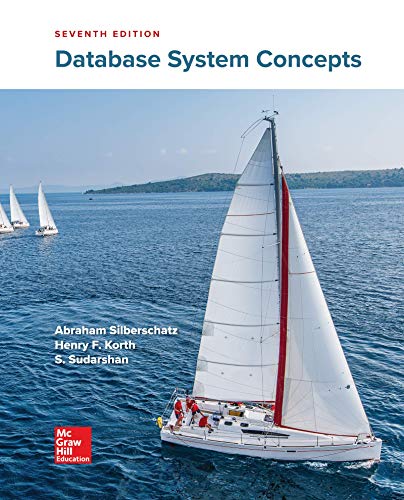
So how fast is the merge sort? Does it depend on the type of array? We saw with some of our previous sorts that it depended on the initial array. For example, an Insertion sort was much faster for a nearly sorted array.
In this exercise, you are given the Sorter class, which contains a static mergeSort method that takes an int array and length as an input. You are also given the SortTester class which has three static methods for creating 3 different types of arrays.
For this test, create one of the three arrays, then take a start time using System.currentTimeMillis(). Next, run the array through the mergeSort method in the Sorter class. Finally, record the end time and print out the results.
Repeat this for the other two array types.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- What is the process to create an explicit bigO calculator for selection sort, insertion sort merge sort, and quicksort? Java. AP Computer Science A. One class. There is supposed to be four original lists, which is a random list of numbers from 1-10 with 10 items, these lists will be used by the four sorts. There should be the sorted list for those original lists from each sort method. There should be an amount of comparisons for each sort. Also there should be bigO information, the best case, average case, worst case, the nlog2n, the n^2, sorting 10 lists from the sort methods of n increasing. Thank you. What the output can look like:arrow_forward// Only few methods are mentioned here //Do remaining methods to pass the test methods /*** Removes the specified (num) of the given element from the internal ArrayList of elements. * If num <= 0, don't remove anything. * If num is too large, remove all instances of the given element from the internal ArrayList of elements.* Example:* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 1, 2, 1* - Calling remove(2, 1) would remove the first 2 instances of 1 * - The CustomIntegerArrayList will then contain the integers: 2, 2, 1* - For a defined CustomIntegerArrayList containing the integers: 100, 100, 100 * - Calling remove(4, 100) would remove all instances of 100 * - The CustomIntegerArrayList will then be empty* - For a defined CustomIntegerArrayList containing the integers: 5, 5, 5, 5, 5 * - Calling remove(0, 5) would remove nothing* * @param num number of instances of element to remove * @param element to remove*/ public void remove(int num, int element) {//…arrow_forwardI need to have a main method in a Tester class that will: Fill an array of type Vehicle[] of size NUM_VEHICLES with Vehicle objects. You need to generate a random number between 0 and 2. If the number is 0, add a Vehicle to the array. 1, add a Car, 2, add a Boat. The Vehicle/Car/Boat that you add must be initialized with a random efficiency between 0 and 100.0. Do this until you have added NUM_VEHICLES objects to the array How do I write it so that the array generates random numbers 0 through 2 (inclusive) and how would I then correlate those numbers to a different class object?arrow_forward
- public class PokerAnalysis implements PokerAnalyzer { privateList<Card>cards; privateint[]rankCounts; privateint[]suitCounts; /** * The constructor has been partially implemented for you. cards is the * ArrayList where you'll be adding all the cards you're given. In addition, * there are two arrays. You don't necessarily need to use them, but using them * will be extremely helpful. * * The rankCounts array is of the same length as the number of Ranks. At * position i of the array, keep a count of the number of cards whose * rank.ordinal() equals i. Repeat the same with Suits for suitCounts. For * example, if your Cards are (Clubs 4, Clubs 10, Spades 2), your suitCounts * array would be {2, 0, 0, 1}. * * @param cards * the list of cards to be added */ publicPokerAnalysis(List<Card>cards){ this.cards=newArrayList<Card>(); this.rankCounts=newint[Rank.values().length]; this.suitCounts=newint[Suit.values().length];…arrow_forwardSo how fast is the merge sort? Does it depend on the type of array? We saw with some of our previous sorts that it depended on the initial array. For example, an Insertion sort was much faster for a nearly sorted array. In this exercise, you are given the Sorter class, which contains a static mergeSort method that takes an int array and length as an input. You are also given the SortTester class which has three static methods for creating 3 different types of arrays. For this test, create one of the three arrays, then take a start time using System.currentTimeMillis(). Next, run the array through the mergeSort method in the Sorter class. Finally, record the end time and print out the results. Repeat this for the other two array types. Sample Output Random Array: ** Results Hidden ** Almost Sorted Array: ** Results Hidden ** Reverse Array: ** Results Hidden **arrow_forwardHow do you do this? JAVAarrow_forward
- Devise an algorithm that detects whether a given array is sorted into ascending order. Write a Java method thatimplements your algorithm. You can use your method to test whether a sort method has executed correctly. Ensure that your program has a test classarrow_forwardIn writing a general-purpose sort method in Java, the sort method needs to compareobjects. There are two ways to give the sort method the code to compare objects,implementing the interfaces Comparable and Comparator respectively. Extend Article class toimplement Comparable interface so that the default sorting of the Articles is by its volumearrow_forwardCan you help me with this question?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
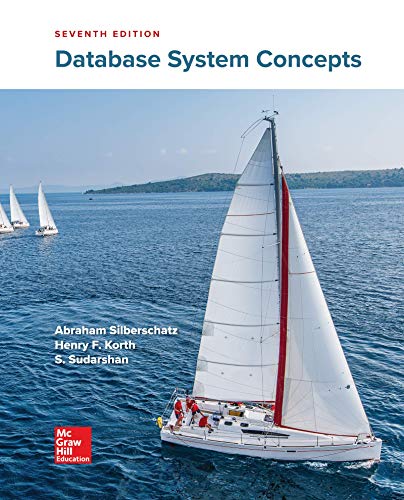
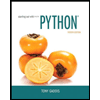
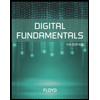
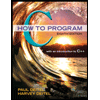
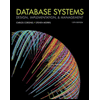
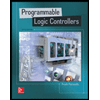