import java.util.*; public class Main0 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int totalEmployee = 10; String [] fname = new String[totalEmployee]; String [] mname = new String[totalEmployee]; String [] lname = new String[totalEmployee]; int [] idNum = new int[totalEmployee]; // Hours Worked Must be Between 0 and 60 Hours int [] workHour = new int[totalEmployee]; // Rate per Hour Must be Between $15.00 and $35.00 int [] ratePerHour = new int[totalEmployee]; // add Data in the array for (int i=0; i 0){ if(ratePerHour[i] >= 15 && ratePerHour[i] <= 35){ if(workHour[i] < 40){ gross[i] = workHour[i] * ratePerHour[i]; } else{ gross[i] = (40 * ratePerHour[i] + (workHour[i] - 40) * ratePerHour[i] * 1.5); } } else{ System.out.println("Please Enter Your Number Between 15 to 35"); } } else{ System.out.println("Please Enter Greater Number"); } } // Stored State Tax, Fed Tax and Net in the array for 10 Employee double [] state_tax = new double[totalEmployee]; double [] fed_tax = new double[totalEmployee]; double [] net = new double[totalEmployee]; for(int i=0; i
import java.util.*;
public class Main0 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int totalEmployee = 10;
String [] fname = new String[totalEmployee];
String [] mname = new String[totalEmployee];
String [] lname = new String[totalEmployee];
int [] idNum = new int[totalEmployee];
// Hours Worked Must be Between 0 and 60 Hours
int [] workHour = new int[totalEmployee];
// Rate per Hour Must be Between $15.00 and $35.00
int [] ratePerHour = new int[totalEmployee];
// add Data in the array
for (int i=0; i<totalEmployee; i++){
// First Name Input
System.out.println("Enter First Name");
fname[i] = sc.next();
// Middle Name Input
System.out.println("Enter Middle Name");
mname[i] = sc.next();
// Last Name Input
System.out.println("Enter Last Name");
lname[i] = sc.next();
// Id Number Input
System.out.println("Enter Id number");
idNum[i] = sc.nextInt();
// Hours Worked Input
System.out.println("Enter Hours worked");
workHour[i] = sc.nextInt();
// Rate Per Hour Input
System.out.println("Enter Rate Per Hour");
ratePerHour[i] = sc.nextInt();
}
// State Tax Percentage
int state_tax_p = 7;
// Fed Tax Percentage
int fed_tax_p = 15;
// Gross Array Created
double [] gross = new double[totalEmployee];
for (int i=0; i<totalEmployee; i++){
if(workHour[i] > 0){
if(ratePerHour[i] >= 15 && ratePerHour[i] <= 35){
if(workHour[i] < 40){
gross[i] = workHour[i] * ratePerHour[i];
}
else{
gross[i] = (40 * ratePerHour[i] + (workHour[i] - 40) * ratePerHour[i] * 1.5);
}
}
else{
System.out.println("Please Enter Your Number Between 15 to 35");
}
}
else{
System.out.println("Please Enter Greater Number");
}
}
// Stored State Tax, Fed Tax and Net in the array for 10 Employee
double [] state_tax = new double[totalEmployee];
double [] fed_tax = new double[totalEmployee];
double [] net = new double[totalEmployee];
for(int i=0; i<totalEmployee; i++){
state_tax[i] = gross[i] * state_tax_p;
fed_tax[i] = gross[i] * fed_tax_p;
net[i] = gross[i] - (state_tax[i] + fed_tax[i]);
}
// Print All The Data With Table Format
System.out.printf("%-15s %-15s %-15s %-15s %-15s %-15s %-15s %-15s %-15s\n", "Last Name", "First Name", "MI Id", "Rate Per Hour", "Hours Worked", "State Tax", "Fed tax", "Gross", "Net");
for(int i=0; i<totalEmployee; i++) {
System.out.printf("%-15s %-15s %-15d %-15d %-15d %-15f %-15f %-15f %-15f\n", lname[i], fname[i], idNum[i], ratePerHour[i], workHour[i], state_tax[i], fed_tax[i], gross[i], net[i]);
}
}
}
Pseudo Code

Step by step
Solved in 2 steps

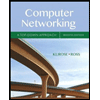
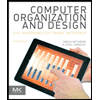
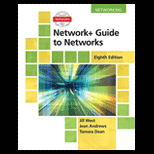
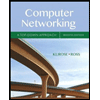
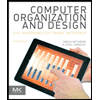
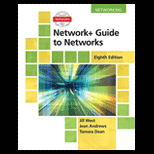
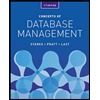
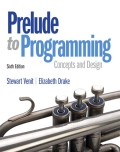
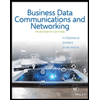