Based on coding below, help me fix all the error until the program can be run properly and neatly #include #include #include #include using namespace std; struct nodeBankAccount { string bank_acc_name; string bank_acc_num; double bank_acc_bal; nodeBankAccount*link; }; nodeBankAccount *head, *rear; nodeBankAccount *current; nodeBankAccount *newNode; nodeBankAccount *deleteBank; nodeBankAccount *previous; nodeBankAccount *found; void addBankAccount() { char answer; int insertOption; do { newNode = new nodeBankAccount; cout << "Fill in the details below" << endl; cout << "Bank Account Name: "; cin>>newNode->bank_acc_name; cout << "Bank Account Number: "; cin>>newNode->bank_acc_num; cout << "Bank Account Balance: " << "RM "; cin>>newNode->bank_acc_bal; newNode->link = NULL; if(head == NULL) { head = newNode; }else{ cout<<"Press 1 to insert the new record at front of the list\n"; cout<<"Press 2 to insert the new record at rear of the list\n"; cout<< "Enter your choice [1 or 2]: "; cin>>insertOption; if(insertOption == 1) { newNode->link = head; head = newNode; }else{ rear->link = newNode; rear = newNode; } } cout<<"\nOne recors has been added into the list "; cout<<"\nAdd more record? Press Y for yes and N for no: "; cin>>answer; }while(toupper(answer) == 'Y'); } void viewBankAccount() { int no=1; int totalDetails = 0; int view; char answer2; cout << "\nChoose view type" << endl; cout << "1 - List View" << endl; cout << "2 - Table View" << endl; cout << "\nEnter your choice: "; cin >> view; switch (view) { case 1: current = head; while (current != NULL) { cout << "\n[Record " << no << "]" << endl; cout << "Bank account name: " << current->bank_acc_name << endl; cout << "Bank account number: " << current->bank_acc_num << endl; cout << "Bank account balance: " << current->bank_acc_bal << endl; no++; current = current->link; totalDetails++; } cout << "\nTotal number of user details: " << totalDetails << endl; break; case 2: cout<bank_acc_name<bank_acc_num<bank_acc_bal<link; totalDetails++; } cout<<"-------------------------------------------------------------------------------------"<> answer2; } void deleteBankAccount() { int searchAccNum; char delete_answer; //delete operation do{ cout << "\n\nEnter account number to be deleted: "; cin >> searchAccNum; current = head; previous = NULL; while(current != NULL) { if(strcmp(current->bank_acc_num, searchAccNum)==0) } deleteBank = current; if(head == rear) { head = NULL; rear = NULL; current = NULL; delete deleteBank; cout << "\n\nRecord has been deleted\n"; }else{ if(current == head) { rear = previous; rear ->link = NULL; current = NULL; delete deleteBank; cout << "\n\nRecord has been deleted\n"; }else{ //node to be deleted is the middle node previous->link = current ->link; current = NULL; delete deleteBank; cout <<"\n\nRecord has been deleted\n"; } } } void searchBankAccount() { int searchAccNum; found = NULL; current = head; if(current == NULL) { cout<<"\n\n List is empty!!!\n\n"; }else{ cout<<"\nEnter bank account number to be searched: "; cin>>searchAccNum; while(current != NULL) { if(strcmp(current->bank_acc_num,searchAccNum)==0 ) { found = current; break; }else{ current = current->link; //move pointer current to the next node } } if(found == NULL) { cout<<"\n\nRecord does not exist!!!\n\n"; }else{ cout<bank_acc_name<bank_acc_num<bank_acc_bal<>menuOption; switch(menuOption) { case 1: addBankAccount(); break; /*case 2: searchBankAccount(); break; case 3: deleteBankAccount(); break; */ case 4: viewBankAccount(); break; case 5: cout<<"\n\n Program End\n\n"; break; default: cout<<"Wrong option. Please reenter your option\n"; } }while(menuOption != 5); return 0; }
Based on coding below, help me fix all the error until the program can be run properly and neatly
#include <iostream>
#include <iomanip>
#include <ctype.h>
#include <string>
using namespace std;
struct nodeBankAccount
{
string bank_acc_name;
string bank_acc_num;
double bank_acc_bal;
nodeBankAccount*link;
};
nodeBankAccount *head, *rear;
nodeBankAccount *current;
nodeBankAccount *newNode;
nodeBankAccount *deleteBank;
nodeBankAccount *previous;
nodeBankAccount *found;
void addBankAccount()
{
char answer;
int insertOption;
do
{
newNode = new nodeBankAccount;
cout << "Fill in the details below" << endl;
cout << "Bank Account Name: ";
cin>>newNode->bank_acc_name;
cout << "Bank Account Number: ";
cin>>newNode->bank_acc_num;
cout << "Bank Account Balance: " << "RM ";
cin>>newNode->bank_acc_bal;
newNode->link = NULL;
if(head == NULL)
{
head = newNode;
}else{
cout<<"Press 1 to insert the new record at front of the list\n";
cout<<"Press 2 to insert the new record at rear of the list\n";
cout<< "Enter your choice [1 or 2]: ";
cin>>insertOption;
if(insertOption == 1)
{
newNode->link = head;
head = newNode;
}else{
rear->link = newNode;
rear = newNode;
}
}
cout<<"\nOne recors has been added into the list ";
cout<<"\nAdd more record? Press Y for yes and N for no: ";
cin>>answer;
}while(toupper(answer) == 'Y');
}
void viewBankAccount()
{
int no=1;
int totalDetails = 0;
int view;
char answer2;
cout << "\nChoose view type" << endl;
cout << "1 - List View" << endl;
cout << "2 - Table View" << endl;
cout << "\nEnter your choice: ";
cin >> view;
switch (view)
{
case 1:
current = head;
while (current != NULL)
{
cout << "\n[Record " << no << "]" << endl;
cout << "Bank account name: " << current->bank_acc_name << endl;
cout << "Bank account number: " << current->bank_acc_num << endl;
cout << "Bank account balance: " << current->bank_acc_bal << endl;
no++;
current = current->link;
totalDetails++;
}
cout << "\nTotal number of user details: " << totalDetails << endl;
break;
case 2:
cout<<endl<<left<<setw(5)<<"No"<<setw(30)<<"Bank account name"<<setw(5)<<"Bank account number"<<setw(30)<<"Bank account balance"<<endl;
cout<<"-------------------------------------------------------------------------------------"<<endl;
current = head;
while(current != NULL)
{
cout<<left<<setw(5)<<no<<setw(30)<<current->bank_acc_name<<setw(5)<<current->bank_acc_num<<current->bank_acc_bal<<endl;
no++;
current = current->link;
totalDetails++;
}
cout<<"-------------------------------------------------------------------------------------"<<endl;
cout<<"\n Total number of user details: "<<totalDetails<<endl;
break;
default: cout << "Wrong input. Please reenter the view option\n";
}
cout << "\nPress Y to go back to MAIN MENU: ";
cin >> answer2;
}
void deleteBankAccount()
{
int searchAccNum;
char delete_answer;
//delete operation
do{
cout << "\n\nEnter account number to be deleted: ";
cin >> searchAccNum;
current = head;
previous = NULL;
while(current != NULL)
{
if(strcmp(current->bank_acc_num, searchAccNum)==0)
}
deleteBank = current;
if(head == rear)
{
head = NULL;
rear = NULL;
current = NULL;
delete deleteBank;
cout << "\n\nRecord has been deleted\n";
}else{
if(current == head)
{
rear = previous;
rear ->link = NULL;
current = NULL;
delete deleteBank;
cout << "\n\nRecord has been deleted\n";
}else{ //node to be deleted is the middle node
previous->link = current ->link;
current = NULL;
delete deleteBank;
cout <<"\n\nRecord has been deleted\n";
}
}
}
void searchBankAccount()
{
int searchAccNum;
found = NULL;
current = head;
if(current == NULL)
{
cout<<"\n\n List is empty!!!\n\n";
}else{
cout<<"\nEnter bank account number to be searched: ";
cin>>searchAccNum;
while(current != NULL)
{
if(strcmp(current->bank_acc_num,searchAccNum)==0 )
{
found = current;
break;
}else{
current = current->link; //move pointer current to the next node
}
}
if(found == NULL)
{
cout<<"\n\nRecord does not exist!!!\n\n";
}else{
cout<<left<<setw(30)<<current->bank_acc_name<<setw(5)<<current->bank_acc_num<<current->bank_acc_bal<<endl;
}
}
}
}
int main()
{
int menuOption;
char answer;
head = NULL; //create list
do
{
cout<<"\n\n\tBank Account Information\n";
cout<<"--------------------------------------\n";
cout<<"\t\tMain Menu\n";
cout<<"--------------------------------------\n";
cout<<"1 - Add Bank Account Details\n";
cout<<"2 - Search Bank Account Details\n";
cout<<"3 - Delete bank account details\n";
cout<<"4 - View details\n";
cout<<"5 - Exit\n";
cout<<"---------------------------------------\n";
cout<<"Enter your option [1, 2, 3, 4 or 5]: ";
cin>>menuOption;
switch(menuOption)
{
case 1: addBankAccount();
break;
/*case 2: searchBankAccount();
break;
case 3: deleteBankAccount();
break;
*/
case 4: viewBankAccount();
break;
case 5: cout<<"\n\n Program End\n\n";
break;
default: cout<<"Wrong option. Please reenter your option\n";
}
}while(menuOption != 5);
return 0;
}

Step by step
Solved in 2 steps with 11 images

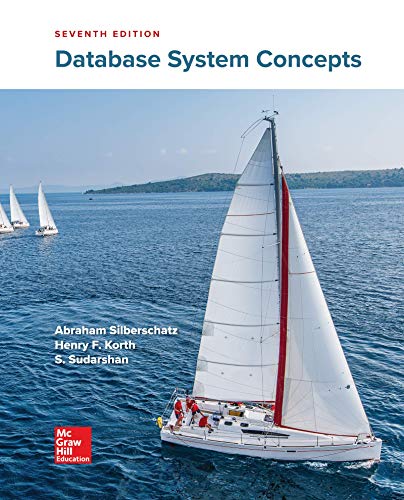
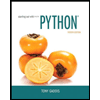
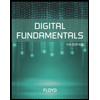
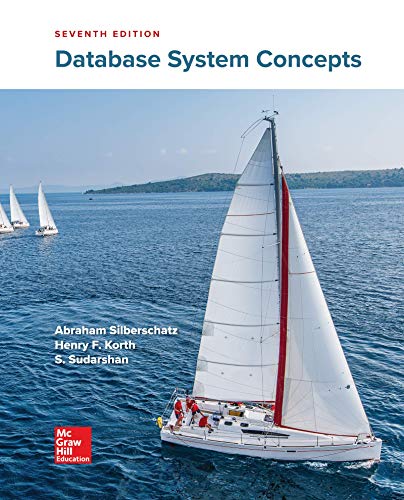
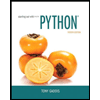
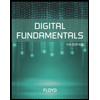
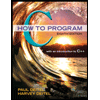
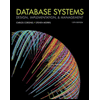
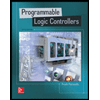