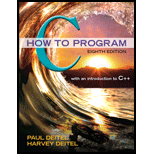
Concept explainers
Find the error in each of the following. (Note: There may be more than one error.)
- The following code should print whether a given integer is odd or even:
- The following code should input an integer and a character and print them. Assume the usere types as input 100 A.
- The following code should output the odd integers from 999 to 1:
- The following code should output the even integers from 2 to 100:
- The following code should sum the integers from 100 to 150 (assume total is initialized to 0):
(a)

To find and debug the error using proper syntax and logic in the given program.
for (int x = 100; x >= 1;--x) { printf ("%d\n", x); }
Explanation of Solution
Given information:
- For (x = 100, x >= 1, ++x) {
- printf ("%d%n", x);
- }
Explanation:
- Loop variable needs to be initialized as an intthat is int x.
- The syntax of a for loop is not followed as well as C is the case-sensitive language that is For and for are the different for C compiler.
- Therefore, For should be written as for and the commas need to be changed into the semi-colons (;) inside the for-loop parenthesis.
- The correct syntax of a for loop is:
- The logical error wasthat loop going to execute the infinite times as it’s variablestarts from 100 and is updating its value by incrementing 1 at every run. Therefore, at every update, the value of x is greater than 1. Thus, instead of updating x as ++x use -- x.
- The last error is that to print every integer in newline \n is used instead of %n .
for (Initializing variable; Testing condition; Updating variable){
//for block to write the statements
}
Output:
(b)

To find and debug the error using proper syntax and logic in the given program to print whether the given integer is odd or even.
switch (value % 2) { case 0: puts ("Even integer"); break; case 1: puts ("Odd integer"); break; }
Explanation of Solution
Given information:
switch (value % 2) { case 0: puts ("Even integer"); case 1: puts ("Odd integer"); }
Explanation:
The cases act as the start point for every switch and case block where the switch takes the data and matches it with the corresponding case. Therefore, if any case is matched then all the statements from that case will be executed till it terminates or breaks. Therefore, cases should have a break keyword to exit the switch and case block, once the case is matched.
(c)

To find and debug the error using proper syntax and logic in the given program to print an integer value and the character value A as 100 A.
scanf ("%d", &intVal);
scanf ("\n%c", &charVal);
printf ("Integer: %d\nCharacter: %c\n", intVal, charVal);
Explanation of Solution
Given information:
scanf("%d", &intVal);
charVal = getchar ();
printf("Integer: %d\nCharacter: %c\n", intVal, charVal);
Explanation:
To stop reading the variable charValblank character, the second statement need to skip the preceding blanks when the user enters the integer value and press return, to rectify this scanf must be used. That is getchar() reads the next line after pressing by inputing the integer value.
Output:
(d)

To find and debug the error using proper syntax and logic in the given program
Explanation of Solution
Given information:
for (x = .000001; x == .0001; x += .000001)
printf("%.7f\n", x);
Explanation:
The numbers are quite small to be compared and the for-loop must not compare the floating-point number using == as it causes impression which might cause the infinite loop. Thus, it is recommended to use integer values as int datatype in the for-loop.
(e)

To find and debug the error using proper syntax and logic in the given program to print odd number from 999 to 1.
for (int x = 999; x >= 1; x -= 2) if (x % 2 == 1) printf ("%d\n", x);
Explanation of Solution
Given information:
for(x = 999; x >= 1; x += 2)
printf("%d\n", x);
Explanation:
- Loop variable need to be initialized as intthat is int x.
- To print the odd values from 999 to 1 the loop variable should decrease by two, instead of increasing by 2 as we need to decrease the value.
- The correct syntax of a for loop is:
- If condition is used to check whether the variable x is odd or not.
for (Initializing variable; Testing condition; Updating variable) {
//for block to write a statement
}
Output:
(f)

To find and debug the error using proper syntax and logic in the given program to print even numbers between 2 to 100.
int counter = 2;
do{
if(counter % 2== 0)
printf("%u\n", counter);
counter += 2;
} while(counter <= 100);
Explanation of Solution
Given information:
counter = 2;
Do {
if (counter % 2== 0) {
printf ("%u\n", counter);
}
counter += 2;
} While (counter < 100);
Explanation:
- The variable need to be initialized as intthat is int counter.
- The programming language - C is the case-sensitive language that is Do and do are different for C compiler and the same goes for While and while.
- To print the even values to 100 inclusively whileloop needs to <= instead of <.
Output:
(g)

To find and debug the error using proper syntax and logic in the given programto find the sum of the numbers between 100 to 150.
int total = 0; for(int x = 100; x <= 150; ++x){ total += x; } printf("%d",total);
Explanation of Solution
Given information:
for(x = 100; x <= 150; ++x);{
total += x;
}
Explanation:
- The declaration of the total variable is missing.
- Loop variable needs to be initialized as an intthat is int x.
- The syntax of a for loop is not followed as there should be no comma (,) after the parenthesis.
- The correct syntax of a for loop is:
- The logical error was that there is no print statement.
for (Initializing variable; Testing condition; Updating variable) {
//for block to write statement
}
Output:
Want to see more full solutions like this?
Chapter 4 Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Concepts of Programming Languages (11th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
C++ How to Program (10th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Database Concepts (7th Edition)
- “Fix” the following 3 lines of code so that the print the last line of code prints correctly: age == input(Greetings! How old are you?) age == age + 1 print["Happy birthday to you! You are" + age + years old!]arrow_forwardConvert the following statements into intermediate code: int r=0;for (int i=0; i<10; i++) r=r+i;arrow_forward2- Number of basic operations of the following statement is equal to : for ( int i = 0 ; i< = 1 ; ++i) System.out.println( "X+1"+"Y"); A- 5 B- 6 C- 7 D- Nonearrow_forward
- Another way to write this code. #Declare variables and constants year = 0 YEARLY_RISE = 1.80 #Print header print("Year\t\tRise (in millimeters)") print("\n--------------------------------------\n") #Iterate 25 times for i in range(1, 26): #Calculate the rise in millimeters print(f"{i}\t\t{YEARLY_RISE * i:.2f}")arrow_forwardWrite TRUE if the truth value of the statement is incorrect, otherwise write FALSE.arrow_forwardFactorial) The factorial of a nonnegative integer n is written n! (pronounced “n factorial”) and is defined as follows:For example, 5!= 5.4.3.2.1 , which is 120. Use while statements in each of the following:A. Write a program that reads a nonnegative integer and computes and prints its factorial.B. Write a program that estimates the value of the mathematical constant e by using the formula:Prompt the user for the desired accuracy of e (i.e., the number of terms in the summation).C. Write a program that computes the value of by using the formula Prompt the user for the desired accuracy of e (i.e., thenumber of terms in the summation).arrow_forward
- Test Average Write a program that asks for five test scores. The program should calculate the average test score and display it. The number displayed should be formatted in fixed-point notation, with one decimal place of precision. C++arrow_forwardint f(int &k){k++;return k * 2;}int main(){int i = 1, j = -1;int a, b, c;a = f(i) + i/2;b = j + f(j) + f(j);c = 2 * f(j);return 0;} What are the values of a, b and c id the operands in the expressions are evaluated from left to right and then what are the values when its evaluated right to left?arrow_forwardGive proper explanation Write a shell scrip program to prompt the user to enter a negative integer for: m and displays the sum of all the odd integers in the range m to 0 inclusive. For the sake of simplicity assume the user enters a negative integer. For example, if the user enters -5 for m the display output is: -9arrow_forward
- 1. a)What is the value of num when the following code snippet runs successfully? int num = 100; if (num < 100) { if (num < 50) { num -= 5; } else { num -= 10; } } else { if (num > 150) { num += 5; } else { num += 10; } }arrow_forward1. What is the value of x and y for the following code: int y = 10; int x = ++y;arrow_forwardBody Mass Index is a simple calculation using a person's height and weight. The formula is BMI = kg/m2 where kg is a person's weight in kilograms and m2 is their height in meters squared. Calculate a persons Body Mass index using their weight in pounds. This means you must first convert pounds to kilograms (kg) then apply the formula above. In converting pounds to kg, 1 pound = 0.453592 kg. So to convert pounds to kg, either multiply the pound value by 0.45359237 or divide by 2.2046226218. Then you must convert their height in inches to meters. The length in meters is equal to the inches multiplied by 0.0254. Write a program that accepts a users height as an integer in inches and their weight in pounds. Convert those inputs to their metric equivalents outlined above and determine their body mass index. Display what the conversions from and to their metric equivalents are. Display the body mass index and the category it belongs to.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
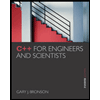
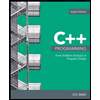