Draw a UML class diagram for the following code: import java.util.Scanner; public class ArrayStack { private int maxSize; private int top; private int[] stackArray; public ArrayStack(int size) { maxSize = size; stackArray = new int[maxSize]; top = -1; } public boolean isEmpty() { return top == -1; } public boolean isFull() { return top == maxSize - 1; } public void push(int value) { if (isFull()) { System.out.println("Stack is full. Cannot push."); return; } stackArray[++top] = value; } public int pop() { if (isEmpty()) { System.out.println("Stack is empty. Cannot pop."); return -1; } return stackArray[top--]; } public void popAll() { while (!isEmpty()) { pop(); } } public int peek() { if (isEmpty()) { System.out.println("Stack is empty. Cannot peek."); return -1; } return stackArray[top]; } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the size of the stack: "); int stackSize = scanner.nextInt(); ArrayStack stack = new ArrayStack(stackSize); while (true) { System.out.println("\nSelect an option:"); System.out.println("1. Push"); System.out.println("2. Pop"); System.out.println("3. Peek"); System.out.println("4. Pop All"); System.out.println("5. Check if stack is empty"); System.out.println("6. Check if stack is full"); System.out.println("7. Exit"); int choice = scanner.nextInt(); switch (choice) { case 1: System.out.print("Enter a value to push: "); int value = scanner.nextInt(); stack.push(value); break; case 2: System.out.println("Popped: " + stack.pop()); break; case 3: System.out.println("Top element: " + stack.peek()); break; case 4: stack.popAll(); System.out.println("All elements popped."); break; case 5: System.out.println("Is stack empty? " + stack.isEmpty()); break; case 6: System.out.println("Is stack full? " + stack.isFull()); break; case 7: System.out.println("Exiting..."); scanner.close(); return; default: System.out.println("Invalid choice."); } } } }
Draw a UML class diagram for the following code:
import java.util.Scanner;
public class ArrayStack {
private int maxSize;
private int top;
private int[] stackArray;
public ArrayStack(int size) {
maxSize = size;
stackArray = new int[maxSize];
top = -1;
}
public boolean isEmpty() {
return top == -1;
}
public boolean isFull() {
return top == maxSize - 1;
}
public void push(int value) {
if (isFull()) {
System.out.println("Stack is full. Cannot push.");
return;
}
stackArray[++top] = value;
}
public int pop() {
if (isEmpty()) {
System.out.println("Stack is empty. Cannot pop.");
return -1;
}
return stackArray[top--];
}
public void popAll() {
while (!isEmpty()) {
pop();
}
}
public int peek() {
if (isEmpty()) {
System.out.println("Stack is empty. Cannot peek.");
return -1;
}
return stackArray[top];
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the size of the stack: ");
int stackSize = scanner.nextInt();
ArrayStack stack = new ArrayStack(stackSize);
while (true) {
System.out.println("\nSelect an option:");
System.out.println("1. Push");
System.out.println("2. Pop");
System.out.println("3. Peek");
System.out.println("4. Pop All");
System.out.println("5. Check if stack is empty");
System.out.println("6. Check if stack is full");
System.out.println("7. Exit");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("Enter a value to push: ");
int value = scanner.nextInt();
stack.push(value);
break;
case 2:
System.out.println("Popped: " + stack.pop());
break;
case 3:
System.out.println("Top element: " + stack.peek());
break;
case 4:
stack.popAll();
System.out.println("All elements popped.");
break;
case 5:
System.out.println("Is stack empty? " + stack.isEmpty());
break;
case 6:
System.out.println("Is stack full? " + stack.isFull());
break;
case 7:
System.out.println("Exiting...");
scanner.close();
return;
default:
System.out.println("Invalid choice.");
}
}
}
}

Step by step
Solved in 3 steps with 1 images

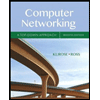
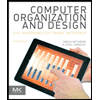
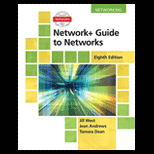
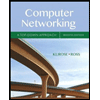
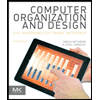
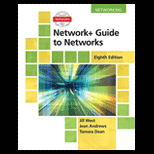
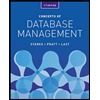
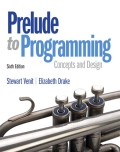
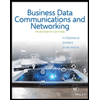