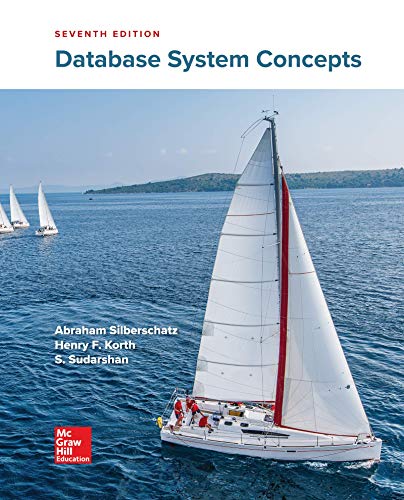
Create the UML Diagram for this java code
import java.util.Scanner;
interface Positive{
void Number();
}
class Square implements Positive{
public void Number() {
Scanner in=new Scanner(System.in);
System.out.println("Enter a number: ");
int a = in.nextInt();
if(a>0) {
System.out.println("Positive number");
}
else {
System.out.println("Negative number");
}
System.out.println("\nThe square of " + a +" is " + a*a);
System.out.println("\nThe cubic of "+ a + " is "+ a*a*a);
}
}
class Sum implements Positive{
public void Number() {
Scanner in = new Scanner(System.in);
System.out.println("\nEnter the value for a: ");
int a = in.nextInt();
System.out.println("Enter the value for b" );
int b= in.nextInt();
System.out.printf("The Difference of two numbers: %d\n", a-b);
System.out.printf("The product of two numbers: %d\n",a*b);
System.out.printf("The quotient of two numbers: %d\n",a/b);
System.out.printf("The largest number: %d\n",Math.max(a, b));
System.out.printf("The lowest number: %d\n",Math.min(a, b));
}
}
class Addition implements Positive{
public void Number() {
int a=10,b=20,c=30,d=40;
int sum=a+b+c+d;
System.out.println("The sum is: "+ sum);
}
}
class main{
public static void main(String args[]) {
Positive a= new Square();
Positive b = new Sum();
Positive c = new Addition();
a.Number();
b.Number();
c.Number();
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- import java.util.Scanner;/** This program computes the time required to double an investment with an annual contribution.*/public class DoubleInvestment{ public static void main(String[] args) { final double RATE = 5; final double INITIAL_BALANCE = 10000; final double TARGET = 2 * INITIAL_BALANCE; Scanner in = new Scanner(System.in); System.out.print("Annual contribution: "); double contribution = in.nextDouble(); double balance = INITIAL_BALANCE; int year = 0; // TODO: Add annual contribution, but not in year 0 do { year++; double interest = balance*(RATE/100); balance = (balance+contribution+interest); } while(balance< TARGET); System.out.println("Year: " + year); System.out.printf("Balance: %.2f%n", balance); }}arrow_forwardpublic static void printIt(int value) { if(value 0) { } System.out.println("Play a paladin"); System.out.println("Play a white mage"); }else { } }else if (value < 15) { System.out.println("Play a black mage"); System.out.println("Play a monk"); } else { public static void main(String[] args) { printit (5); printit (10); printit (15); Play a black mage Play a white mage (nothing/they are all printed) Play a paladin Play a monkarrow_forwardComplete the isExact Reverse() method in Reverse.java as follows: The method takes two Strings x and y as parameters and returns a boolean. The method determines if the String x is the exact reverse of the String y. If x is the exact reverse of y, the method returns true, otherwise, the method returns false. Other than uncommenting the code, do not modify the main method in Reverse.java. Sample runs provided below. Argument String x "ba" "desserts" "apple" "regal" "war" "pal" Argument String y "stressed" "apple" "lager" "raw" "slap" Return Value false true false true true falsearrow_forward
- Type the program's output import java.util.Scanner; public class NumberSearch { public static void findNumber(int number, int lowVal, int highVal, String indentAmt) { int midVal; midVal = (highVal + lowVal) / 2; System.out.print(indentAmt); System.out.print(midVal); if (number == midVal) { System.out.println(" a"); } else { if (number < midVal) { System.out.println(" b"); findNumber(number, lowVal, midVal, indentAmt + " "); } else { System.out.println(" c"); findNumber(number, midVal + 1, highVal, indentAmt + " "); } } System.out.println(indentAmt + "d"); } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int number; number = scnr.nextInt(); findNumber(number, 0, 8, ""); } } Input 5arrow_forwardFix all logic and syntax errors. Results Circle statistics area is 498.75924968391547 diameter is 25.2 // Some circle statistics public class DebugFour2 { public static void main(String args[]) { double radius = 12.6; System.out.println("Circle statistics"); double area = java.Math.PI * radius * radius; System.out.println("area is " + area); double diameter = 2 - radius; System.out.println("diameter is + diameter); } }arrow_forward1 public class Main { 1234567 7 8 9 10 11 ENERGET 12 13 14 15 16 17 18} public static void main(String[] args) { String present = ""; double r = Math.random(); // between 0 and 1 if (r > >>arrow_forward
- using System; class Program{ // Define PrintBoard method public static void PrintBoard() { Console.WriteLine(" X | | "); Console.WriteLine(" | | "); Console.WriteLine(" | O | "); Console.WriteLine(); } public static void Main(string[] args) { PrintBoard(); // Call PrintBoard method PrintBoard(); // Call it again PrintBoard(); // And again! }} This program is really simple and basic to print a tic tac toe board for the user to eventually play the computer in C#. How can it be modified a little bit to print the board and have each tile labeled with a number to identify the space? I'm a beginner and would like to take this step by step in modifying the code. Thank you!arrow_forwardCorrect Code for Java: Enter an integer >> 11 Enter another integer >> 23 The sum is 34 The difference is -12 The product is 253 import java.util.Scanner; public class DebugTwo2 { public static void main(String args[]) { int a, b; Scanner input = new Scanner(System.in); System.out.print("Enter an integer >> "); a = nextInt (); System.out.print("Enter b = nextInt (); System.out.println("The System.out.println("The System.out.println("The } } another integer >> "); sum is " + (a + b)); difference is " + (a - b)); product is + (a*b));arrow_forwardpublic class Qla { Run | Debug public static void main (String [] args) { int n = 1000; int mySum 0; %3D for (int i = n; i >= 0; i--) { %3D if (i % 2 == 0) { mySum += i; } } Stdout.println(mySum);arrow_forward
- Fix all errors to make the code compile and complete. //MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forwardpublic class main { public static void main(String [] args) { Dog dog1=new Dog(“Spark”,2),dog2=new Dog(“Sammy”,3); swap(dog1, dog2); System.out.println(“dog1 is ”+ dog1); System.out.println(“dog2 is ”+ dog2); } public static void swap(Dog a, Dog b) { String nameA = a.getName(); String nameB = b.getName(); a.setName(nameB); b.setName(nameA); } What is the output of the main()?arrow_forwardpublic class Test { } public static void main(String[] args){ int a = 10; System.out.println(a*a--); }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
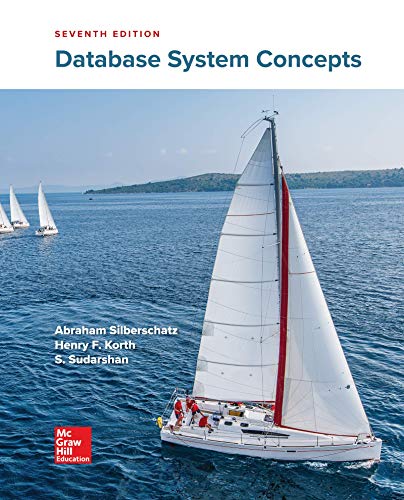
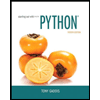
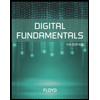
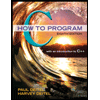
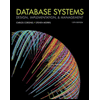
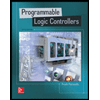