What are literals in programming?
A literal in computer science is used to express a fixed value in source code. They cannot be modified. Almost all programs have these fixed values or literals.
Literal can be of type Boolean, integer, character or string.
Purpose of literals
Literals can be associated with the variables or can be used as an identifier in Java, python, or other languages. For example, number 2 is an integer literal whereas 'dog' is a string literal. Literals are important as they provide a meaning to specific values in a program. There are six types of literals.
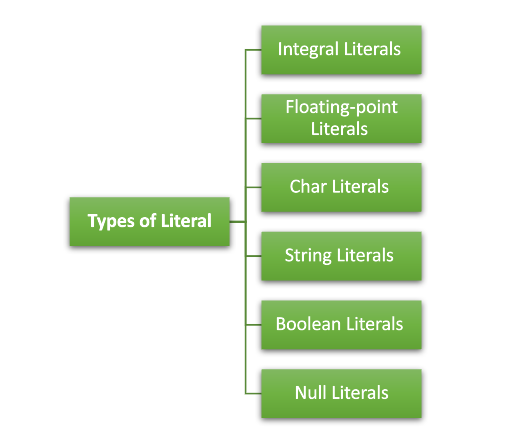
These literals also have distinct subtypes.
Integral literals
There are two kinds of integer literals:
Integer literals (Prefixes) are further subdivided into:
- Decimal integer literals
- Hexadecimal integer literals
- Octal integer literals
- Binary integer literals
Decimal integer literals (base 10)
A decimal integer literal can have any of the numbers between 0 to 9. The first digit cannot be 0.
Hexadecimal integer literals (base 16)
The literal integer begins with a number 0. It is followed by either x or X further followed by the combination of numbers between 0 and 9 and letters between a and f, A or F.
The numbers 10 to15 are represented by the letters A (or a) through F (or f). Although Java is case-sensitive, it also allows the usage of either uppercase or lowercase characters in code for hexadecimal literals.
Octal integer literals (base 8)
An octal integer literal starts with the number 0 and can include any of the numbers between 0 to 7. When assigning an octal literal in programming, a number must have the prefix 0.
Binary integer literals (base 2)
It can be expressed by using binary literals, which are, 0 and 1 with the prefix 0b or 0B.
Integer literals (suffixes)
Depending on the data type, they can also be represented in a variety of ways.
- int- There is no need for a suffix because integer value constants are assigned as int data types by default.
- Unsigned int- The character u or U is used at the end of an integer constant.
- Long int- This integer constant has the character l or L at the end.
- Unsigned long int- The character ul or UL is used at the end of the integer constant.
- Long long int- The character ll or LL is present at the end of the integer constant.
- Unsigned long long int- The character ull or ULL is present at the end of the integer constant.
Floating-point literals
A floating-point constant is made up of the following elements:
- An integral part
- A decimal point
- A fractional part
- An exponent part
- An optional suffix
Floating-point number with a single-precision (4 bytes) signifies either f or F
Floating-point integer with double precision (8 bytes) signifies d or D.
The integral and fractional portions are both composed of decimal digits. Either the integral or fractional component can be omitted or else the decimal point or the exponent portion can be omitted, but not both. The suffix f or F denotes a float type, whereas the suffix l or L suggests a long double type. The floating-point constant has the type double if no suffix is given.
Char literals
A character literal expression is made up of a single character. The character is enclosed by single quotation marks. A prefix L can be used to mark a char literal. C and C++ are the programming languages that predominantly use character literals.
A single character contained in a single quotation is known as a literal to a char data type. For instance, char ch = 'a';
Escape sequence
Every escape character can be defined as a char literal.
For instance, char ch = ‘n'
String literals
A string literal is a set of characters and the escape sequences that remain enclosed in double quotation marks. A string literal that lacks the prefix L is referred to as an ordinary or narrow string literal, where multiple spaces within a string literal are preserved. To indicate a newline character as part of a string, the escape sequence 'n' can be used. To portray a backslash character as part of a string, the \ escape sequence can be used. A single quote mark sign can be represented by itself or with the escape sequence '. To indicate a double quote mark, the escape sequence " can be used.
Java provides implicit string literal concatenation, where adjacent string literals can get concatenated or joined into a single literal during the compile time. Concatenation could be done using the '+' operator. Three """ characters can be used in the Java multi-line declaration.
Although string literals cannot contain unescaped line feed or newline characters, the compiler always evaluates compile-time expressions. Unicode escape sequences or special characters can be used as backlash characters inside the string and character literal to skip special characters, as indicated in the table below:
Value | Escape sequence |
Newline | n |
Backslash | \ |
Horizontal tab | t |
Question mark | ? or ? |
Vertical tab | v |
Single-quote | ' |
Backspace | b |
Double quote | " |
Carriage return | r |
The null character |