The language used here is in Java. Please answer the question in the screenshot. Please use the code below as a base. import java.util.*; class SortingPack { // just in case you need tis method for testing public static void main(String[] args) { // something } // implementation of insertion sort // parameters: int array unsortedArr // return: sorted int array public static int[] insertionSort(int[] unsortedArr) { // to be removed return unsortedArr; } // implementation of quick sort // parameters: int array unsortedArr // return: sorted int array public static int[] quickSort(int[] unsortedArr) { // to be removed return unsortedArr; } // implementation of merge sort // parameters: int array unsortedArr // return: sorted int array public static int[] mergeSort(int[] unsortedArr) { // to be removed return unsortedArr; } // you are welcome to add any supporting methods }
The language used here is in Java. Please answer the question in the screenshot. Please use the code below as a base.
import java.util.*;
class SortingPack {
// just in case you need tis method for testing
public static void main(String[] args) {
// something
}
// implementation of insertion sort
// parameters: int array unsortedArr
// return: sorted int array
public static int[] insertionSort(int[] unsortedArr) {
// to be removed return unsortedArr;
}
// implementation of quick sort
// parameters: int array unsortedArr
// return: sorted int array
public static int[] quickSort(int[] unsortedArr) {
// to be removed return unsortedArr;
}
// implementation of merge sort
// parameters: int array unsortedArr
// return: sorted int array
public static int[] mergeSort(int[] unsortedArr) {
// to be removed return unsortedArr;
}
// you are welcome to add any supporting methods
}
![1. Implement three sorting algorithms in the file named "SortingPack.java" as three
static methods:
Insertion sorting: Shall be named as "insertionSort"; accepts an integer array as
the only parameter; return the sorted array
public static int[] insertionSort(int[] unsortedArr)
Quick sorting: Shall be named as “quickSort"; accepts an integer array as the
only parameter; return the sorted array
public static int[] quickSort(int|] unsortedArr)
Merge sorting: Shall be named as "mergeSort"; accepts an integer array as the
only parameter; return the sorted array
public static int[] mergeSort(int[] unsortedArr)
The skeleton of the code has been provided to you. You are NOT allowed to change
the parameter numbers or return data types. However, you can add as many
helper methods as you want, and do anything about the helper methods.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1725b614-c61a-470a-b05f-008b5304bcc7%2F804d7383-e383-4186-9931-3ef64b7963bd%2Fd7v0hk_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

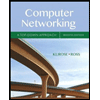
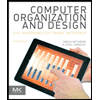
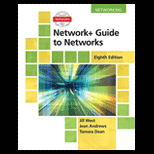
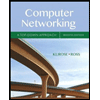
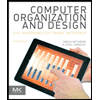
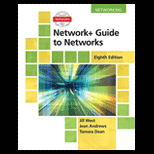
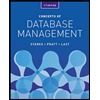
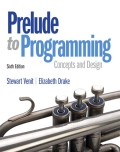
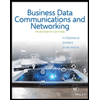