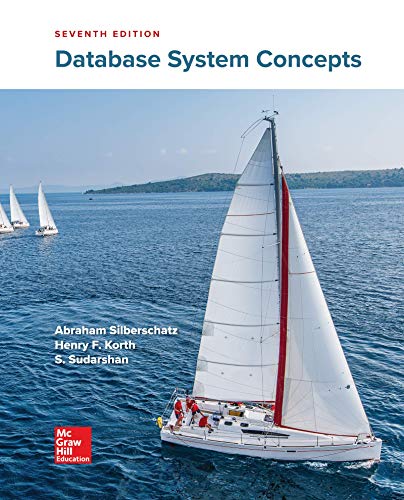
Concept explainers
I need help with fixing this java program as described in the image below:
LablProgram.java:
import java.util.Scanner;
public class LabProgram {
/* TODO: Write recursive printLinkedList() method here. */
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int size;
int value;
size = scnr.nextInt();
value = scnr.nextInt();
IntNode headNode = new IntNode(value); // Make head node as the first node
IntNode lastNode = headNode; // Node to add after
IntNode newNode = null; // Node to create
// Insert the second and the rest of the nodes
for (int n = 0; n < size - 1; ++n) {
value = scnr.nextInt();
newNode = new IntNode(value);
lastNode.insertAfter(newNode);
lastNode = newNode;
}
// Call printLinkedList() with the head node
printLinkedList(headNode);
}
}
intNode.java:
public class IntNode {
int dataVal;
private IntNode nextNodeRef; // Reference to the next node
// Constructor
public IntNode(int value) {
this.dataVal = value;
this.nextNodeRef = null;
}
// Insert a new node after the current node
public void insertAfter(IntNode nodeLoc) {
IntNode tmpNext;
tmpNext = this.nextNodeRef;
this.nextNodeRef = nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
// Get location pointed by nextNodeRef
public IntNode getNext() {
return this.nextNodeRef;
}
// Print the node's data
public void printData() {
System.out.printf("%d, ", this.dataVal);
}
}
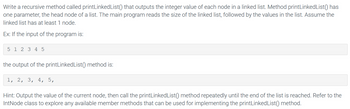

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Using Java.arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardCorrect my codes in java // Arraysimport java.util.Scanner;public class Assignment1 {public static void main (String args[]){Scanner sc=new Scanner (System.in);System.out.println("Enter mark of student");int n=sc.nextInt();int a[]=new int [n];int i;for(i=0;i<n;i++){System.out.println("Total marks of student in smster");a[i]=sc.nextInt();}int sum=0;for(i=0;i<n;i++){sum=sum+a[i];}System.out.println("Total marks is :");for (i=0;i<n;i++);{System.out.println(a[i]);}System.out.println();}}arrow_forward
- How do I code: public static void rotateElements(int[] arr, int rotationCount) public static void reverseArray(int[] arr) In Java?arrow_forwardPRACTICE CODE import java.util.TimerTask;import org.firmata4j.ssd1306.MonochromeCanvas;import org.firmata4j.ssd1306.SSD1306;public class CountTask extends TimerTask {private int countValue = 10;private final SSD1306 theOledObject;public CountTask(SSD1306 aDisplayObject) {theOledObject = aDisplayObject;}@Overridepublic void run() {for (int j = 0; j <= 3; j++) {theOledObject.getCanvas().clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "Hello");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}theOledObject.clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "My name is ");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}while (true) {for (int i = 10; i >= 0; i--)…arrow_forwardWrite in Java What is this program's exact outputarrow_forward
- need help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forwardI need help with this Java Problem as described in the image below: import java.util.Scanner; public class LabProgram { public static int fibonacci(int n) { /* Type your code here. */ } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int startNum; startNum = scnr.nextInt(); System.out.println("fibonacci(" + startNum + ") is " + fibonacci(startNum)); }}arrow_forwardI need help with this Java problem to output as it's explained in this image below: import java.util.Scanner;import java.util.ArrayList;import java.util.Collections;public class LabProgram { static public int recursions = 0; static public int comparisons = 0; private static ArrayList<Integer> readNums(Scanner scnr) { int size = scnr.nextInt(); ArrayList<Integer> nums = new ArrayList<Integer>(); for (int i = 0; i < size; ++i) { nums.add(scnr.nextInt()); } return nums; } static public int binarySearch(int target, ArrayList<Integer> integers, int lower, int upper) { recursions+=1; int index = (lower+upper)/2; comparisons+=1; if(target == integers.get(index)){ return index; } if(lower == upper){ comparisons+=1; if(target == integers.get(lower)){ return lower; } else{…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
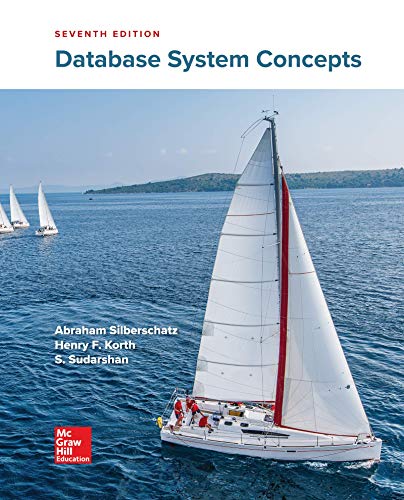
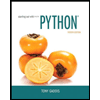
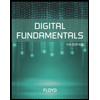
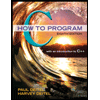
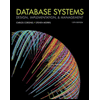
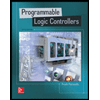