java DDI Osrc main java > ControlPanel.java 129 v 130 131 132 133 134 135 ✓ 136 137 138 v 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161, 162 163 164 165 166 167 168 169 170 } } private void execute Instruction ( Instruction instruction) { String opcode= instruction.getOpcode(); int[] args = instruction.getArgs(); switch (opcode) { case "load": } for (Instruction instruction instructions) { if (!miniMac.isRunning()) break; executeInstruction (instruction); } }$$ } miniMac.load(args[0], args[1]); break; case "halt": miniMac.halt (); $ break; case "add": miniMac.add(args[0], args[1], args[2]); break; case "mul": miniMac.mul(args[0], args[1], args[2]); break; default: // Placeholder for other operations break; private void updateMemoryView() { DefaultListModel memoryModel = new DefaultListModel(); Integer [] memory - miniMac.getMemory(); for (int i = 0; i< memory.length; i++) { memoryModel.addElement("Memory[" + i + "]: " + memory[i]); } updateMemoryView(); memoryView.setModel (memoryModel); public static void main(String[] args) { SwingUtilities.invokeLater (Control Panel::new); Format Hell
import javax.swing.*;
import java.awt.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Arrays;
class MiniMac {
private Integer[] memory;
private boolean running;
public MiniMac() {
memory = new Integer[32];
Arrays.fill(memory, 0);
running = true;
}
public void load(int location, int value) {
memory[location] = value;
}
public void halt() {
running = false;
}
public void add(int src1, int src2, int dest) {
memory[dest] = memory[src1] + memory[src2];
}
public void mul(int src1, int src2, int dest) {
memory[dest] = memory[src1] * memory[src2];
}
public Integer[] getMemory() {
return memory;
}
public boolean isRunning() {
return running;
}
}
class Instruction {
private String opcode;
private int[] args;
public Instruction(String opcode, int[] args) {
this.opcode = opcode;
this.args = args;
}
public String getOpcode() {
return opcode;
}
public int[] getArgs() {
return args;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(opcode);
for (int arg : args) {
sb.append(" ").append(arg);
}
return sb.toString();
}
}
class MiniMacParser {
public static List<Instruction> parse(String
List<Instruction> instructions = new ArrayList<>();
String[] lines = program.split("\n");
for (String line : lines) {
instructions.add(parseInstruction(line.trim()));
}
return instructions;
}
private static Instruction parseInstruction(String line) {
String[] tokens = line.split("\\s+");
String opcode = tokens[0];
int[] args = new int[tokens.length - 1];
for (int i = 1; i < tokens.length; i++) {
args[i - 1] = Integer.parseInt(tokens[i]);
}
return new Instruction(opcode, args);
}
}
public class ControlPanel extends JFrame {
private MiniMac miniMac;
private JList<String> memoryView;
private JList<String> programView;
public ControlPanel() {
miniMac = new MiniMac();
setupUI();
}
private void setupUI() {
memoryView = new JList<>();
programView = new JList<>();
JButton loadProgramButton = new JButton("Load Program");
loadProgramButton.addActionListener(e -> loadProgram());
JPanel panel = new JPanel(new GridLayout(1, 2));
panel.add(new JScrollPane(memoryView));
panel.add(new JScrollPane(programView));
add(panel, BorderLayout.CENTER);
add(loadProgramButton, BorderLayout.SOUTH);
setTitle("MiniMac Control Panel");
setSize(600, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void loadProgram() {
String program = "load 0 10\nload 1 20\nadd 0 1 2\nmul 0 1 3";
List<Instruction> instructions = MiniMacParser.parse(program);
DefaultListModel<String> programModel = new DefaultListModel<>();
for (Instruction instruction : instructions) {
programModel.addElement(instruction.toString());
}
programView.setModel(programModel);
executeProgram(instructions);
}
private void executeProgram(List<Instruction> instructions) {
for (Instruction instruction : instructions) {
if (!miniMac.isRunning()) break;
executeInstruction(instruction);
}
}
I paste the half code and the rest half i sent you in screenshot. Please check why the output i am getting "Hello World" PLease check where i am doing mistake and help me to fix
![Search
- Files Ⓒ
src
main
java
Control Panel.java
Packager files
target
pom.xml
→ Tools
Recent
All
CAI
Deployments
Authentication
Chat
Main.java
Control Panel.java X +
□src › □ main › java › Control Panel.java
129 v
130
131
132
133
134
135
136
V
137
138 v
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158 v
159
160
161
162
163
164
165
166
167 v
168
169
170 }
171
}
}
private void executeInstruction ( Instruction instruction) {
String opcode instruction.getOpcode();
instruction.getArgs();
int[] args
switch (opcode) {
case "load":
for (Instruction instruction: instructions) {
if (!miniMac.isRunning()) break;
executeInstruction (instruction);
}
}
}
}$$
=
}
miniMac.load(args[0], args[1]);
=
break;
case "halt":
miniMac.halt();$
break;
case "add":
miniMac.add(args[0], args[1], args[2]);
break;
case "mul":
miniMac.mul(args[0], args[1], args[2]);
break;
default:
private void updateMemoryView() {
DefaultListModel<String> memoryModel = new DefaultListModel<>();
Integer [] memory miniMac.getMemory();
for (int i = 0; i < memory.length; i++) {
memoryModel.addElement("Memory[" + i + "]:
// Placeholder for other operations
break;
updateMemoryView();
memoryView.setModel (memoryModel );
public static void main(String[] args) {
SwingUtilities.invokeLater(Control Panel::new);
+ memory[i]);
Format
Console
Run
Hello world!
Shell
+](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F325f30ca-56bc-46bd-9932-3ddac22cee03%2F0cb732e7-c67e-4a13-a949-c8171e8702e0%2Fsu0odga_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

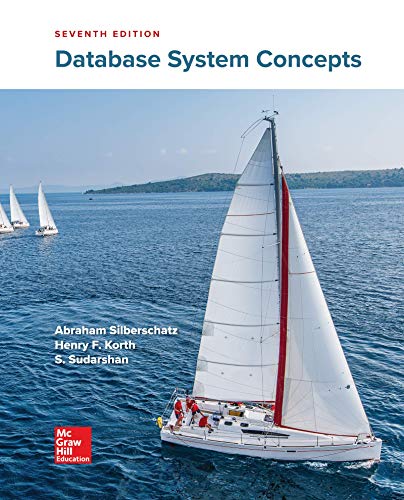
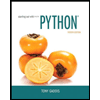
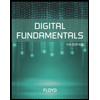
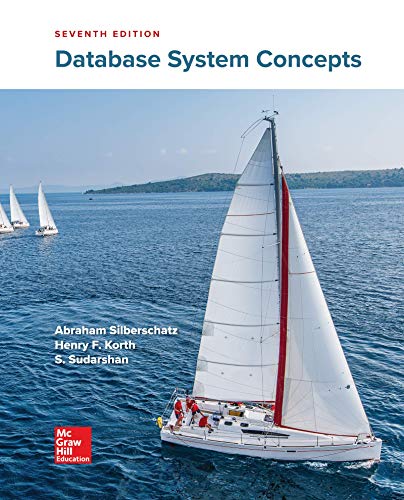
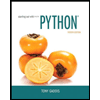
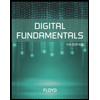
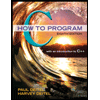
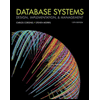
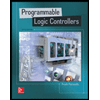