/* Remove node index 0, node index 2 and index 4 from the list*/ //Add your code here
Please help with the following in JAVA
static class Node {
String element;
public Node next;
public Node prev;
public Node(String element){
this.element = element;
}
}
public static void main(String[] args){
Node firstNode=new Node("one"); //create the first node referenced by head
Node second=new Node("two");
head.next=second; second.prev=firstNode; //create the second node and attach it to the first
Node third=new Node("three");
second.next=third; third.prev=second; //create the third node and attach it to the second
Node fourth=new Node("four");
third.next=fourth; fourth.prev=third; //create the fourth node and attach it to the third
Node fifth=new Node("five");
four.next= fifth; fifth.prev= four; //create the fifth node and attach it to the fourth
Node lastNode= fifth;
System.out.println("\n ---The original doubly linked list - forward");
Node<String> current=firstNode;
while(current!=null) {System.out.print(current.element+" "); current=current.next;}
System.out.println("\n ---The original doubly linked list - backward");
current=lastNode;
while(current!=null) {System.out.print(current.element+" ");current=current.prev;}
/* Remove node index 0, node index 2 and index 4 from the list*/
//Add your code here
System.out.println("\n ---The list after removing even-index nodes - forward");
current=firstNode;
while(current!=null) {System.out.print(current.element+" "); current=current.next;}
System.out.println("\n ---The list after removing even-index nodes - backward");
current=lastNode;
while(current!=null) {System.out.print(current.element+" "); current=current.prev;}
System.out.println();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

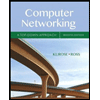
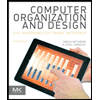
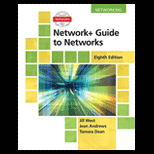
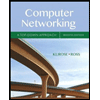
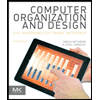
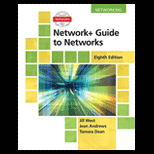
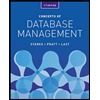
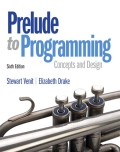
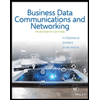