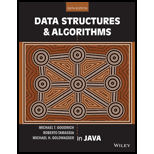
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 3, Problem 19C
Explanation of Solution
Methods add() and remove() for Scoreboard class without any loops:
Code for add() method:
//Define the add() method to add a new score to collection
public void add(GameEntry e)
{
/*Check whether the "numEntries" is less than length of board. */
if (numEntries < board.length)
{
//Assign the new entry to score board
board[numEntries] = e;
//Increment "numEntries" by "1"
numEntries++;
}
}
Explanation:
In the above code,
- This method accepts the input parameter of “e” to add a new score entry to collection of score board.
- Check whether the “numEntries” is less than length of board. If yes,
- Assign the new entry to score board.
- Increment “numEntries” by “1”.
Code for remove() method:
/*Define the remove() method to remove and return a high score from collection. */
public GameEntry remove(int i) throws IndexOutOfBoundsException
{
/*Check whether the "i" is less than "0" and "i" is greater than "numEntries"...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
write a java program (method) to store all arrangments(permuations) of a given string in an array.
note: Here it has to be mentioned that the permutations can also be of shorter length than the list of letters and that no letter is to be repeated. For example, if the initial list of letters is "STOP", then "TOP" and "TOPS" are both valid permutations, but "STOPS" isn't.
Use Java programming.
Write the removeEvens() method, which receives an array of integers as a parameter and returns a new array of integers containing only the odd numbers from the original array. The main program outputs values of the returned array.
Hint: If the original array has even numbers, then the new array will be smaller in length than the original array and should have no blank elements.
Ex: If the array passed to the removeEvens() method is [1, 2, 3, 4, 5, 6, 7, 8, 9], then the method returns and the program output is:
[1, 3, 5, 7, 9]
Ex: If the array passed to the removeEvens() method is [1, 9, 3], then the method returns and the program output is:
[1, 9, 3]
import java.util.Arrays;
public class LabProgram {
public static int[] removeEvens(int [] nums) {
/* Type your code here */}
public static void main(String[] args) {
int [] input = {1,2,3,4,5,6,7,8,9};int [] result = removeEvens(input);
// Helper method Arrays.toString() converts int[] to a…
You are asked to re-implement the insertion sort (InsertionSort.java), with additional requirements. Particularly:
1. For each iteration, how a number from a unsorted region is placed in the correction posion in the sorted region;
2. How to make the whole array be sorted based on the previous step, and count the total number of shifts during the whole insertion sort process.
To complete the whole implementation, you should write at least the following important methods:
Part1: insertLast
/**
A method to make an almost sorted array into fully sorted.
@param arr: an array of integers, with all the numbers are sorted excepted the last one
@param size: the number of elements in an array
*/
public static void insertLast(int[] arr, int size)
{
// your work
}
In this method, you are required to print the following information for each iteration until the termination of the loop:
•The intermediate array content and
•The comparing pair information
Part2: insertionSort
/**
A method to make an…
Chapter 3 Solutions
Data Structures and Algorithms in Java
Ch. 3 - Prob. 1RCh. 3 - Write a Java method that repeatedly selects and...Ch. 3 - Prob. 3RCh. 3 - The TicTacToe class of Code Fragments 3.9 and 3.10...Ch. 3 - Prob. 5RCh. 3 - Prob. 6RCh. 3 - Prob. 7RCh. 3 - Prob. 8RCh. 3 - Prob. 9RCh. 3 - Prob. 10R
Ch. 3 - Prob. 11RCh. 3 - Prob. 12RCh. 3 - Prob. 13RCh. 3 - Prob. 14RCh. 3 - Prob. 15RCh. 3 - Prob. 16RCh. 3 - Prob. 17CCh. 3 - Prob. 18CCh. 3 - Prob. 19CCh. 3 - Give examples of values for a and b in the...Ch. 3 - Suppose you are given an array, A, containing 100...Ch. 3 - Write a method, shuffle(A), that rearranges the...Ch. 3 - Suppose you are designing a multiplayer game that...Ch. 3 - Write a Java method that takes two...Ch. 3 - Prob. 25CCh. 3 - Prob. 26CCh. 3 - Prob. 27CCh. 3 - Prob. 28CCh. 3 - Prob. 29CCh. 3 - Prob. 30CCh. 3 - Prob. 31CCh. 3 - Prob. 32CCh. 3 - Prob. 33CCh. 3 - Prob. 34CCh. 3 - Prob. 35CCh. 3 - Write a Java program for a matrix class that can...Ch. 3 - Write a class that maintains the top ten scores...Ch. 3 - Prob. 38PCh. 3 - Write a program that can perform the Caesar cipher...Ch. 3 - Prob. 40PCh. 3 - Prob. 41PCh. 3 - Prob. 42PCh. 3 - Prob. 43P
Knowledge Booster
Similar questions
- run the main() method of class Lab11D. Pls do the SWAPIN LAB 11D and below is the tester For example, A B C D E F should turn into D E F A B C. You should assume that the array list has an even number of elements (not necessarily 6). One solution is to keep removing the element at index 0 and adding it to the back. Each step would look like this: A B C D E F B C D E F A C D E F A B D E F A B C When you run the code, you will find that there is a lot of movement in the array list. Each call to remove(0) causes n - 1 elements to move, where n is the length of the array. If n is 100, then you move 99 elements 50 times, (almost 5000 move operations). That's an inefficient way of swapping the first and second halves. (Learn more about time complexity at the end of the lab). Come up with a better way in which you swap the elements directly. Hint: How do you swap the two elements A and D? A B C D E F D B C A E F D E C A B F D E F A B C Write pseudocode for this algorithm. Add…arrow_forward: Imagine you are reading in a stream of integers. Periodically, you wish to be ableto look up the rank of a numberx (the number of values less than or equal to x). lmplementthe datastructures and algorithms to support these operations. That is, implement the method track ( intx), which is called when each number is generated, and the method getRankOfNumber(intx), which returns the number of values less than or equal to x (not including x itself).EXAMPLEStream (in order of appearance): 5, 1, 4, 4, 5, 9, 7, 13, 3getRankOfNumber(l) 0getRankOfNumber(3) = 1getRankOfNumber(4) =3arrow_forwardMake a recursive method for factoring an integer n. First, find a factor f, then recursively factor n / f. This assignment needs a resource class and a driver class; these two classes will need to be in two separate files. The resource class will contain all of the methods and the driver class only needs to call the methods. The driver class needs to have only 5 lines of code. The code needs to be written in Java.arrow_forward
- Write a java method that receive a reference to an array of integers and then prints only the prime numbers which their locations in the array are not contiguous(i.e. non-prime numbers must remain in their locations, and prime numbers which are not contiguous must be sorted ). Note: you can not define new arrays. (i.e. You have to do the sorting job in-place) Example: 4 7 5 6 8 3: 7 and 5 are contiguous which 5 and 3 are not contiguousarrow_forwardProvide a different implementation of ChoiceQuestion. Instead of storing the choices in an array list, the addChoice method should add the choice to the question text. For this purpose, an addLine method has been added to the Question class. Use the following files: Question.java /** A question with a text and an answer.*/public class Question{ private String text; private String answer; /** Constructs a question with empty text and empty answer. */ public Question() { text = ""; answer = ""; } /** Sets the answer for this question. @param correctResponse the answer */ public void setAnswer(String correctResponse) { answer = correctResponse; } /** Checks a given response for correctness. @param response the response to check @return true if the response was correct, false otherwise */ public boolean checkAnswer(String response) { return response.equals(answer); } /** Add a line of text to…arrow_forwardFor this exercise, you need to shift all the elements in the array one space to the right (increase the index of the element by 1). The last element will wrap around and become the new first element. For example, given: moveUp({"first", "second", "third", "fourth"}); You would return: {"fourth", "first", "second", "third"} in javaarrow_forward
- So how fast is the merge sort? Does it depend on the type of array? We saw with some of our previous sorts that it depended on the initial array. For example, an Insertion sort was much faster for a nearly sorted array. In this exercise, you are given the Sorter class, which contains a static mergeSort method that takes an int array and length as an input. You are also given the SortTester class which has three static methods for creating 3 different types of arrays. For this test, create one of the three arrays, then take a start time using System.currentTimeMillis(). Next, run the array through the mergeSort method in the Sorter class. Finally, record the end time and print out the results. Repeat this for the other two array types. 10.3.6: Merge Sort Benchmark Testingarrow_forwardSo how fast is the merge sort? Does it depend on the type of array? We saw with some of our previous sorts that it depended on the initial array. For example, an Insertion sort was much faster for a nearly sorted array. In this exercise, you are given the Sorter class, which contains a static mergeSort method that takes an int array and length as an input. You are also given the SortTester class which has three static methods for creating 3 different types of arrays. For this test, create one of the three arrays, then take a start time using System.currentTimeMillis(). Next, run the array through the mergeSort method in the Sorter class. Finally, record the end time and print out the results. Repeat this for the other two array types. Sample Output Random Array: ** Results Hidden ** Almost Sorted Array: ** Results Hidden ** Reverse Array: ** Results Hidden **arrow_forwardcan you please write it in java.util.scanner form and can you make it so i can copy and past it please thank you, The product of two matrices is a calculation between two matrices. When the inner dimensions are the same, meaning that the number of columns of the first matrix A is equal to the number of rows of the second matrix B, the product of these two matrices is defined as: C[i][j] = a[i][1] * b[1][j] + a[i][2] * b[2][j] … + a[i][n] * b [n][j] (If A is an m × n matrix and B is an n × p matrix) For details on the topic, check: https://www.mathsisfun.com/algebra/matrix-multiplying.html Write a program to perform this calculation. Provide two example input arrays and calculate the output array. Note that matrix can easily be implemented in Java using 2 dimensional array. However, in Java, 2D array’s elements start at row 0 and column 0, while in mathematical definition, matrix elements start at row 1 and column 1. Consider how you will handle this difference and…arrow_forward
- : Imagine you are reading in a stream of integers. Periodically, you wish to be able to look up the rank of a number x (the number of values less than or equal to x). Implement the data structures and algorithms to support these operations. That is, implement the method track(int x), which is called when each number is generated, and the method getRankOfNumber(int x), which returns the number of values less than or equal to x (not including x itself). EXAMPLE Stream (in order of appearance): 5, 1, 4, 4, 5, 9, 7, 13, 3 getRank Of Number (1) = 0 getRankOf Number (3) = 1 getRankOfNumber (4) = 3arrow_forwardWrite in Java. Given an array of integers A, find the Majority Element. Majority Element in an array of size N in an element that appears more than N/2 times. Write a function: int findMajority(int[] A) that accepts an array A. The function should return the Majority Element in the array. If no majority element then return 0. Use following methods to solve the problem: int findCandidate(int a[]) that accepts the array and find a candidate for the majority boolean isMajority(int a[], int cand) that accept the array and the candidate element and check if the candidate occurs more than n/2 times Input: 5 1 2 1 2 2 Where, First line represents the size of an array. Second line represents array elements separated by single space. Output: 2 Here for the given array, 2 appears 3 times in the array of size 5. No space after the element in the output. Assume that, N is an integer within the range [1 to 10000]. Array elements are within the range…arrow_forwardWrite a Java program to work with two arrays and perform operations on them. Your program should have 2 methods: int[] union (int[] al, int[] a2): takes two integer arrays and returns a new integer array consisting of all ints that appear in either a1 or a2. The returned array should be appropriately sized and should contain any duplicate ints since everything from both a1 and a2 should be in there. The returned array should not have any empty spots and should be sorted numerically. ● ● int[] intersection (int[] al, int[] a2): takes two integer arrays and returns a new integer array consisting of all ints that appear in both a1 and a2. The returned array should be appropriately sized and sorted numerically, and it should not contain any duplicate ints or empty spots. For example, when a1 = {1,2,3,4,5} and a2 {1,5,6,7,8}, union (al, a2) will return {1,1,2,3,4,5,5,6,7,8} and intersection (al, a2) will return {1,5}. = Provide the following menu using a do..while loop in the main method to…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
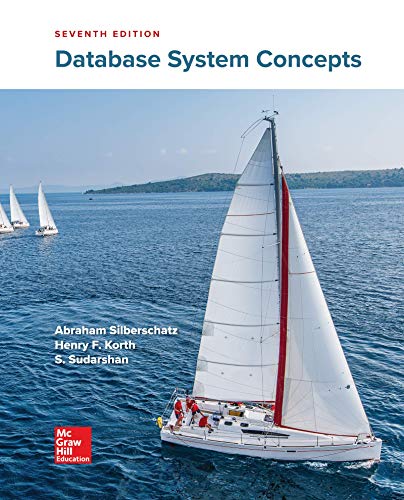
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
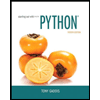
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
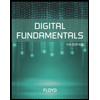
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
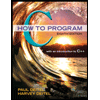
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
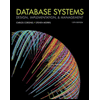
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
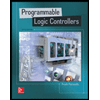
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education