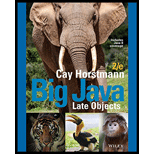
Concept explainers
Given Program:
The program given in the textbook is given here with comments for better understanding.
File Name: TaxReturn.java
// Class definition
public class TaxReturn
{
// Declare and initialize the required variables
public static final int SINGLE = 1;
public static final int MARRIED = 2;
private static final double RATE1 = 0.10;
private static final double RATE2 = 0.25;
private static final double RATE1_SINGLE_LIMIT = 32000;
private static final double RATE1_MARRIED_LIMIT = 64000;
private double income;
private int status;
/*Constructs a TaxReturn object for a given income and marital status.
@param anIncome the taxpayer income
@param aStatus either SINGLE or MARRIED */
// Method definition
public TaxReturn(double anIncome, int aStatus)
{
income = anIncome;
status = aStatus;
}
// Method definition
public double getTax()
{
// Declare and initialize the required variables
double tax1 = 0;
double tax2 = 0;
/* If the entered status is "Single", compute income tax based on their income */
if (status == SINGLE)
{
/* Check whether the income is less than or equal to $32000 */
if (income <= RATE1_SINGLE_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $32000, compute the tax */
else
{
tax1 = RATE1 * RATE1_SINGLE_LIMIT;
tax2 = RATE2 * (income - RATE1_SINGLE_LIMIT);
}
}
/* If the entered status is "Married", compute income tax based on their income */
else
{
/* Check whether the income is less than or equal to $64000 */
if (income <= RATE1_MARRIED_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $64000, compute the tax */
else
{
tax1 = RATE1 * RATE1_MARRIED_LIMIT;
tax2 = RATE2 * (income - RATE1_MARRIED_LIMIT);
}
}
// Return the tax to the main function
return tax1 + tax2;
}
}
File Name: TaxCalculator.java
// Import the required package
import java.util.Scanner;
/**
This program calculates a simple tax return.
*/
// Class definition
public class TaxCalculator
{
// Main class declaration
public static void main(String[] args)
{
// Create an object for scanner class
Scanner in = new Scanner(System.in);
// Prompt the user to enter the income
System.out.print("Please enter your income: ");
// Store the entered income in the variable
double income = in.nextDouble();
// Prompt the user to enter marital status
System.out.print("Are you married? (Y/N) ");
// Store the entered value in a variable
String input = in.next();
// Declare the variable
int status;
/* Check whether the user input value for marital status is "Y" */
if (input.equals("Y"))
{
/* If it is "Y", store the taxreturn value for a married person in the variable */
status = TaxReturn.MARRIED;
}
/* Check whether the user input value for marital status is "N" */
else
{
/* If it is "N", store the taxreturn value for a single person in the variable */
status = TaxReturn.SINGLE;
}
// Create an object for TaxReturn class
TaxReturn aTaxReturn = new TaxReturn(income, status);
// Display the tax return value based on the user input
System.out.println("Tax: "
+ aTaxReturn.getTax());
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Big Java Late Objects
- A real estate agency pays a sales representative monthly minimum income of 1000, 54 TL, additional allowance according to his/her title, plus 200 TL for each property sold by the sales representative in that month and a certain amount of the sales amount. Additional allowance amounts by title and bonus rates are given in the tables below: Title Additional Allowance (TL) Trainee (1) Beginner (2) 250 350 Expert (3) 500 Senior (4) 750 Sales Amount (TL) Bonus Rate (%) 0- 499.999,99 0.25 500.000- 999.999,99 0.5 1.000.000- 1.999.999,99 0.75 2.000.000-... 1 By adhering the rules stated above, find the total salary amount of a sales representative for a particular month, by obtaining the title of a sales representative from the user (1 for the trainee, 2 for the novice, 3 for the specialist, 4 for the senior), the number of real estate sold this month and the total sales amount made by the sales representative. Write a MATLAB code that prints the total salary on the screen using the…arrow_forwardusing C programming language: Create Struct Employee with his information and input an array of Employees then print itarrow_forwardA multiple-choice quiz consists of 5 questions with 4 possible choices. In how many ways can a student answer the questions if the student answers every question (i.e. they don't leave any question blank). Group of answer choices. 1024,512,2048,256arrow_forward
- Computer sciencearrow_forwardPlease right solution only please fast u will like itarrow_forwardA real estate agency pays a sales representative monthly minimum income of 1000, 54 TL, additional allowance according to his/her title, plus 200 TL for each property sold by the sales representative in that month and a certain amount of the sales amount. Additional allowance amounts by title and bonus rates are given in the tables below: Title Additional Allowance (TL) Trainee (1) 250 Beginner (2) 350 Expert (3) Senior (4) 500 750 Sales Amount (TL) Bonus Rate (%) 0- 499.999,99 0.25 500.000- 999.999,99 0.5 1.000.000- 1.999.999,99 0.75 2.000.000-.. 1 By adhering the rules stated above, find the total salary amount of a sales representative for a particular month, by obtaining the title of a sales representative from the user (1 for the trainee, 2 for the novice, 3 for the specialist, 4 for the senior), the number of real estate sold this month and the total sales amount made by the sales representative. Write a C code that prints the total salary on the screen using the switch-case…arrow_forward
- As you review the recipe for chocolate chip cookies, you realize that the recipe is a(n) representative heuristic algorithm artificial rule availability heuristicarrow_forwardMatlabarrow_forwardCompany Dinner Lili works part-time in a restaurant near her campus as a waitress. One day, a boss from a company down the street decided to hold a company dinner in the restaurant she worked at. Before the dinner, the food is arranged in a sorted order A according to their type. Moments later, the manager of the restaurant confronted Lili and asks Q questions, each one asks how many foods of type Bi are there. Lili has asked you as her friend to help her count and answer as fast as possible. Format Input A single line with two integers N and Q denoting the number of food and the number of question respectively, followed by a line containing N elements denoting the type of the foods and another Q lines each containing a single integer denoting the number of occurrences of the type of food asked by the manager. Format Output Q lines each with a single integer denoting the number of occurence of Bi in A. Constraints • 1 ≤ N, Q ≤ 10^5 • 1 ≤ Ai, Bi ≤ 10^9 • Ai ≤ Aj for all 1 ≤ i ≤ j ≤ N…arrow_forward
- A rail transport company wishes to hold details of train services, stations and operators. Details include the following: Trains: A train has a unique id code, a collection of days it runs on (Monday, Sunday etc.), and the number of carriages it has. Each train must have at least one driver but can have many drivers that can drive it, and a driver may drive many trains. Each driver is identified by an employee number and a name. A train is designated as either an InterCity or District train though other types of train are possible. Each train must have an operator and not more than one operator. Each operator has a name and a phone number. The operator can operate many trains (or none at all!) Stations: A station has a name and the number of platforms it has. All stations are classified as either Main or District only. There are no other types of stations. Timetable: A train has a number of stops at a station during its journey. It never visits the same station twice on the same…arrow_forwardIf a child has red hair, they inherited a certain From their parentsarrow_forwardIn the US Higher Education sector, a degree is classified using a Grade Point Average (GPA). The grades ‘A’, ‘B’, ‘C’, ‘D’ or ‘F’ are called academic grades. Each ‘A’ is worth 4 points, each ‘B’ is worth 3 points, each ‘C’ is worth 2 points, each ‘D’ is worth 1 point and each ‘F’ is worth 0 points. The GPA is found by calculating the number of points and then dividing by the number of academic grades. A student may also have a non-academic grade of ‘W’ (for withdrew) which is not counted at all in the calculation. You can assume that the student will have at least one academic grade in their list of grades. There are many ways of calculating a GPA from a list of grades, but you must follow the algorithm given by this top-level decomposition: > Find GPA. >> Input a list of academic and non-academic grades. >> Create a new list that consists of the number of points for each academic grade in the input list. >> Add up the values in the new list and divide by the…arrow_forward
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- Enhanced Discovering Computers 2017 (Shelly Cashm...Computer ScienceISBN:9781305657458Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. CampbellPublisher:Cengage LearningA Guide to SQLComputer ScienceISBN:9781111527273Author:Philip J. PrattPublisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
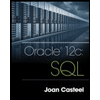
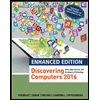
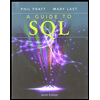
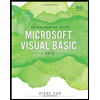