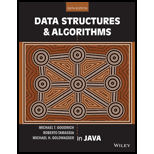
Explanation of Solution
Consequences when delete the two lines from code:
Note: Refer Code Fragment 3.15 “SinglyLinkedList class definition” in the Text book.
The SinglyLinkedList class contains number of methods such as “size()”, “first()”, “last()”, “addFirst()”, “addLast()”, “removeLast()”, and “removeFirst()”.
In removeFirst() method,
- Remove the line number “51” and “52” in this method.
- That is, resetting the “tail” field to “null”.
Modified:
In the above code, just remove or delete the Line “51” and “52”. Then the code is given below:
//Method definition
public E removeFirst( ) //Line 46
{
//Check whether the linked list is empty
if (isEmpty( )) //Line 47
//Return null, there is no element to remove
return null;
/*Get the head node from linked list and assigned it. */
E answer = head.getElement( ); //Line 48
//Assign the head node as next node of head
head = head...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- Design and implement a getLastHalf() method for the MyLinkedList class. The method should return the last half of the list as a new list leaving the original list intact. If the list has an uneven number of elements the extra one element must be in the list returned. Example : [1,3,5,4] return [5,4] and [1,6,9] return [6,9]. Follow the three step method for designing the method Develop the java code for the method Create a test program to test the method thoroughly using the Integer wrapper class. public class MyLinkedList<E> { private Node<E> head, tail; public MyLinkedList() { head = null; tail = null; } /** Return the head element in the list */ public E getFirst() { if (head == null) { return null; } else { return head.element; } } /** Return the last element in the list */ public E getLast() { if (head==null) { return null; } else { return tail.element; } } /** Add an element to the beginning of the list */ public void prepend(E e) { Node<E> newNode = new…arrow_forwardDesign and implement a getLastHalf() method for the MyLinkedList class. The method should return the last half of the list as a new list leaving the original list intact. If the list has an uneven number of elements the extra one element must be in the list returned. Example : [1,3,5,4] return [5,4] and [1,6,9] return [6,9]. Follow the three step method for designing the method Develop the java code for the method Create a test program to test the method thoroughly using the Integer wrapper class.arrow_forwardDesign and implement an insertSorted() method for the MyArrayList class. The method should receive a single object and insert it in its correct position. You can assume that the calling array is already in a sorted order. The calling array is therefore updated. Follow the three step method for designing the method. Develop the java code for the method. Create a test program to test the method thoroughly using the Integer wrapper class.arrow_forward
- You may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as: 1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index() Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. 4. delete_at_start() 5. delete_at_end() . 6. display() the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display(). hint on how to start thee code # Initialize the Node class Node: def __init__(self, data): self.item = data…arrow_forwardWrite a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forwardGiven the linked list data structure, implement a sub-class TSortedList that makes sure that elements are inserted and maintained in an ascending order in the list. So, given the input sequence {1,7,3,11,5}, when printing the list after inserting the last element it should print like 1, 3, 5, 7, 11. Note that with inheritance, you have to only care about the insertion situation as deletion should still be handled by the parent class.arrow_forward
- Write a method for the Linked Based List class which returns the largest item in the list. If the list is empty return null. Assume that class T is Comparable.DO NOT USE ANY OTHER METHODS OF THE LINKED BASED LIST CLASS. What is the worst case and best case complexity of the code in the previous problem? Explain your answer.arrow_forwardImport the ArrayList and List classes from the java.util package to create a list of phone numbers and also import the HashSet and Set classes from the java.util package to create a set of unique prefixes. Create a class called PhoneNumberPrefix with a main method that will contain the code to find the unique prefixes. Create a List called phoneNumbers and use the add method to add several phone numbers to the list. List<String> phoneNumbers = new ArrayList<>(); phoneNumbers.add("555-555-1234"); phoneNumbers.add("555-555-2345"); phoneNumbers.add("555-555-3456"); phoneNumbers.add("444-444-1234"); phoneNumbers.add("333-333-1234"); Create a Set called prefixes and use a for-each loop to iterate over the phoneNumbers list. For each phone number, we use the substring method to extract the first 7 characters, which represent the prefix, and add it to the prefixes set using the add method. Finally, use the println method to print the prefixes set, which will contain all of…arrow_forwardImplement the insert function in SLList class to insert an item x at a particular position in the list indexed by i. For example, let L= [1,2,3]. A call toL.insert (4,1) will make L=[1,4,2,3]. You should not change the item of existing nodes. Below is a skeleton of the Node and SLList class: template class Node public: ItemType item; Node *next; Node (ItemIype i, Node *n = nullptr) { item - i; next = n; } ; template class SLList { private: /** Pointer pointing to the sentinel node. */ Node *sentinel; /** Stores the current size of the list. */ int count; public: // Other functions omitted... void insert (const Item &x, int i) { // Copy this function in the answer and write code below this line. } };arrow_forward
- 4 O T O I D06 It is time for you to demonstrate your skills in a project of your own choice. You must DESIGN, ANALYSE AND CODE any method for the GENERIC MyLinkedList class that will manipulate the linked list. You can decide yourself what it should be following the specification below: 1. Purpose: The method must make logical sense - it should be of some purpose to somebody. You should describe in the text who will use the method for which purpose. 2. Clearly explain the problem. Then clearly explain how your method will solve it. 3. Test program: Test the method using a wranned class like Integer l Filters Add a caption. > Status (Contacts)arrow_forwardThere’s a somewhat imperfect analogy between a linked list and a railroad train, where individual cars represent links. Imagine how you would carry out various linked list operations, such as those implemented by the member functions insertFirst(), removeFirst(), and remove(int key) from the LinkList class in this hour. Also implement an insertAfter() function. You’ll need some sidings and switches. You can use a model train set if you have one. Otherwise, try drawing tracks on a piece of paper and using business cards for train cars.arrow_forwardWrite a Java class myLinkedList to simulate a singly linked list using arrays as the underlying structure. Include the following methods: 1. insert an element within the linked list.(this should also work for the front and the rear of the list) 2. Remove an element from the linked list 3. Display (print) the elements of the linked list in order. 4. A method to check if the list is "empty". Test your solution using a linked list that initially has the characters A, B, D, E, F, and G. Insert "C" between B and D. Remove element "F". [Hint: One solution (recommended) is to use 2 arrays. One for the data, and the other for the "next" pointer. Also, consider using dummy nodes for the front, and possibly the rear of the list] Extend the solution so that it simulates a doubly linked list! (hint: You will need a third array to represent the "backward" pointers)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
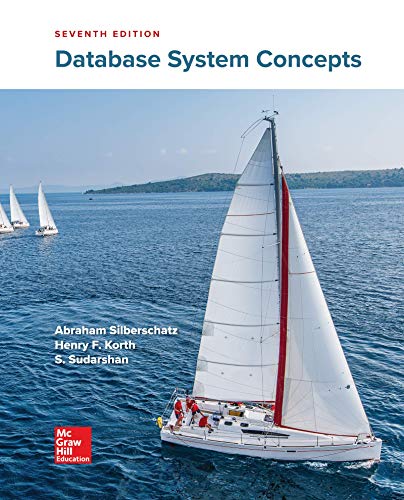
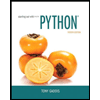
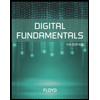
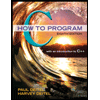
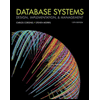
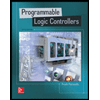