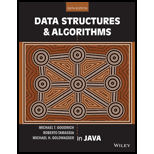
Explanation of Solution
The algorithm to swap two nodes “x” and “y” in singly linked list “L” is given below:
Algorithm:
Input: Two nodes “x” and “y”.
Output: Swap the two nodes in singly linked list “L”.
swapSingly(x, y):
//Create a node to assign the head into it
Node n = head;
/*Loop executes until the next node of head is not equal to "x" node. */
while(n.getNext() != x)
/*Get next node of head and assign it into "n" node. */
n = n.getNext();
/*Get the next node of "y" node and assign it into "v" node. */
Node v = y.getNext();
/*Call setNext() method to set the next node as "y" node by using "n".*/
n.setNext(y);
/*Call setNext() method to set the next node as "x" node by using "y". */
y.setNext(x);
/*Call setNext() method to set the next node as "v" node by using "x". */
x.setNext(v);
Explanation:
In the above algorithm,
- This method accepts two input parameters such as “x” and “y”.
- Create new node “n” and “v” to hold nodes “x” and “y” from singly linked lists.
- The while loop executes until the next node of head is not equal to “x” node.
- Get next node of head and assign it into “n” node.
- Get the next node of “y” node and assign it into “v” node.
- Call setNext() method to set the next node as “y” node by using “n”.
- Call setNext() method to set the next node as “x” node by using “y”.
- Call setNext() method to set the next node as “v” node by using “x”.
- Finally, the two nodes “x” and “y” are swapped in singly linked list.
Algorithm to swap two nodes in doubly linked list:
The algorithm to swap two nodes “x” and “y” in singly linked list “L” is given below:
Algorithm:
Input: Two nodes “x” and “y”.
Output: Swap the two nodes in doubly linked list “L”.
swapDoubly(x, y):
/*Create a node to assign the previous node of "x" node into it...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- Given a singly linked list of integers, reverse the nodes of the linked list 'k' at a time and return its modified list. 'k' is a positive integer and is less than or equal to the length of the linked list. If the number of nodes is not a multiple of 'k,' then left-out nodes, in the end, should be reversed as well.Example :Given this linked list: 1 -> 2 -> 3 -> 4 -> 5 For k = 2, you should return: 2 -> 1 -> 4 -> 3 -> 5 For k = 3, you should return: 3 -> 2 -> 1 -> 5 -> 4 Note :No need to print the list, it has already been taken care. Only return the new head to the list. Input format :The first line contains an Integer 't' which denotes the number of test cases or queries to be run. Then the test cases follow. The first line of each test case or query contains the elements of the singly linked list separated by a single space. The second line of input contains a single integer depicting the value of 'k'. Remember/Consider :While specifying the list…arrow_forwardGiven a linked list consists of data, a next pointer and also a random pointerwhich points to a random node of the list. Give an algorithm for cloning the list.?arrow_forwardNote: Your solution should have O(n) time complexity, where n is the number of elements in l, and O(1) additional space complexity, since this is what you would be asked to accomplish in an interview. Given a linked list l, reverse its nodes k at a time and return the modified list. k is a positive integer that is less than or equal to the length of l. If the number of nodes in the linked list is not a multiple of k, then the nodes that are left out at the end should remain as-is. You may not alter the values in the nodes - only the nodes themselves can be changed.arrow_forward
- A linked list has a cycle such that if you start at any node p and follow a sufficient number of subsequent links, you will end up at node p. Not that p does not have to be the list's initial node. Assume you are given an N-node linked list. The value of N, on the other hand, remains unknown. 1. Create an O(N) algorithm to check if a list includes a cycle. You may add O(N) more spaces. 2. Rep the previous question, this time using just O(1) more space. (Hint: Use two pointers that start at the beginning of the list but progress at separate rates.)arrow_forwardA linked list contains a cycle if, starting from some node p , following a sufficient number of next links brings us back to node p . p does not have to be the first node in the list. Assume that you are given a linked list that contains N nodes. However, the value of N is unknown. Design an O (N ) algorithm to determine if the list contains a cycle. You may use O (N ) extra space. Repeat part (a), but use only O (1) extra space. (Hint: Use two iterators that are initially at the start of the list, but advance at different speeds.)arrow_forwardWrite a function to count the number of nodes in a singly linked list. Discuss the approach and complexity of your solution.arrow_forward
- there are similar questions please come up with an original answer because the questions are slighty different, thank you. FREE = 2 B FIRST = 5 D SHOW CHANGES IN DATA AND NEXT MEMBERS OF THE ARRAY AND THE VALUES OF FIRST AND FREE AFTER H IS INSERTED INTO THE LINKED LIST SO THE RESULTING LIST IS IN ALPHABETICAL ORDER.arrow_forwardCreate two singly linked lists A and B to represents elements in the following set A and B respectively. 3. A = { 5, 6, 8, 9, 10, 11} B = { 1, 6, 2, 9, 3, 11, 4} Develop an algorithm and implement the same to represent only intersection of two lists A and B and print the same.arrow_forwardGiven two lists sorted in increasing order, create and return a merged list of all the elements in sorted order. You may modify the passed in lists. Ideally, the solution should work in "linear" time, making a single pass through each list.arrow_forward
- 1. How many ways can I make a list of length n out of n elements if I allow repeats? (For example, if n=2, if there are no repeats my possible lists are 12 and 21. But if I allow repeats the possible lists are 11, 12, 21, and 22.arrow_forwardImplement a self-organizing list by building a single linked list of nodes and frequently and randomly retrieving data from the list. Show what happens to the list when the count, move to front, and transpose techniques are applied to the same set of frequently retrieved items.arrow_forwardcan I have the solution for this question in Java coding please? Write an algorithm for the following to swap two adjacent elements by adjusting only the links (and not the data) using: Singly-linked lists. Doubly linked lists.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
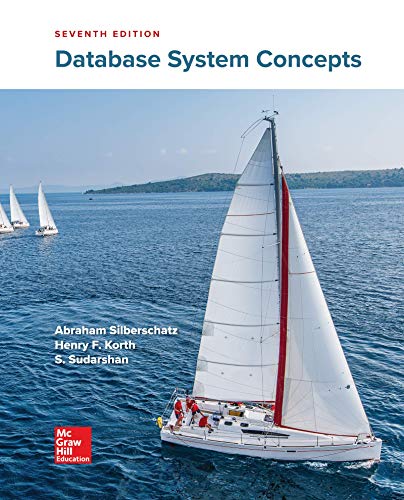
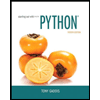
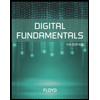
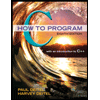
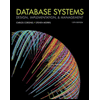
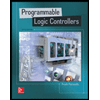