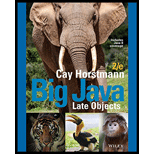
What is the value of each variable after the if statement?
- a. int n = 1; int k = 2; int r = n;
if (k < n) { r = k; }
- b. int n = 1; int k = 2; int r;
if (n < k) { r = k; }
else { r = k + n; }
- c. int n = 1; int k = 2;
int r = k; if (r < k) { n = r; }
else { k = n; }
- d. int n = 1; int k = 2; int r = 3;
if (r < n + k) { r = 2 * n; }
else { k = 2 * r; }

Explanation of Solution
a.
//Initialize the variables
int n=1; int k=2; int r=n;
//Check the condition
if (k<n)
{
//Assign k to r
r=k
}
Value of each variable after “if” statement:
n=1, k=2, r=1
Explanation:
The value assigned to variable “n” is “1”, the condition inside the “if” statement fails. As the value of “n” is not greater than “k” and so the value of “r” remains unchanged.

Explanation of Solution
b.
//Initialize the variables
int n=1; int k=2; int r;
//Check the condition
if (n<k)
{
//Assign k to r
r=k
}
//Otherwise
else
{
//Add k+n and store it in r
r=k+n;
}
Value of each variable after “if”statement:
n=1, k=2, r=2
Explanation:
The value assigned to variable “n” is “1”, the condition inside the “if” statement is true. As the value of “k” is greater than “n” and so the value of “r” is changed to “2”.

Explanation of Solution
c.
//Initialize the variables
int n=1; int k=2; int r=k;
//Check the condition
if (r<k)
{
//Assign k to r
n=r
}
//Otherwise
else
{
//Assign n to k
k=n;
}
Value of each variable after “if”statement:
n=1, k=1, r=2
Explanation:
The value assigned to variable “n” is “1”, the condition inside the “if” statement fails. As the value of “r” is not greater than “k” and it moves to the “else” part where the value of “k” is changed to value of “n” that is “1”. In the first statement, the value of “r” is initialized to value of “k” that is “2”.

Explanation of Solution
d.
//Initialize the variables
int n=1; int k=2; int r=3;
//Check the condition
if (r<n+k)
{
//Assign k to r
r=2*n
}
//Otherwise
else
{
//Assign n to k
k=2*r;
}
Value of each variable after “if”statement:
n=1, k=6, r=3
Explanation:
The value assigned to variable “n” is “1”, the condition inside the “if” statement fails. As the value of “r” is not greater than “n+k” and it moves to the “else” part where the value of “k” is changed to value of “2*r” that is “6”. In the first statement, the value of “r” is initialized to “3”.
Want to see more full solutions like this?
Chapter 3 Solutions
Big Java Late Objects
Additional Engineering Textbook Solutions
Starting Out with Java: Early Objects (6th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
C++ How to Program (10th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Programming in C
Starting Out With Visual Basic (8th Edition)
- Language: JAVA Leap-Year Write a program to find if a year is a leap year or not. We generally assume that if a year number is divisible by 4 it is a leap year. But it is not the only case. A year is a leap year if − It is evenly divisible by 100 If it is divisible by 100, then it should also be divisible by 400 Except this, all other years evenly divisible by 4 are leap years. So the leap year algorithm is, given the year number Y, Check if Y is divisible by 4 but not 100, DISPLAY "leap year" Check if Y is divisible by 400, DISPLAY "leap year" Otherwise, DISPLAY "not leap year" For this program you have to take the input, that is the year number from an input file input.txt that is provided to you. The input file contains multiple input year numbers. Use a while loop to input the year numbers from the input file one at a time and check if that year is a leap year or not. Create a class named LeapYear which will contain the main method and write all your code in the main…arrow_forwardint main(){ if(var1>50) { num = 20.0 } else { if(var1>35) ---- Line 7 { num= 35.0; } else if (var1<80) { num=60.0; } else { num=40.0; }} What should be the outcome of the condition in line 7 in order for the value of num to be equal to 60.0?arrow_forwardа. int GreaterThan(int no) { if (no > 10) printf("%d is greater than 10", no); else printf("%d is less than 10", no); }arrow_forward
- OOP JAVAarrow_forwardJava Lab 5 Problem Statement Write a program that quizzes the user on the names of the capital cities for all the states in the United States. Each state is presented to the user, and they must enter the name of the capital. The program then either tells the user they're correct or tells them the correct answer. The user's answer should not be case-sensitive. At the end, the program tells the user the total number they got correct. Here's an example: What is the capital of Alabama? MontogomeryThe correct answer is Montgomery.What is the capital of Alaska? juneauThat is the correct answer!...You got 32 out of 50 correct! The state capitals will be stored in a 2D String array of size 2 x 50, with the first column the name of the state and the second the name of the capital city, for all 50 states. To create the state capitals array, follow these instructions: Copy the String from the attached file into your program (found below these instructions). The String is a pipe delimited…arrow_forwardConvert totalMeters to hectometers, decameters, and meters, finding the maximum number of hectometers, then decameters, then meters. Ex: If the input is 815, then the output is: Hectometers: 8 Decameters: 1 Meters: 5 Note: A hectometer is 100 meters. A decameter is 10 meters. 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 15 16 17 18 int totalMeters; int numHectometers; int numDecameters; int numMeters; cin >>totalMeters; cout << "Hectometers: cout << "Decameters: cout << "Meters: " << numMeters << endl; " << numHectometers << endl; << numDecameters << endl; 2 3arrow_forward
- 8. Determine the value of variable num1, num2 and num3 at the end of the following code. int product(int A, int *B){ *B += 1; A *= *B; return A; } int main(){ int num1 = 2, num2 = 3, num3; num3 = product(num2, &num1); O A. num1 = 3, num2 = 3, num3 = 9 num1 = 2, num2 = 3, num3 = 9 num1 = 2, num2 = 3, num3 = 6 %3D D. num1 = 3, num2 = 2, num3 = 6 B.arrow_forwarddvvxarrow_forwardJAVA based program need helparrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
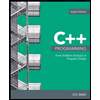
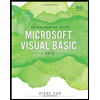