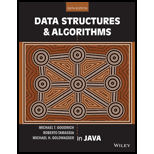
Explanation of Solution
Method equals() in CircularlyLinkedList class:
//Define the equals() method
public boolean equals(CircularlyLinkedList<E> other)
{
//Declare and initailze the Boolean variable
boolean result = false;
/*Call size() to find the first list size and assign it into "size1" variable. */
int size1 = size();
/*Call size() to find the second list size and assign it into "size2" variable. */
int size2 = other.size();
//Check whether the size of the two lists is equal
if(size1 != size2)
//Return false that is, two lists are not equal
return result;
/*Call equals() method recursively to check first element in two lists are equal and the result into "result" variable. */
result = head.equals(other.head);
//Check whether "result" is not equal to false
if (!result)
//Return true that is, two lists are equal
return result;
/*Assign the next of head from current list into "next" node. */
Node<E> next = head.getNext();
/*Assign the next of head from other list into "nextOfOther" node. */
Node<E> nextOfOther = other.head.getNext();
/*Loop executes until the size of current list is greater than 1. */
while (size1 > 1)
{
/*Call equals() method recursively to check all the elements in two lists are equal and assign the true if it is equal otherwise, false. */
result = next.equals(nextOfOther);
//Check whether "result" is not equal to true
if (!result)
// Break it, because, they aren't equal
break;
/*Assign the next of next from current list into "next" node...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- Import the ArrayList and List classes from the java.util package to create a list of phone numbers and also import the HashSet and Set classes from the java.util package to create a set of unique prefixes. Create a class called PhoneNumberPrefix with a main method that will contain the code to find the unique prefixes. Create a List called phoneNumbers and use the add method to add several phone numbers to the list. List<String> phoneNumbers = new ArrayList<>(); phoneNumbers.add("555-555-1234"); phoneNumbers.add("555-555-2345"); phoneNumbers.add("555-555-3456"); phoneNumbers.add("444-444-1234"); phoneNumbers.add("333-333-1234"); Create a Set called prefixes and use a for-each loop to iterate over the phoneNumbers list. For each phone number, we use the substring method to extract the first 7 characters, which represent the prefix, and add it to the prefixes set using the add method. Finally, use the println method to print the prefixes set, which will contain all of…arrow_forwardConsider Bag, SinglyLinkedList, and DoublyLinkedLists classes for integers. Implement and test the following methods: 1. Write a method in Bag class for integers that finds the number of odd and even numbers in the list. 5. Write a method in DoublyLinkedList class that swaps the first and last nodes in the list. 6. Write a Main class to test all these methods.arrow_forwardWrite a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forward
- Add a method swapTwoNodes to SinglyLinkedList class from week 2 lecture examples. This method should swap two nodes node1 and node2 (and not just their contents) given references only to node1 and node2. The new method should check if node1 and node2 are the same node, etc. Write the main method to test the swapTwoNodes method. Hint: You may need to traverse the list. Please give me the full code and share how it works thank you! I need java code, not C ++ or C#.arrow_forwardWrite a method for the Linked Based List class which returns the largest item in the list. If the list is empty return null. Assume that class T is Comparable.DO NOT USE ANY OTHER METHODS OF THE LINKED BASED LIST CLASS. What is the worst case and best case complexity of the code in the previous problem? Explain your answer.arrow_forwardYou may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as: 1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index() Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. 4. delete_at_start() 5. delete_at_end() . 6. display() the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display(). hint on how to start thee code # Initialize the Node class Node: def __init__(self, data): self.item = data…arrow_forward
- Trying this again since part of my question keeps disappearing Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts public class ItemNode { private String item; private ItemNode nextNodeRef; // Reference to the next node public ItemNode() { item = ""; nextNodeRef = null; } // Constructor public ItemNode(String itemInit) { this.item = itemInit; this.nextNodeRef = null; } // Constructor…arrow_forward1. According to the following LinkedList, write pseudo code for the question belowHow do you insert a node with the data, "Brandon" between the node of "Chu" and the node of "Bethany"? 2.Assume that there is a linked list that connected several names.How do you set up a "while" loop to print the whole list? 3.What is the advantage of using a LinkedList over an ArrayList?arrow_forwardImplement a member method for the List ADT called removeDuplicates(). The method removes every duplicate from the list such that there is only ONE copy of each element in the list. The method also returns the number of copies it removed. For example, if L = {Joe, Bob, Joe, Ned, Bob, Ron, Ron}, a call to L.removeDuplicates modifies the list such that L = {Joe, Bob, Ned, Ron} and returns 3. import java.util.Iterator;import java.util.NoSuchElementException; public class Mock1ListWrapper { public static interface List<E> extends Iterable<E> { public int size(); public boolean isEmpty(); public boolean isMember(E e); public int firstIndexOf(E e); public int lastIndexOf(E e); public void add(E e); public void add(E e, int position); public E get(int position); public E remove(int position); public E replace(int position, E newElement); public void clear(); public Object[] toArray(); public int removeDuplicates(); } @SuppressWarnings("unchecked") public static…arrow_forward
- File TesSLL.java contains a driver that allows you to experiment with these methods. Compile and run TestSLL, and play around with it to see how it works. Then add the following methods to the SinglyLinkedList class. For each, add an option to the driver to test it. Do this in incremental steps!! This means, implement and test the methods one at a time.arrow_forwardThe curly bracket used in java to enclose units of code can not be used in a fill in questions. For this questions the curly brackets will be substituted with comments. Interpret the begin and end comments as curly brackets. Complete the code for the method filter. The method receives two parameter of generic type E called low and high. The method filters the elements within the linked list and return a linked list with all the elements from the low value to the high value. Example: [1,2,4,5,8,9,11,12] Called with low = 2 and high =10 will return [2,4,5,8,91. public //begin method MyLinkedList newlist = 0; Node befPtrD if(this. ==null) return newlist; for (Node clptr= //begin for if && //begin if newlist.append(clptr.element); if( head=head.next; else if(clptr==tail) +ail-bofPtrarrow_forwardSuppose that you want an operation for the ADT list that adds an array of items to the end of the list. The header of the method could be as follows: public void addAll( T[ ] items) Write an implementation of this method for the class LinearLinkedListarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
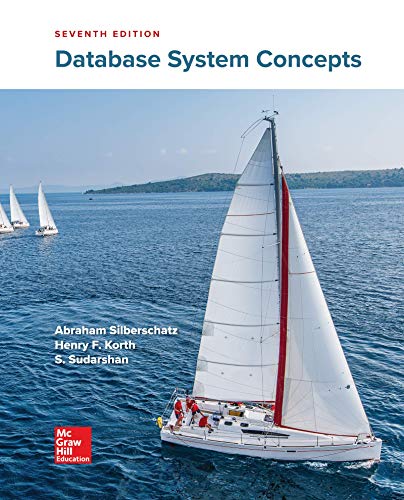
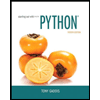
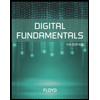
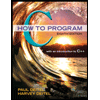
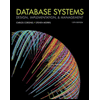
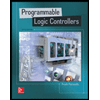