1. Given the attached program code, answer the following: (a) Match each function name with its task. Function Name Task F1 Insert a node at the front of the list. F2 Insert a node after a given node. F3 Delete a node in a specific position. F4 Insert a node at the end of the list. F5 Create the first node in the list. F6 Display all the nodes of the list. (b) What is the result of program execution with the data given in main(). (c) Write a function to count the number of nodes in the list.
1. Given the attached program code, answer the following: (a) Match each function name with its task. Function Name Task F1 Insert a node at the front of the list. F2 Insert a node after a given node. F3 Delete a node in a specific position. F4 Insert a node at the end of the list. F5 Create the first node in the list. F6 Display all the nodes of the list. (b) What is the result of program execution with the data given in main(). (c) Write a function to count the number of nodes in the list.
Chapter3: Data Representation
Section: Chapter Questions
Problem 1VE
Related questions
Question

Transcribed Image Text:Question4 (Linked List):
1. Given the attached program code, answer the following:
(a) Match each function name with its task.
Function Name
Task
F1
Insert a node at the front of the list.
F2
Insert a node after a given node.
F3
Delete a node in a specific position.
F4
Insert a node at the end of the list.
F5
Create the first node in the list.
F6
Display all the nodes of the list.
(b) What is the result of program execution with the data given in main().
(c) Write a function to count the number of nodes in the list.

Transcribed Image Text:The program code:
#include <iostream>
// ***** F5 ****
using namespace
std; struct Node
int data;
void F5 (int value, int position)
{
* curr,
*newNode,
*succ, *head;
newNode = new Node;
newNode->data = value;
newNode->next = NULL;
Node
*pred,
Node* next;};
// ***** F1 ****
void F1 ( int n)
{
Node *head%3new Node;
head->data = n;
curr=head;
for (int i=1;i<=position; i++)
head->next =NULL; }
{pred=curr; curr = curr->next; }
curr=head;
// ***** F2 ****
void F2 (int value)
for (int i=1;i<=position+1;i++)
{succ=curr; curr = curr->next;}
pred->next=newNode;
= succ;
Node *newNode, *head, *curr;
newNode = new Node;
newNode-
newNode->next
>data = value;
newNode->next =
NULL;
if (head=-NULL)
// ***** F6 ****
head = newNode;
F6(int
else
position)
Node
{ curr = head;
while
{
*curr, *pred, *succ, *head;
curr=head;
(curr->next!=NULL)
if (position==1)
{curr = curr->next; head%3curr;}
curr = curr->next;
curr-
>next = newNode;
else
{
for (int i=1;i<=position-1;i++)
{pred=curr;curr curr->next; }
curr=head;
// ***** F3 ****
void F3 ()
{Node *curr;Node
*head;
while (curr!=NULL)
{cout
for (int i=1;i<=position+1;i++)
(succ=curr;curr = curr->next;}
pred->next = succ;
curr = head;
くく
curr->data
くく
->
";
curr
curr->next;
// ***** main
**** int main ()
cout
くく
"NULL";
cout <<
endl;
{ F1 (5); F2 (10);
F2 (15) ; F2 (20);
F2 (25); F4 (-20);
F3 () ;
F5 (500, 3); F3 ();
F5 (600,3); F3 ();
F6 (1); F3 ();
F6 (1); F3 ();
F6 (4); F3 ();
return 0;
// **** * F4 ****
void F4 (int value)
{ Node *newNode, *head,
* curr;
newNode = new Node;
newNode->data = value;
newNode->next = NULL; if
(head!=NULL)
>next=head;
newNode-
head=newNode;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Recommended textbooks for you
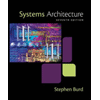
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
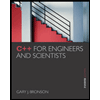
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
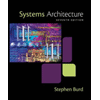
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
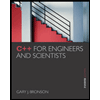
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr