#include #include #include #include using namespace std; int main() { int myints[] = {}; list l, l1 (myints, myints + sizeof(myints) / sizeof(int)); list::iterator it; int choice, item; while (1) { cout<<"\n---------------------"<>choice; switch(choice) { case 1: cout<<"Enter value to be inserted at the front: "; cin>>item; l.push_front(item); break; case 2: cout<<"Enter value to be inserted at the end: "; cin>>item; l.push_back(item); break; case 3: cout<<"Front Element of the List: "; cout<
#include <iostream>
#include <list>
#include <string>
#include <cstdlib>
using namespace std;
int main()
{
int myints[] = {};
list<int> l, l1 (myints, myints + sizeof(myints) / sizeof(int));
list<int>::iterator it;
int choice, item;
while (1)
{
cout<<"\n---------------------"<<endl;
cout<<"***List Implementation***"<<endl;
cout<<"\n---------------------"<<endl;
cout<<"1.Insert Element at the Front"<<endl;
cout<<"2.Insert Element at the End"<<endl;
cout<<"3.Front Element of List"<<endl;
cout<<"4.Last Element of the List"<<endl;
cout<<"5.Size of the List"<<endl;
cout<<"6.Display Forward List"<<endl;
cout<<"7.Exit"<<endl;
cout<<"Enter your Choice: ";
cin>>choice;
switch(choice)
{
case 1:
cout<<"Enter value to be inserted at the front: ";
cin>>item;
l.push_front(item);
break;
case 2:
cout<<"Enter value to be inserted at the end: ";
cin>>item;
l.push_back(item);
break;
case 3:
cout<<"Front Element of the List: ";
cout<<l.front()<<endl;
break;
case 4:
cout<<"Last Element of the List: ";
cout<<l.back()<<endl;
break;
case 5:
cout<<"Size of the List: "<<l.size()<<endl;
break;
case 6:
cout<<"Elements of the List: ";
for (it = l.begin(); it != l.end(); it++)
cout<<*it<<" ";
cout<<endl;
break;
case 7:
exit(1);
break;
default:
cout<<"Wrong Choice"<<endl;
}
}
return 0;
}
TASK:
Run the above code and display the output of list as your Data of Birth, e.g., if your DOB is 17-02-1994, then output should be:
1 7 0 2 1 9 9 4
Also perform deletion from front and rare of list and attach output.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

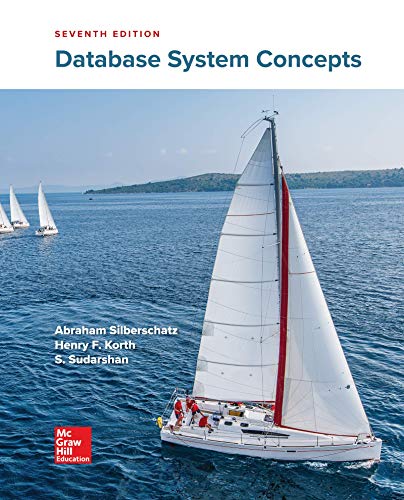
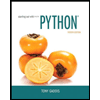
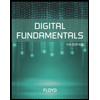
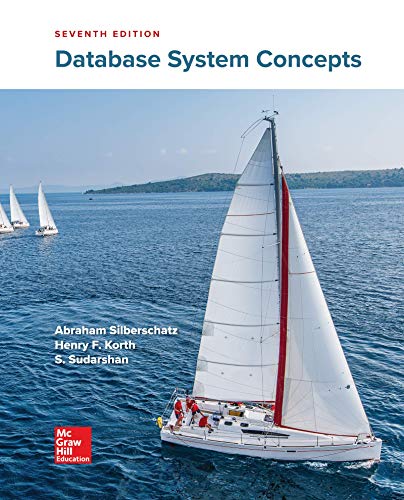
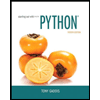
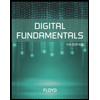
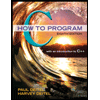
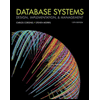
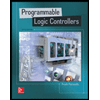