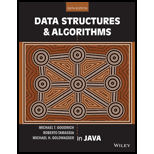
Explanation of Solution
Reimplementation of DoublyLinkedList class using only one sentinel node:
The doubly linked list is a linked data structure which contains the collection of sequentially data with two sentinel nodes such as “header” and “trailer”.
- If there is only one sentinel node, then the list is circularly linked using sentinel node.
- That is, header is sentinel node means, the next of the header is first node of list and previous node of header is last node of list.
Code for DoublyLinkedList class using only one sentinel node:
//Define the DoublyLinkedList class
public class DoublyLinkedList<E>
{
//Declare only one sentinel node
private Node<E> header;
//Declare and initialize the "size" variable
private int size = 0;
/*Define the Constructor to construct a new empty list. */
public DoublyLinkedList()
{
//Create header sentinel
header = new Node<>(null, null, null);
}
//Define the size() method
public int size()
{
/*Returns the number of elements in the linked list. */
return size;
}
//Define isEmpty() method
public boolean isEmpty()
{
//Check whether the linked list is empty
return size == 0;
}
//Define first() method
public E first()
{
//Check whether the list is empty
if(isEmpty())
//Return null
return null;
//Returns the first element of the list
return header.getNext().getElement();
}
//Define last() method
public E last( )
{
//Check whether the list is empty
if(isEmpty())
//Return null
return null;
/*Returns the last element of the list using the single sentinel node "header". */
return header.getPrev().getElement( );
}
//Define addFirst() method
public void addFirst(E e)
{
/*Call addBetween() method to adds element "e" to the front of the list. */
addBetween(e, header, header.getNext
}
/Define the addLast() method
public void addLast(E e)
{
/*Call addBetween() method to adds element e to the end of the list using single sentinel node "header". */
addBetween(e, header.getPrev(), header);
}
//Define the removeFirst() method
public E removeFirst()
{
//Check whether the list is empty
if (isEmpty())
//Return null
return null;
/*Call remove() method to removes and returns the first element of the list. */
return remove(header...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- Create an implementation of a doubly linked DoubleOrderedList class. You will need to create a DoubleNode class, a DoubleList class, and a DoubleIterator classarrow_forwardImplement class “LinkedList” which has two private data members head: A pointer to the Node class length: length of the linked list // Node* GetNode(int index) const;A private function which is only accessible to the class methods. This function takes an array-like index and returns the address of the node at that index. For example, index 0 corresponds to the head and index length-1 corresponds to end node of the linked list. The function returns NULL if the index is out of bound.// Implement the following public method: 1. bool RemoveHead();// Use RemoveAt FunctionRemove first node. Return true if successful, otherwise, false.2. bool RemoveEnd();// Use RemoveAt FunctionRemove last node. Return true if successful, otherwise, false.3 . void DisplayList() const;Display data of the whole linked list this in c++.arrow_forwardCreate an implementation of singly linked list using classes with minimum 5 nodes (spectre, togusa, yuri, siren, shepherd) in Python with the following capabilities/functions: Traverse - print out all data from the linked list Insert - generate a node and attach to an existing linked list Search - find an item (data) from the linked list and return the node Remove - remove a node from the linked listarrow_forward
- Implement a class for Circular Doubly Linked List (with a dummy header node) which stores integers in unsorted order. Your class definitions should look like as shown below:class CDLinkedList;class DNode {friend class CDLinkedList;private int data;private DNode next;private DNode prev;};class CDLinkedList {private:DNode head; // Dummy header nodepublic CDLinkedList(); // Default constructorpublic bool insert (int val); //Inserts val at end into the linked list.Time complexity:O(1)public bool removeSecondLastValue ();//Note: Remove the Second last val from the linked list. Time complexity: O(1)public void findMiddleValue(); // find middle value of the linklist and delete it.public void display(); // Displays the contents of linked list on screen …};arrow_forwardImplement a class for Circular Doubly Linked List (with a dummy header node) which stores integers in unsorted order. Your class definitions should look like as shown below:class CDLinkedList;class DNode {friend class CDLinkedList;private int data;private DNode next;private DNode prev;};class CDLinkedList {private:DNode head; // Dummy header nodepublic CDLinkedList(); // Default constructorpublic bool insert (int val); //Inserts val at end into the linked list.Time complexity:O(1)public bool removeSecondLastValue ();//Note: Remove the Second last val fromarrow_forwardWrite a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forward
- Create a lazy elimination deletion function for the AVLTree class.There are several approaches you can take, but one of the simplest is to add a Boolean property to the Node class that indicates whether or not the node is designated for deletion. This variable must then be considered by your other techniques.arrow_forwardConsider the implementation of the Ordered Linked list class, implement the following functions as part of the class definition: ▪ index(item) returns the position of item in the list. It needs the item and returns the index. Assume the item is in the list. ▪ pop() removes and returns the last item in the list. It needs nothing and returns an item. Assume the list has at least one item. ▪ pop_pos(pos) removes and returns the item at position pos. It needs the position and returns the item. Assume the item is in the list. ▪ a function that counts the number of times an item occurs in the linked list ▪ a function that would delete the replicate items in the linked list (i.e. leave one occurrence only of each item in the linked list) Your main function should do the following: ▪ Generate 15 random integer numbers in the range from 1 to 5.▪ Insert each number (Item in a node) in the appropriate position in a linked list, so you will have a sorted linked list in ascending order.▪…arrow_forwardwrite a code in modules and also with main class to implement the operation on linked list.. (searching , traversing , updating , and inserting.) insertion will be In empty list, In front of list, In the middle of list, at the end of list. The code should be in java language and also with simple coding and explanation.arrow_forward
- Implement the insert function in SLList class to insert an item x at a particular position in the list indexed by i. For example, let L= [1,2,3]. A call toL.insert (4,1) will make L=[1,4,2,3]. You should not change the item of existing nodes. Below is a skeleton of the Node and SLList class: template class Node public: ItemType item; Node *next; Node (ItemIype i, Node *n = nullptr) { item - i; next = n; } ; template class SLList { private: /** Pointer pointing to the sentinel node. */ Node *sentinel; /** Stores the current size of the list. */ int count; public: // Other functions omitted... void insert (const Item &x, int i) { // Copy this function in the answer and write code below this line. } };arrow_forwardA linked list cannot be used to represent a collection. This is a set data structure that doesn't have any values in it whatsoever. There are no resemblances between sets. Mathematics set functions like union and intersection are applicable to sets.arrow_forwardImplementing a Double-Ended List:contains the firstLastList.cpp program, which demonstrates a doubleended list. (Incidentally, don’t confuse the double-ended list with the doubly linked list, which we’ll explore later in Hour 10, “Specialized Lists.”)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
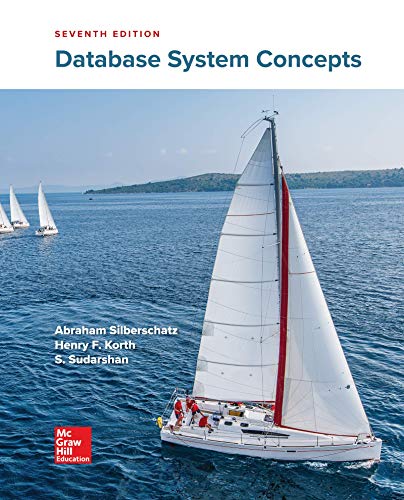
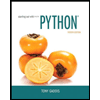
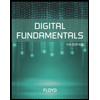
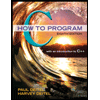
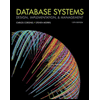
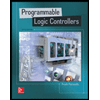