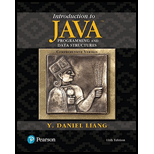
Concept explainers
Test whether a graph is connected
Program Plan:
Exercise.java:
- Import the required packages.
- Create a class “Exercise”:
- Define the main method
- Prompt user to enter the link.
- Get the input
- The input is validated to read the number of vertices present in the graph.
- Display the count of the vertices.
- New array list gets defined.
- Loop that iterates to validate the input and remove the tokens.
- The graph values are validating by performing a depth first search.
- Condition to validate the vertices of the graph.
- Display the graph is connected or not connected.
- Define the main method
UnweightedGraph.java:
- Import the required packages.
- Create a class “UnweightedGraph”:
- New list for the vertices gets created.
- New list for the neighbor node gets created.
- Create an empty constructor.
- Method to create new graph gets created and adjacency list gets created.
- Method to create an adjacency list gets created.
- Method to return the size of the vertices.
- Method to return the index of the vertices gets defined.
- Method to gets the neighbor node gets defined.
- Method to return the degree of the vertices gets created.
- Method to print the Edges gets created.
- New to clear the graph gets created.
- Method to add vertex gets created.
- Method to add edge gets created.
- Method to perform the depth first search gets defined.
- Method to perform breadth first search gets defined.
- Search tree gets returned.
- Create a class “SearchTree”,
- Define the method to return the root.
- Method to return the parent of the vertices
- Method to return the search order gets defined.
- Method to return the number of vertices found gets defined.
- Method to get the path of the vertices gets defined.
- Loop to validate the path gets defined.
- Path gets returned.
- Method to print the path gets defined.
- Method to print the tree gets defined.
- Display the edge.
- Display the root.
- Condition to validate the parent node to display the vertices gets created.
Graph.java:
- A graph interface gets created.
- Method to return the size gets defined.
- Method to return the vertices gets defined.
- Method to return the index gets created.
- Method to get the neighbor node gets created.
- Method to get the degree gets created.
- Method to print the edges.
- Method to clear the node gets created.
- Method to add the edges, add vertex gets created.
- Method to remove the vertices gets defined.
- Method for the depth first search gets defined.
- Method for the breadth first search gets defined.
Edge.java
- Create a class “Edge”,
- Define and declare the required variables.
- Constructor gets defined.
- Method that defines Boolean objects gets defined.
- Return the value after validating the vertices.

The below program is used to test whether the graph given is connected or not
Explanation of Solution
Program:
Exercise.java:
//import the required headers
import java.util.*;
//define the class exercise
public class Exercise
{
//main method
public static void main(String[] args) throws Exception
{
//scanner input gets defined
java.util.Scanner input = new java.util.Scanner(System.in);
//prompt user to enter the link
System.out.print("Enter a URL: ");
//get the link
java.net.URL url = new java.net.URL(input.nextLine());
//method to open the url
java.util.Scanner inFile = new java.util.Scanner(url.openStream());
//number of vertices are read
String s = inFile.nextLine();
//get the number of vertices
int ver_num = Integer.parseInt(s);
//display the count of vertices
System.out.println("The number of vertices is "
+ ver_num);
//new array list gets defined
java.util.List<Edge> list = new java.util.ArrayList<>();
//loop that validate the file
while (inFile.hasNext())
{
//read the file
s = inFile.nextLine();
//key words are read
String[] tokens = s.split("[\\s+]");
//read the starting vertex
int startingVertex = Integer.parseInt(tokens[0].trim());
/*loop that iterates for the entire length of the file*/
for (int i = 1; i < tokens.length; i++)
{
//remove the tokens
int adjacentVertex = Integer.parseInt(tokens[i].trim());
//add integers to the list
list.add(new Edge(startingVertex, adjacentVertex));
}
}
//new graph gets defined
Graph<Integer> mygraph = new UnweightedGraph<>(list, ver_num);
//display the edges
mygraph.printEdges();
//perform depth first serach
UnweightedGraph<Integer>.SearchTree mytree = mygraph.dfs(0);
//condition to validate the vertices
if (mytree.getNumberOfVerticesFound() == ver_num)
//display the graph is connected
System.out.println("The graph is connected");
else
//display the graph is not connected
System.out.println("The graph is not connected");
}
}
UnweightedGraph.java: Refer Listing 28.4 in the textbook.
Graph.java: refer Listing 28.3 in the textbook.
Edge.java: refer Listing 28.1 in the textbook.
Enter a URL:
http://liveexample.pearsoncmg.com/test/GraphSample1.txt
The number of vertices is 6
0 (0): (0, 1) (0, 2)
1 (1): (1, 0) (1, 3)
2 (2): (2, 0) (2, 3) (2, 4)
3 (3): (3, 1) (3, 2) (3, 4) (3, 5)
4 (4): (4, 2) (4, 3) (4, 5)
5 (5): (5, 3) (5, 4)
The graph is connected
Want to see more full solutions like this?
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- a) Write a program that asks user to enter number of vertices in an undirected graph and then the adjacency matrix representing the undirected graph. The program, then, must display whether the given graph is connected or not. You will find two sample runs of the program below. Sample 1 Sample 2 Enter number of vertices: 3 Enter number of vertices: 3 Enter adjacency matrix: 0 1 1 1 0 0 1 0 0 Enter adjacency matrix: 0 1 0 1 0 0 0 0 0 The graph is connected. The graph is not connected.arrow_forwardC++ Coding: Arrays Implement a 4x4 two-dimensional array of integers. Use a nested loop to populate the array with random values 0 to 100 inclusive. Use a nested loop to output the array as a grid. Use a single loop to output all elements in the third row. Use a single loop to output all elements in the first column. Use a single loop to output all elements in the first diagonal (r==c). Use a single loop to output all elements in the opposing diagonal. Example Output: 10 75 67 8820 66 55 4831 57 93 3943 76 81 71Row 3: 31 57 93 39Col 1: 10 20 31 43Diagonal 1: 10 66 93 71Diagonal 2: 88 55 57 43arrow_forwardGroup B Q1) A vector is given by x = [-3.5-5 6.2 11 0 8.1-903-132.5]. Using conditional statements and loops, write a program that creates two vectors from x-one (call it P) that contains the positive elements of x, and a second (call it N) that contains the negative elements of x. In both P and N, the elements are in the same order as in x.arrow_forward
- please code in python Forbidden concepts: arrays/lists (data structures), recursion, custom classes You have been asked to take a small icon that appears on the screen of a smart telephone and scale it up so it looks bigger on a regular computer screen.The icon will be encoded as characters (x and *) in a 3 x 3 grid as follows: (refer image1 ) Write a program that accepts a positive integer scaling factor and outputs the scaled icon. A scaling factor of k means that each character is replaced by a k X k grid consisting only of that character. Input Specification:The input will be an integer such that 0 < k ≤ 10. Output Specification:The output will be 3k lines, which represent each individual line scaled by a factor of k and repeated k times. A line is scaled by a factor of k by replacing each character in the line with k copies of the character. [refer image2]arrow_forward8.7 LAB: File name change A photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names. Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output. Ex: If the input of the program is: ParkPhotos.txt and the contents of ParkPhotos.txt are: Acadia2003_photo.jpg AmericanSamoa1989_photo.jpg BlackCanyonoftheGunnison1983_photo.jpg CarlsbadCaverns2010_photo.jpg CraterLake1996_photo.jpg GrandCanyon1996_photo.jpg IndianaDunes1987_photo.jpg LakeClark2009_photo.jpg…arrow_forwarda) Write a program that asks user to enter number of vertices in a directed graph and thenthe adjacency matrix representing the directed graph. The program, then, must display thenode with the highest outdegree. Assume that nodes are named as 0, 1, 2 and so on.arrow_forward
- Q2) An image is a matrix of pixels. You must have seen two types of images: color andgrayscale. A color image pixel has three colors: Red, Green, and Blue, but a grayscale imagepixel has only one color: Gray. Regardless of the image type, a color always has an 8-bitinteger value (0-255) associated with it. You need to implement a program that can input,create, update and display the images (matrices). It should be able to compute their negatives(matrices) as well. NOTE: You need to generalize the computation of negatives, for we onlyneed to subtract the color values from 255, regardless of the image type.arrow_forwardjava language coding please Object Relationship and File IO Write a program to perform statistical analysis of scores for a class of students. The class may have up to 40 students.There are five quizzes during the term. Each student is identified by a four-digit student ID number. The program is to print the student scores and calculate and print the statistics for each quiz. The output is in the same order as the input; no sorting is needed. The input is to be read from a text file. The output from the program should be similar to the following: Here is some sample data (not to be used) for calculations: Stud Q1 Q2 Q3 Q4 Q5 1234 78 83 87 91 86 2134 67 77 84 82 79 1852 77 89 93 87 71 High Score 78 89 93 91 86 Low Score 67 77 84 82 71 Average 73.4 83.0 88.2 86.6 78.6 The program should print the lowest and highest scores for each quiz. Plan of Attack Learning Objectives You will apply the following topics in this assignment: File Input operations. Working and…arrow_forwardExercise Objectives Problem Description Write a program that reads a string and mirrors it around the middle character. Examples: abcd becomes cdab. abcde becomes deCab AhmadAlami becomes AlamiAhmad Page 1 of 2 Your program must: • Implement function void reflect (char* str) which receives a string (array of characters) and mirrors it. This function does not print anything. • Read from the user (in main()) a string and then print the string after calling function reflect(). • Use pointers and pointer arithmetic only. The use of array notation and/or functions from the string.h library is not allowed.arrow_forward
- change this code to C++ #include <stdio.h> #include <stdlib.h> /** * structure to hold each point */ struct Point3D { float x; float y; float z; }; /** * Parses all the vertices from the path. * @param path The input file path. * @param points The points storage. * @param numPoints The number of points. * @return points Dynamically allocated array of points. * @return The center of mass. */ struct Point3D parseInput(const char *path, struct Point3D **points, int *numPoints) { // to hold each record char f_or_v; float x; float y; float z; // to store the file pointer FILE *inFile; // to iterate the points array int it; // structure to store the center of mass struct Point3D centerOfMass; // opening the file inFile = fopen(path, "r"); // counting the number of points *numPoints = 0; while(!feof(inFile)) { fscanf(inFile, "%c %f %f %f\n", &f_or_v, &x, &y, &z); *numPoints += f_or_v == 'v'; } // dynamically allocating space // for the points *points =…arrow_forwardWhere i need help has multiple parenthesis beside them thank you. def readMatrix(inputfilename): ''' Returns a two-dimentional array created from the data in the given file. Pre: 'inputfilename' is the name of a text file whose first row contains the number of vertices in a graph and whose subsequent rows contain the rows of the adjacency matrix of the graph. ''' # Open the file f = open(inputfilename, 'r') # Read the number of vertices from the first line of the file n = int(f.readline().strip()) # Read the rest of the file stripping off the newline characters and splitting it into # a list of intger values rest = f.read().strip().split() # Create the adjacency matrix adjMat = [] (I need help creating the adjacency matrix)()() ... # Return the matrix return adjMat testFile = input("Enter the name of the input file")graphMatrix = readMatrix(testFile)graphMatrix def Prim(m): ''' Runs Prim's…arrow_forward8.11 LAB: Movie show time display Java Please Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
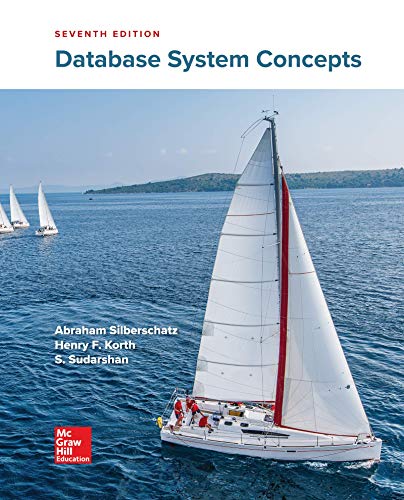
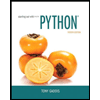
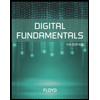
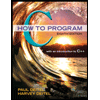
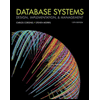
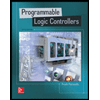