The language used here is in Java. Please answer the question in the screenshot. Please use the code below as a base. Please only answer question 2. import java.util.*; class SortingPack { // just in case you need tis method for testing public static void main(String[] args) { // something } // implementation of insertion sort // parameters: int array unsortedArr // return: sorted int array public static int[] insertionSort(int[] unsortedArr) { // to be removed return unsortedArr; } // implementation of quick sort // parameters: int array unsortedArr // return: sorted int array public static int[] quickSort(int[] unsortedArr) { // to be removed return unsortedArr; } // implementation of merge sort // parameters: int array unsortedArr // return: sorted int array public static int[] mergeSort(int[] unsortedArr) { // to be removed return unsortedArr; } // you are welcome to add any supporting methods } import java.util.*; public class SortingTest{ public static void main(String[] args) { SortingTest obj = new SortingTest(); // DO NOT DELETE THE THREE TEST cases obj.test("insertSort", 10); obj.test("quicktSort", 20); obj.test("mergeSort", 30); // you are welcome to add more testing cases } // test any one of the three given sorting
The language used here is in Java. Please answer the question in the screenshot. Please use the code below as a base. Please only answer question 2.
import java.util.*;
class SortingPack {
// just in case you need tis method for testing
public static void main(String[] args) {
// something
}
// implementation of insertion sort
// parameters: int array unsortedArr
// return: sorted int array
public static int[] insertionSort(int[] unsortedArr) {
// to be removed return unsortedArr;
}
// implementation of quick sort
// parameters: int array unsortedArr
// return: sorted int array
public static int[] quickSort(int[] unsortedArr) {
// to be removed return unsortedArr;
}
// implementation of merge sort
// parameters: int array unsortedArr
// return: sorted int array
public static int[] mergeSort(int[] unsortedArr) {
// to be removed return unsortedArr;
}
// you are welcome to add any supporting methods
}
import java.util.*;
public class SortingTest{
public static void main(String[] args) {
SortingTest obj = new SortingTest();
// DO NOT DELETE THE THREE TEST
cases obj.test("insertSort", 10);
obj.test("quicktSort", 20);
obj.test("mergeSort", 30);
// you are welcome to add more testing cases
}
// test any one of the three given sorting algorithms in SortingPack
// parameters:
// sortAlgorithm - string, name of the sorting
// size - int, the length of the randomly generated array
// no return data
public void test(String sortAlgorithm, int size) {
}
![1. Implement three sorting algorithms in the file named "SortingPack.java" as three
static methods:
Insertion sorting: Shall be named as “insertionSort"; accepts an integer array as
the only parameter; return the sorted array
public static int[] insertionSort(int[] unsortedArr)
Quick sorting: Shall be named as “quickSort"; accepts an integer array as the
only parameter; return the sorted array
public static int[] guickSort(int[] unsortedArr)
Merge sorting: Shall be named as “mergeSort"; accepts an integer array as the
only parameter; return the sorted array
public static int[] mergeSort(int[] unsortedArr)
The skeleton of the code has been provided to you. You are NOT allowed to change
the parameter numbers or return data types. However, you can add as many
helper methods as you want, and do anything about the helper methods.
2. Test your sorting algorithms
Implement a method named "test" in the file named "SortingTest.java" that tests a
specific sorting algorithm. This method will take three parameters:
name of the sorting algorithm (string): insertionSort, quickSort, or mergeSort
ww
size of a randomly generated array (int): e.g., 1000
This method will:
1. Generate a random array of the length specified by the 2nd parameter
2. Time the milliseconds required to perform sorting on the generated array
3. Print out a set of user-friendly explanation of the testing and the required time:
Testing for insertionSort starts.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1725b614-c61a-470a-b05f-008b5304bcc7%2F39296fa7-6b4b-4f48-a9b5-79f4a79145e1%2Fte3i4hl_processed.png&w=3840&q=75)
![• The randomly generated array is [3, 1, 2, 7, 10]
The sorting took 393429 milliseconds.
The sorted array is [1, 2, 3, 7, 10].
Testing for insertionSort ends.
The words in green may change depending on your testing cases. If users of this
testing method accidentally provide a sorting algorithm name that is not one of
the three (insertionSort, quickSort, or quickSort), this method alert users of this
issue and quit. The alert message is up to you.
For timing the execution of a section of your program, you are recommended to use
System.current TimeMillis() and do some basic research by yourself. Here is an example
of System.currentTimeMillis():
long startTime = System.currentTimeMillis();
// .your program section....
long endTime = System.currentTimeMillis();
long totalTime= endTime - startTime:
System.out.println(totalTime):
You may need to do more research on this to get a better understanding.
The skeleton of the code has been provided to you. You are NOT allowed to change
the parameter numbers or return data types. However, you can add as many
helper methods as you want, and do anything about the helper methods.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1725b614-c61a-470a-b05f-008b5304bcc7%2F39296fa7-6b4b-4f48-a9b5-79f4a79145e1%2Fhs0l4hn_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

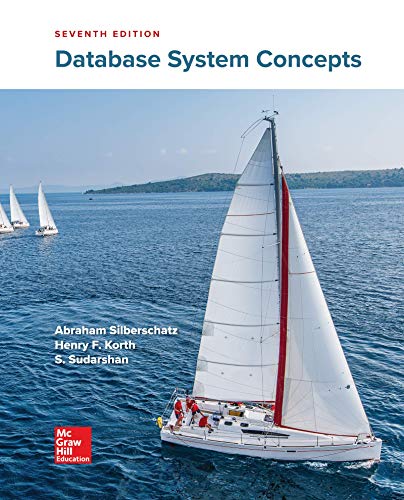
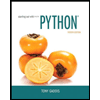
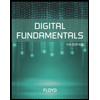
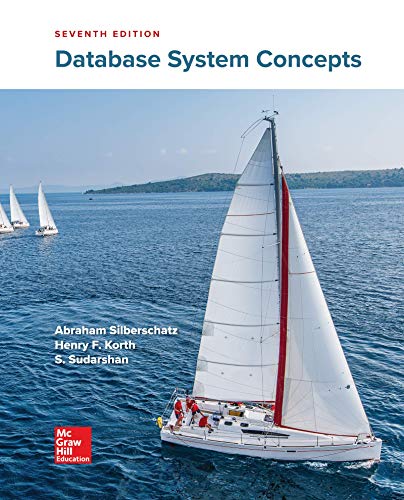
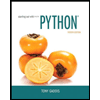
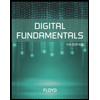
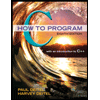
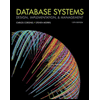
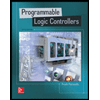