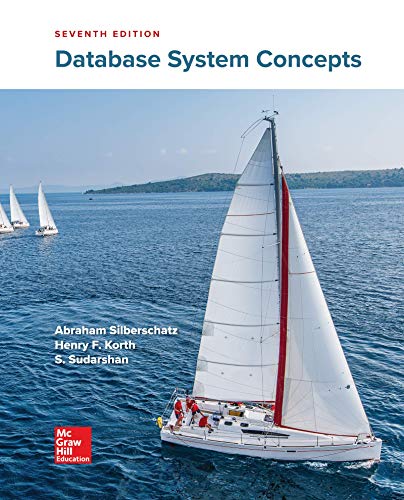
Help me fix all the error in this programme
#include <iostream>
#include <iomanip>
#include <ctype.h>
#include <string.h>
using namespace std;
struct nodeBankAccount
{
char bank_acc_name[30];
string bank_acc_num;
double bank_acc_bal;
nodeBankAccount*link;
};
nodeBankAccount *head, *rear;
nodeBankAccount *current;
nodeBankAccount *newNode;
nodeBankAccount *deleteBank;
nodeBankAccount *previous;
nodeBankAccount *found;
void searchBankAccount()
{
char searchName[30];
int searchNumber = 0;
int searchOption;
char answer;
int searchNumber_No = 0;
found = NULL;
current = head;
if(current == NULL)
{
cout<<"\n\n List is empty!!!\n";
cout<< "Cannot perfom search operation \n\n";
}else{
do
{
cout << "\n\tSearch record\n";
cout << "\t\tSearch Menu\n";
cout<<"\nEnter 1 to search by Bank Account Name\n: ";
cout<<"\nEnter 2 to search by Bank Account Number \n: ";
cout<<"\nEnter 3 to back to Main Menu\n: ";
cout << "Enter your option: ";
cin >> searchOption;
switch (searchOption)
{
case 1: cout<<"\nEnter Bank Account Name to be searched: ";
cin>>ws;
cin.getline(searchName,30);
while (current != NULL)
{
if(strcmp(current->bank_acc_name,searchName)==0 )
{
found = current;
break;
}else{
current = current->link;
}
}
if(found == NULL)
{
cout<<"\n\nRecord does not exist!!!\n\n";
}else{
cout<<endl<<left<<setw(30)<<"Bank Account Name"<<setw(30)<<"Bank Account Number"<<setw(10)<<"Bank Account Balance"<<endl;
cout<<left<<setw(30)<<current->bank_acc_name<<setw(30)<<current->bank_acc_num<<current->bank_acc_bal<<endl;
}
if (searchName == 0)
{
cout<<"\n\nRecord does not exist!!!\n\n";
}else{
cout << "\nTotal number of records found: " << searchName << endl;
}
break;
case 2: cout << "\n\nEnter Bank Account Number to be searched: ";
cin >> searchNumber;
cout<<endl<<left<<setw(30)<<"Bank Account Name"<<setw(30)<<"Bank Account Number"<<setw(10)<<"Bank Account Balance"<<endl;
while (current != NULL)
{
if(current->bank_acc_num,searchNumber)
{
cout<<left<<setw(30)<<current->bank_acc_name<<setw(30)<<current->bank_acc_num<<current->bank_acc_bal<<endl;
searchNumber_No++;
}
current = current->link;
}
if (searchNumber_No == 0)
{
cout << "\n\nRecord does not exist!!\n\n";
}else{
cout << "\nTotal Number of Records found: " << searchNumber_No << endl;
}
break;
case 3: cout << "\n\nBack to Main Menu" << endl;
cin >> answer;
}
}
}
void viewBankAccount()
{
int no=1;
int totalDetails = 0;
cout<<endl<<left<<setw(5)<<"No."<<setw(30)<<"Bank Account Name"<<setw(30)<<"Bank Account Number"<<setw(10)<<"Bank Account Balance"<<endl;
current = head;
while(current != NULL)
{
cout<<left<<setw(5)<<no<<setw(30)<<current->bank_acc_name<<setw(30)<<current->bank_acc_num<<current->bank_acc_bal<<endl;
no++;
current = current->link;
totalDetails++;
}
cout<<"\n Total number of user details: "<<totalDetails<<endl;
}
int main()
{
int menuOption;
char answer;
head = NULL;
do
{
cout<<"\n\n\tBank Account Details\n";
cout<<"\t\tMain Menu\n";
cout<<"1 - Add Bank Account details\n";
cout<<"2 - Search Bank Account details\n";
cout<<"3 - Delete bank account details\n";
cout<<"4 - View details\n";
cout<<"5 - Exit\n";
cout<<"Enter your option [1, 2, 3, 4 or 5]: ";
cin>>menuOption;
switch(menuOption)
{
case 2: searchBankAccount();
break;
case 4: viewBankAccount();
break;
case 5: cout<<"\n\n Program End\n\n";
break;
default: cout<<"Wrong option. Please reenter your option\n";
}
}while(menuOption != 5);
return 0;
}

Step by stepSolved in 2 steps with 6 images

- Part-2 Debugging Debug#0int main(){char* ptr = nullptr;int size; cout << "Enter the size of the sentence: "; cin >> size; ptr = new char[size];char* ptr2 = ptr; cout << static_cast<void*>(&ptr) << endl;cout << static_cast<void*>(&ptr2) << endl; cout << "\nEnter your sentence:" << endl;for (int i = 0; i < size; i++){cin >> *(ptr + i);} cout << *ptr << endl;cout << ptr[size - 1]; while (static_cast<void*>(&ptr) <= static_cast<void*>(&ptr[size -1])){cout << "HI" << endl;cout << static_cast<void*>(&ptr) << endl;static_cast<void*>(&ptr);ptr++;} }arrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forwardplease go in to the detail and explain the purpose and function to each line & function of code listed below. Dsecribe each line. #include <stdio.h>//including headers#include <string.h>#include <stdlib.h> struct node{//structure intialization int data; struct node *next; }; #include <assert.h> typedef struct Info_ { char name[100]; } Info; typedef struct Compar_ { Info info; struct Compar_* next; } Compar; void print_comparisons(Compar* CP)//comparison method { assert(CP); Compar* cur = CP; Compar* next = cur->next; for (; next; cur = next, next = next->next) { if (strcmp(cur->info.name, next->info.name) == 0) printf("Same name\n"); else printf("Diff name\n"); } } struct node *head, *tail = NULL; void addNode(int data) {//adding node method struct node *newNode = (struct node*)malloc(sizeof(struct node)); newNode->data = data; newNode->next = NULL; if(head == NULL) { head = newNode; tail = newNode; } else { tail->next =…arrow_forward
- 6. sum_highest_five This function takes a list of numbers, finds the five largest numbers in the list, and returns their sum. If the list of numbers contains fewer than five elements, raise a ValueError with whatever error message you like. Sample calls should look like: >>> sum_highest_five([10, 10, 10, 10, 10, 5, -17, 2, 3.1])50>>> sum_highest_five([5])Traceback (most recent call last): File "/usr/lib/python3.8/idlelib/run.py", line 559, in runcode exec(code, self.locals) File "<pyshell#44>", line 1, in <module> File "/homework/final.py", line 102, in sum_highest_five raise ValueError("need at least 5 numbers")ValueError: need at least 5 numbersarrow_forwardQ# Describe what a data structure is for a char**? (e.g. char** argv) Group of answer choices A. It is a pointer to a single c-string. B. It is an array of c-strings C. It is the syntax for dereferencing a c-string and returning the value.arrow_forward/* yahtC */ #include <stdio.h> #include <string.h> #include <stdlib.h> #include <time.h> void seed(int argc, char ** argv){ srand(time(NULL)); // Initialize random seed if (argc>1){ int i; if (1 == sscanf(argv[1],"%d",&i)){ srand(i); } } } void instructions(){ printf("\n\n\n\n" "\t**********************************************************************\n" "\t* Welcome to YahtC *\n" "\t**********************************************************************\n" "\tYahtC is a dice game (very) loosely modeled on Yahtzee\n" "\tBUT YahtC is not Yahtzee.\n\n" "\tRules:\n" "\t5 dice are rolled\n" "\tThe user selects which dice to roll again.\n" "\tThe user may choose to roll none or all 5 or any combination.\n" "\tAnd then the user selects which dice to roll, yet again.\n" "\tAfter this second reroll the turn is scored.\n\n" "\tScoring is as follows:\n" "\t\t*\t50 points \t5 of a kind scores 50 points.\n" "\t\t*\t45 points \tNo pairs (all unique) scores 45…arrow_forward
- #ifndef LLCP_INT_H#define LLCP_INT_H #include <iostream> struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endifarrow_forwardCreate pseudocode for the following #include <iostream> #include <string> #include <cstdlib> #include <ctime> using namespace std; char water[10][10] = {{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'}}; void createBoard(int &numShip); void promptCoords(int& userX, int &userY); void shipGen(int shipX[] ,int shipY[], int &numShip); void testCoords(int &userX, int &userY, int shipX[], int shipY[], int &numShip, int& victory); void updateBoard();arrow_forward#include <iostream> #include <cmath> #include <iomanip> using namespace std; int calc_qty(int qty); void receipt( int qty, int qty2); int calc_qty(int qty); void coffee_size(int qty, int size, int choice, int qty2); void slices(); void beanounces(); int main() { int qty = 0, order = 0, size = 0, qty2 = 0; receipt (qty,qty2); calc_qty (qty); do { //displays the menu cout<<" Please select an item on the menu you would like to buy."<<endl; cout<<"Enter 1 for Coffee\n"<<"Enter 2 for cheese cake\n"<<"Enter 3 for coffee beens\n"<<endl; cin>>choice; } switch (choice) { case 1: coffee_size(qty, size, order, qty2); break; case 2: slices(); break; case 3: beanounces(); break; case 4: cout<<"Enjoy your day"<<endl;…arrow_forward
- here is a and b part plz solve c part #include <iostream>#include <string.h>#include <stdlib.h>#include <sstream>#include <cmath>using namespace std; int main(){ /*Variable declarations*/int higestDegree,higestDegree1;int diffDegree,smallDegree;std::stringstream polynomial,addpolynomial;/*prompt for degree*/std::cout<<"Please enter higest degree of polynomial"<<std::endl;std::cin>>higestDegree; std::cout<<"Please Enter highest degree of 2nd polynomial"<<std::endl;std::cin>>higestDegree1;int *cofficient=new int[higestDegree+1];//cofficient array creation for 1st polynomailint *cofficient1=new int[higestDegree1+1];//cofficient array creation for 2st polynomail /*taking cofficient for 1st polynomial*/std::cout<<"Please enter cofficient of each term from higest degree to lowest for 1st polynomial"<<std::endl;for(int i=0;i<=higestDegree;i++){std::cin>>cofficient[i];}/*taking cofficient for 2st…arrow_forwardplease use DEQUE #include <iostream>#include <string>#include <deque> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","TUL"}; int main(){// define stack (or queue ) herestring origin;string dest;string citypair;cout << "Loading the CONTAINER ..." << endl;// LOAD THE STACK ( or queue) HERE// Create all the possible Airport combinations that could exist from the list provided.// i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ...// DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached shell program (AirportCombos.cpp), create a list of strings to process and place on a STL DEQUE container. Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible…arrow_forwardinitial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
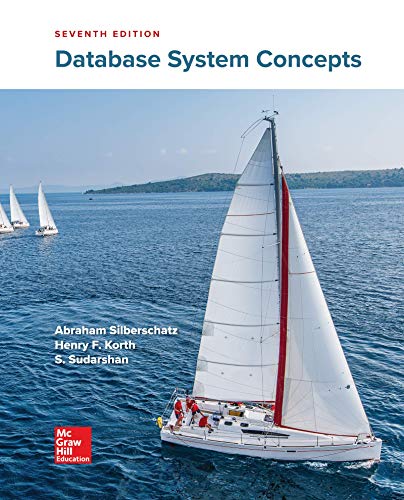
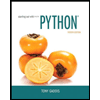
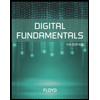
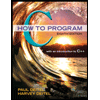
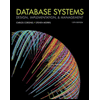
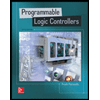