=0;int lowmark=0;float passrate=0;void inputdata(){int i=0,tot;cout<<"-------------------------------------------"<<endl;cout<<"Welcome to Technology College Muscut"<<endl;cout<<"-------------------------------------------"<<endl;cout<<"Students Personnel data Entry"<<endl;cout<<"-------------------------------------------"<<endl;for (i=0;i<5;i++){tot=0;cout<<"Enter Student "<<i+1 << "Name :";cin>>stud[i].name;cout<<"Enter Student "<<i+1 << "Id Number :";cin>>stud[i].studid;cout<<"Enter Student "<<i+1 << "Age…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
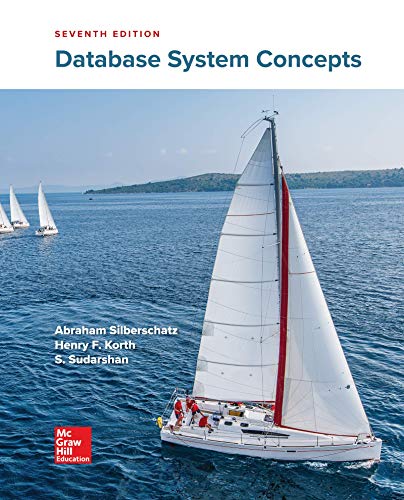
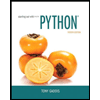
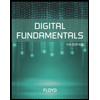
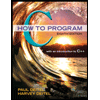
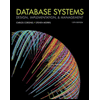
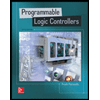