import java.util.*; import java.io.FileWriter; import java.io.IOException; public class Main { public static void main(String[] args) throws IOException { //For taking inputs Scanner in = new Scanner(System.in); ArrayList daysEntered = new ArrayList(); // For storing dangerous reading value int dangerous; // Ask and input the reading that is considered dangerous System.out.print("Enter dangerous reading: "); dangerous = in.nextInt(); // To write in file FileWriter myWriter = new FileWriter("report.txt"); // To store day of the month int day = 1; // To store the three readings in a day int first_reading, second_reading, third_reading; // Loops until user input 0 as day while(day != 31) { // Ask and input day of the month System.out.print("Enter day of the month ( 1 to 31 ): "); day = in.nextInt(); // If readings for the entered day has already entered if(daysEntered.contains(day)) { System.out.println("Readings for this day has already been entered. "); } // Else else { daysEntered.add(day); // If day entered is valid if(day >= 1 && day <= 31) { // Ask and input three daily readings System.out.println("Enter the three readings: "); first_reading = in.nextInt(); second_reading = in.nextInt(); third_reading = in.nextInt(); // Find the peak flow reading of the day int maximum_reading = Math.max(Math.max(first_reading, second_reading), third_reading); // If peak flow reading is below dangerous reading if(maximum_reading < dangerous) { // Tell user he/she needs to go to hospital System.out.println("--- You need to go to hospital immediately ---"); } // Write the day and peak flow reading in a file separated with comma (CSV) myWriter.write(day+","+maximum_reading+"\n"); } } } // Close myWriter myWriter.close(); } } Explain briefly in your own words (separately from the code, in the box provided) how one important method of your program answering part i) works. It should be a method specific to this program and not, for example, a generic method provided for you such as a generic input method.
import java.util.*;
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) throws IOException {
//For taking inputs
Scanner in = new Scanner(System.in);
ArrayList<Integer> daysEntered = new ArrayList<Integer>();
// For storing dangerous reading value
int dangerous;
// Ask and input the reading that is considered dangerous
System.out.print("Enter dangerous reading: ");
dangerous = in.nextInt();
// To write in file
FileWriter myWriter = new FileWriter("report.txt");
// To store day of the month
int day = 1;
// To store the three readings in a day
int first_reading, second_reading, third_reading;
// Loops until user input 0 as day
while(day != 31) {
// Ask and input day of the month
System.out.print("Enter day of the month ( 1 to 31 ): ");
day = in.nextInt();
// If readings for the entered day has already entered
if(daysEntered.contains(day)) {
System.out.println("Readings for this day has already been entered. ");
}
// Else
else {
daysEntered.add(day);
// If day entered is valid
if(day >= 1 && day <= 31) {
// Ask and input three daily readings
System.out.println("Enter the three readings: ");
first_reading = in.nextInt();
second_reading = in.nextInt();
third_reading = in.nextInt();
// Find the peak flow reading of the day
int maximum_reading = Math.max(Math.max(first_reading, second_reading), third_reading);
// If peak flow reading is below dangerous reading
if(maximum_reading < dangerous) {
// Tell user he/she needs to go to hospital
System.out.println("--- You need to go to hospital immediately ---");
}
// Write the day and peak flow reading in a file separated with comma (CSV)
myWriter.write(day+","+maximum_reading+"\n");
}
}
}
// Close myWriter
myWriter.close();
}
}
Explain briefly in your own words (separately from the code, in the box provided) how one important method of your program answering part i) works. It should be a method specific to this program and not, for example, a generic method provided for you such as a generic input method.

Step by step
Solved in 3 steps with 2 images

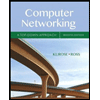
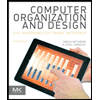
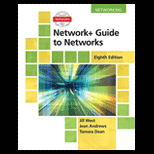
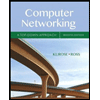
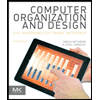
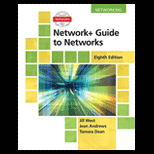
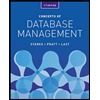
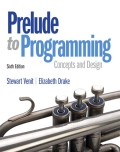
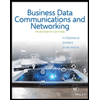