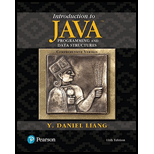
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 28, Problem 28.17PE
Program Plan Intro
Program Plan:
- Include the required import statement.
- Create a package “main”.
- Add a java class named “Edge” to the package which is used to get the edges from the graph.
- Add a java class named “Graph” to the package which is used to add and remove vertices, edges.
- Add a java class named “UnweightedGraph” to the package which is used to store vertices and neighbors.
- Add a java class named “WeightedGraph” to the package which is used to get the weighted edges and print the edges.
- Add a java class named “WeightedEdge” to the package which is used to compare edges.
- Add a java class named “NineTailModel” to the package which is used to compare edges.
- Define the main method using public static main.
- Allocate memory to the class “Test”.
- Define the “Test” class.
- Declare and set the values for the variables.
- Create an object1 for the “Graph” class.
- Display the edge.
- Allocate memory for the “edge” variable.
- Create an object2 for the “Graph” class.
- Display the edge.
- Display the results.
- Define the “Graph” class.
- A graph interface gets created.
- Method to return the size gets defined.
- Method to return the vertices gets defined.
- Method to return the index gets created.
- Method to get the neighbor node gets created.
- Method to get the degree gets created.
- Method to print the edges.
- Method to clear the node gets created.
- Method to add the edges, add vertex gets created.
- Method to remove the vertices gets defined.
- Method for the depth first search gets defined.
- Method for the breadth first search gets defined.
- Create a class “UnweightedGraph”:
- New list for the vertices gets created.
- New list for the neighbor node gets created.
- Create an empty constructor.
- Method to create new graph gets created and adjacency list gets created.
- Method to create an adjacency list gets created.
- Method to return the size of the vertices.
- Method to return the index of the vertices gets defined.
- Method to gets the neighbor node gets defined.
- Method to return the degree of the vertices gets created.
- Method to print the Edges gets created.
- New to clear the graph gets created.
- Method to add vertex gets created.
- Method to add edge gets created.
- Method to perform the depth first search gets defined.
- Method to perform breadth first search gets defined.
- Search tree gets returned.
- Create a class “SearchTree”
- Define the method to return the root.
- Method to return the parent of the vertices
- Method to return the search order gets defined.
- Method to return the number of vertices found gets defined.
- Method to get the path of the vertices gets defined.
- Loop to validate the path gets defined.
- Path gets returned.
- Method to print the path gets defined.
- Method to print the tree gets defined.
- Display the edge.
- Display the root.
- Condition to validate the parent node to display the vertices gets created.
- Define the “getHamiltonianPath” method.
- If the size of graph is equal to “i” value, return a Hamiltonian path from the specified vertex object.
- Otherwise, return null if the graph does not have a Hamiltonian path.
- Define the “getHamiltonianPath” method.
- Return the value using array list.
- Define the “getHamiltonianPath” method.
- Declare the required variables.
- If the length of the graph, indicate no subpath from “i” is found.
- The vertices in the Hamiltonian path are stored in result.
- Reorder the adjacency list for each vertex.
- Create a list for path
- Add vertex to the result list.
- Get the next vertex in the path.
- Return null if no Hamiltonian path is found.
- Define the “reorderNeigborsBasedOnDegree” method.
- Check the condition.
- Find the maximum in the list.
- Check the condition and swap the list with another list.
- Check the condition.
- Define the “allVisited” method.
- Declare the variables.
- Check if all the elements in array is visited and return true.
- Otherwise return the result.
- Define the “getHamiltonianPath” method.
- Declare the variables
- If all the vertices are found then include all the vertices.
- Then search for a Hamiltonian path from “v”.
- Define “getHamiltonianCycle” method.
- Return a Hamiltonian cycle.
- Define “getHamiltonianCycle” method.
- Declare the variable.
- If the length of the graph, indicate no subpath from “i” is found.
- The vertices in the Hamiltonian path are stored in result.
- Reorder the adjacency list for each vertex.
- Create a list for path
- Add vertex to the result list.
- Get the next vertex in the path.
- Return null if no Hamiltonian path is found.
- Define “getHamiltonianCycle” method.
- Declare the variable.
- If all the vertex is visited the return true.
- Otherwise backtrack “v” is marked unvisited.
- Return false.
- Define “isCycle” method.
- Return the value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2.
Q1)
You are given an undirected connected planar graph. There are 10 vertices and 7 faces in the graph. What is the number of edges in the graph?
Note that: A graph is said to be planar if it can be drawn in a plane so that no edge cross.
Q2)
In how many ways can we pick any number of balls from a pack of three different balls?
Q3)
The distance between 2 points A and B is 1320Km. Two cars start moving towards each other with 50 Km/hr and 60 Km/hr. After how many hours do they meet?
[]
[]
dir_euler
Write a function "dir_euler(digraph)" that takes in a connected, directed graph as its input, and then determines whether or not a directed Eulerian circuit exists.
After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs.
print(dir_euler({"A" : ["A", "B", "C"], "B" : ["A"], "C" : ["A"]}),
dir_euler({"A" : ["B", "D"], "B" : ["A", "D"], "C" :
This should return
True False
["C", "D"], "D" : []}))
Python
Python
2. (Begun in class) Write a YES instance of 3-SAT. Then draw the Vertex Cover instance that comes from
your YES instance. What is K (#variables +2*#clauses). Mark the vertices that form the Vertex Cover.
b. Write a NO instance of 3-Sat, and again, draw the graph from this. Mark the vertices that come as
close as possible to being a Vertex Cover.
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (0)URGENT!!!! Please use this graph class and SOLVE FOR ISOLATEDTRIPLETS QUESTION AND AVERAGE BALANCE RATIO Here is the graph class: public class Graph {private final int V;private int E;private final Bag<Integer>[] adj; /*** Create an empty graph with V vertices.*/@SuppressWarnings("unchecked")public Graph(int V) {if (V < 0) throw new Error("Number of vertices must be nonnegative");this.V = V;this.E = 0;this.adj = new Bag[V];for (int v = 0; v < V; v++) {adj[v] = new Bag<>();}} /*** Return the number of vertices in the graph.*/public int V() { return V; } /*** Return the number of edges in the graph.*/public int E() { return E; } /*** Add the undirected edge v-w to graph.* @throws java.lang.IndexOutOfBoundsException unless both 0 <= v < V and 0 <= w < V*/public void addEdge(int v, int w) {if (v < 0 || v >= V) throw new IndexOutOfBoundsException();if (w < 0 || w >= V) throw new IndexOutOfBoundsException();E++;adj[v].add(w);adj[w].add(v);} /***…arrow_forward1. Using the above class map, write function void map::mapToGraph(graph &g){...} to create a graph g that represents the legal moves in the map m. Each vertex should represent a cell, and each edge should represent a legal move between adjacent cells. 2. Write a recursive function findPathRecursive(graph &g, stack &moves) that looks for a path from the start island to the destination island. If a path from the start to the destination exists, your function should call the map::printPath() function that should print a sequence of correct moves (Go left, Go right, Go down, Go up, etc.). If no path from the start to the destination exists, the program should print, "No path exists". If a solution exists the solver should also simulate the solution to each map by calling the map::print() function. The map::print() function prints out a map visualization, with the goal and current position of the car in the map at each move, marked to show the progress. Hint: consider recursive-DFS. 3.…arrow_forwardExercise # 2 - Detecting Cycle between List of N Airports The following graph is an example from Rosen (2011). It shows the flights and distances between some of the major airports in the United States. Dallas 200 1300 200 Austin Washington Denver 1400 Atlanta 160 800 800 Chicago Houston Write a function that takes a list of N airports and checks if they form a cycle of size N. {A cycle is a directed path that starts and ends at the same vertex. Before writing code, make sure you can identify cycles yourself} >> check_cycles (G, ['Austin','Houston', 'Atlanta','Washington','Dallas'l) Yes >>> check_cycles (G, ['Austin', 'Houston','Atlanta','Washington']) No 600 600 780 0000 000L 006arrow_forward
- In this project, you will develop algorithms that find road routes through the bridges to travel between islands. The input is a text file containing data about the given map. Each file begins with the number of rows and columns in the map considered as maximum latitudes and maximum longitudes respectively on the map. The character "X" in the file represents the water that means if a cell contains "X" then the traveler is not allowed to occupy that cell as this car is not drivable on water. The character "0" in the file represents the road connected island. That means if a cell contains "0" then the traveler is allowed to occupy that cell as this car can drive on roads. The traveler starts at the island located at latitude = 0 and longitude = 0 (i.e., (0,0)) in the upper left comer, and the goal is to drive to the island located at (MaxLattitude-1, MaxLongitudes-1) in the lower right corner. A legal move from an island is to move left, right, up, or down to an immediately adjacent cell…arrow_forward8. Graph traversalarrow_forwardTranscribed Image Text Elon Musk is running the graph construction business. A client has asked for a special graph. A graph is called special if it satisfies the following properties: • It has <105 vertices. It is a simple, undirected, connected 3-regular graph. It has exactly k bridge edges, (k given as input). For a graph G, define f(G) to be the minimum number of edges to be removed from it to make it bipartite. The client doesn't like graphs with a high value of f, so you have to minimize it. If there doesn't exist any special graph, print –1. Otherwise, find a special graph G with the minimum possible value of f(G) and also find a subset of its edges of size f(G) whose removal makes it bipartite. In case there are multiple such graphs, you can output any of those. Elon has assigned this task to you, now vou have to develon a C++ code that takes bridge edges as innut and print all the possible granhsarrow_forward
- *Discrete Math In the graph above, let ε = {2, 3}, Let G−ε be the graph that is obtained from G by deleting the edge {2,3}. Let G∗ be the graph that is obtain from G − ε by merging 2 and 3 into a single vertex w. (As in the notes, v is adjacent to w in the new if and only if either {2,v} or {3,v is an edge of G.) (a) Draw G − ε and calculate its chromatic polynomial. (b) Give an example of a vertex coloring that is proper for G − ε, but not for G. (c) Explain, in own words, why no coloring can be proper for G but not proper for G − ε. (d) Draw G∗ and calculate its chromatic polynomial. (e) Verify that, for this example,PG(k) = PG−ε(k) − PG∗ (k).arrow_forward"""1.In a directed graph, a strongly connected component is a set of vertices suchthat for any pairs of vertices u and v there exists a path (u-...-v) thatconnects them. A graph is strongly connected if it is a single stronglyconnected component.""" from collections import defaultdict class Graph: """ A directed graph where edges are one-way (a two-way edge can be represented by using two edges). """ def __init__(self,vertex_count): """ Create a new graph with vertex_count vertices. """ self.vertex_count = vertex_count self.graph = defaultdict(list) def add_edge(self,source,target): """ Add an edge going from source to target """ self.graph[source].append(target) def dfs(self): """ Determine if all nodes are reachable from node 0 """ visited = [False] * self.vertex_count self.dfs_util(0,visited) if visited == [True]*self.vertex_count: return True…arrow_forward5. Icosian Game A century after Euler's discovery (see Problem 4), another famous puzzle-this one invented by the renowned Irish mathematician Sir William Hamilton (1805–1865)-was presented to the world under the name of the Icosian Game. The game's board was a circular wooden board on which the following graph was carved: Find a Hamiltonian circuit-a path that visits all the graph's vertices exactly once before returning to the starting vertex-for this graph.arrow_forward
- Give example of ArrayListarrow_forwardConsider the following function:int mystery(NodeInt32* node){int counter = 0; while (node != NULL) {counter++;node = node->next; } return counter;}(a)The mystery function is recursive.A. True B. Falsearrow_forwardPart 2) Adjacency-matrix graph representation: Java implementation Adjacency-list graph representation: Java implementation Create two classes Graph, one for each representation. Add variable members, constructors, addEdge, searchEdge (given two nodes return true if adjacent or false otherwise), and Iterable (iterates through adjacent nodes).arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
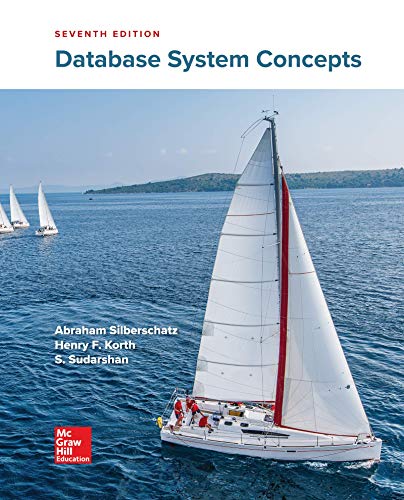
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
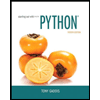
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
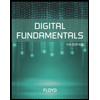
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
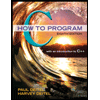
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
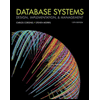
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
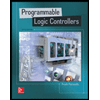
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education