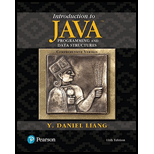
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 28, Problem 28.10PE
Program Plan Intro
Find the shortest path
Program Plan:
Exercise.java:
- Import the required packages.
- Create a class “Exercise”:
- Define the main method
- Prompt user to enter the file.
- Validate whether the file exists or not.
- Prompt user to enter the vertices.
- Loop that read the contents of the file.
- The token that are present in the file are removed.
- Add the edges and vertices of the graph into the list.
- New graph gets created
- Perform the breadth first search for the graph.
- Display the path of the graph.
- Define the main method
UnweightedGraph.java:
- Import the required packages.
- Create a class “UnweightedGraph”:
- New list for the vertices gets created.
- New list for the neighbor node gets created.
- Create an empty constructor.
- Method to create new graph gets created and adjacency list gets created.
- Method to create an adjacency list gets created.
- Method to return the size of the vertices.
- Method to return the index of the vertices gets defined.
- Method to gets the neighbor node gets defined.
- Method to return the degree of the vertices gets created.
- Method to print the Edges gets created.
- New to clear the graph gets created.
- Method to add vertex gets created.
- Method to add edge gets created.
- Method to perform the depth first search gets defined.
- Method to perform breadth first search gets defined.
- Search tree gets returned.
- Create a class “SearchTree”
- Define the method to return the root.
- Method to return the parent of the vertices
- Method to return the search order gets defined.
- Method to return the number of vertices found gets defined.
- Method to get the path of the vertices gets defined.
- Loop to validate the path gets defined.
- Path gets returned.
- Method to print the path gets defined.
- Method to print the tree gets defined.
- Display the edge.
- Display the root.
- Condition to validate the parent node to display the vertices gets created.
Graph.java:
- A graph interface gets created.
- Method to return the size gets defined.
- Method to return the vertices gets defined.
- Method to return the index gets created.
- Method to get the neighbor node gets created.
- Method to get the degree gets created.
- Method to print the edges.
- Method to clear the node gets created.
- Method to add the edges, add vertex gets created.
- Method to remove the vertices gets defined.
- Method for the depth first search gets defined.
- Method for the breadth first search gets defined.
Edge.java
- Create a class “Edge”
- Define and declare the required variables.
- Constructor gets defined.
- Method that defines Boolean objects gets defined.
- Return the value after validating the vertices.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
<<Write in Java>>
- Challenge 7
File encryption is the science of writing the contents of a file in a secret code. Your encryption program should work like a filter, reading the contents of one file, modifying the data into a code, and then writing the coded contents out to a second file. The second file will be a version of the first file, but written in a secret code. Although there are complex encryption techniques, you should come up with a simple one of your own. For example, you could read the first file one character at a time, and add 10 to the character code of each character before it is written to the second file.
- Challenge 8
Write a program that decrypts the file produced by the program in Programming Challenge 7. The decryption program should read the contents of the coded file, restore the data to its original state, and write it to another file
(PYTHON) A photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names.
Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output.
Ex: If the input of the program is:
ParkPhotos.txt
and the contents of ParkPhotos.txt are:
Acadia2003_photo.jpg AmericanSamoa1989_photo.jpg BlackCanyonoftheGunnison1983_photo.jpg CarlsbadCaverns2010_photo.jpg CraterLake1996_photo.jpg GrandCanyon1996_photo.jpg IndianaDunes1987_photo.jpg LakeClark2009_photo.jpg Redwood1980_photo.jpg…
JAVA PPROGRAM
Write a program that prompts the user to enter a file name, then opens the file in text mode and reads names. The file contains one name on each line. The program then compares each name with the name that is at the end of the file in a symmetrical position. For example if the file contains 10 names, the name #1 is compared with name #10, name #2 is compared with name #9, and so on. If you find matches you should print the name and the line numbers where the match was found.
While entering the file name, the program should allow the user to type quit to exit the program.
If the file with a given name does not exist, then display a message and allow the user to re-enter the file name.
The file may contain up to 100 names.
You can use an array or ArrayList object of your choosing, however you can only have one array or ArrayList.
Input validation:
a) If the file does not exist, then you should display a message "File 'somefile.txt' is not found." and allow the…
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Knowledge Booster
Similar questions
- (C Language) A photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names. Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output. Assume also the maximum number of characters of all file names is 100.arrow_forwardMerge two files (1 point) Write a program that reads the content of two files “text1.txt” and “text2.txt" line by line and merges them into another file called “merge12.txt". You can safely assume that the input is valid. IN C PROGRAMMINGarrow_forwardC++ Problem!! Sort A List Of Integers Given a file of 100,000 unsorted integers, write a program that first prompt user to enter file name and reads those integers into an array and sorts the integers in the array. The program should then prompt the user for an index, and then display the integer at that index. For example, if the array contains ten integers and the contents are 79 4 42 51 12 22 33 17 91 10 after sorting, the integer at index 6 is 42 Download the "List of 100,000 Unsorted Integers" from below link and sort that list, then enter the value at index 10000 (ten thousand) for a screenshot. https://1drv.ms/t/s!AuFS4mkcuYyBjHZ_SIudb93bq2uy?e=CsyvZjarrow_forward
- 3) Implement the if __name__ == "__main__" : block at the bottom of this file to do the following: 3a) The if main block at the bottom should get the name of the highway graph file from the command line arguments. See the video in Python that explains how to get command line arguments. 3b) Call your parse_highway_graph_file function to parse that file, and to construct a WeightedGraph object from it. 3c) Write some code that outputs (with print statements) the degree of each vertex. You can have one vertex per line, indicating its id (just its 0-based index) followed by its degree. IMPORTANT: Your parse_highway_graph_file function should NOT produce any output. You will have code in your if main block that will have the print statements. The WeightedGraph class has a degree method, inherited from Graph that will return to you the degree of a vertex.arrow_forwardPython programming: Topic: Compresion - using functional function Write a program that prints the number of palindromes in this file ..../englishWords (tips , open file first then read the content before printing)arrow_forward(21) 2. Write a Java application that asks the user to enter a car model or 'done' when finished. If the user enters a car model, ask the user to enter the color of that car model. Store the cars and colors in ArrayLists of type String. When the while loop completes, use a for loop to print out the car and color as shown below: Enter a car or 'done if done: Camry Enter color of car: White Enter a car or 'done' if done: Accord Enter color of car: Blue Enter a car or 'done' if done: done car: Camry, color: White car: Accord, color: Bluearrow_forward
- *Code in python Write a program that allows the user to navigate the lines of text in a file. The program should prompt the user for a filename and input the lines of text into a list. The program then enters a loop in which it prints the number of lines in the file and prompts the user for a line number. Actual line numbers range from 1 to the number of lines in the file. If the input is 0, the program quits. Otherwise, the program prints the line associated with that number. An example file and the program input and output is shown below: example.txt Line 1.Line 2.Line 3. Enter the input file name: example.txt The file has 3 lines. Enter a line number [0 to quit]: 2 2 : Line 2. The file has 3 lines. Enter a line number [0 to quit]: 4 ERROR: line number must be less than 3. The file has 3 lines. Enter a line number [0 to quit]: 0 Make sure the program gracefully handles a user entering a line number that is too high.arrow_forwardDoubly Linked List (C Language, without headers) This assignment asks you to sort the letters in an input file and print the sorted letters to an output file (or standard output) which will be the solution. Your program, called codesolve, will take the following command line arguments: % codesolve [-o output_file_name] input_file_name Read the letters in from the input file and convert them to upper case if they are not already in uppercase. If the output_file_name is given with the -o option, the program will output the sorted letters to the given output file; otherwise, the output shall be to standard output. In addition to parsing and processing the command line arguments, your program needs to do the following: You need to construct a doubly linked list as you read from input. Each node in the list will link to the one in front of it and the one behind it if they exist. They will have NULL for the previous pointer if they are first in the list. They will have NULL for the next…arrow_forward*Code in Python Write a program that inputs a text file. The program should print the unique words in the file in alphabetical order. Uppercase words should take precedence over lowercase words. For example, 'Z' comes before 'a'. The input file can contain one or more sentences, or be a multiline list of words. An example input file is shown below: example.txt the quick brown fox jumps over the lazy dog An example of the program's output is shown below: Enter the input file name: example.txt brown dog fox jumps lazy over quick thearrow_forward
- *Code in Python A file concordance tracks the unique words in a file and their frequencies. Write a program that displays a concordance for a file. The program should output the unique words and their frequencies in alphabetical order. Variations are to track sequences of two words and their frequencies, or n words and their frequencies. Below is an example file along with the program input and output: example.txt I AM SAM I AM SAM SAM I AM Enter the input file name: example.txt AM 3 I 3 SAM 3 The program should handle input files of varying length (lines).arrow_forward*Code in Python Write a script named dif.py. This script should prompt the user for the names of two text files and compare the contents of the two files to see if they are the same. If they are, the script should simply output "Yes". If they are not, the script should output "No", followed by the first lines of each file that differ from each other. The input loop should read and compare lines from each file, including whitespace and punctuation. The loop should break as soon as a pair of different lines is found. Below are examples of the program input and output along with relevant files: one.txt: This is text. abc 123 sameAsOne.txt: This is text. abc 123 differentFromOne.txt This is text. xyz 123 Comparing files that are the same: Enter the first file name: one.txt Enter the second file name: sameAsOne.txt Yes Comparing files that are different: Enter the first file name: one.txt Enter the second file name: differentFromOne.txt No abc xyzarrow_forwardTopical Information Use C++. This lab is designed to give you practice with labeled data in files. Again, classes can help ease your design woes. Program Information Write a program that transfers the contents of one file to a second file. The first file will contain an arbitrary (unknown) number of data groups. A data group will normally consist of a person's name (a string containing spaces), the person's student ID (a large integer), the person's GPA (a floating point number), and the person's gender (a character). The data in each group should be in a labeled data format. Be sure to use some kind of string library to help you with the label/value processing (as we discussed in class). (The library provided here is good but not everything you'll need. You still need functions to convert strings into integers and into floating point numbers. string to bool, string to string, and string to character conversions should be fairly easy.) You can choose the actual values to use for the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
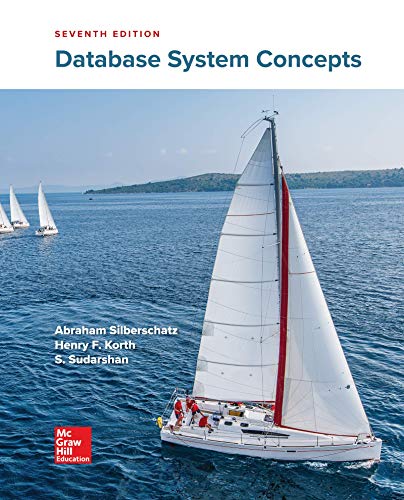
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
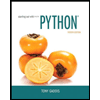
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
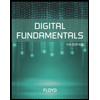
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
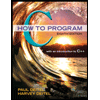
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
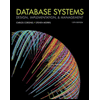
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
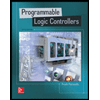
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education