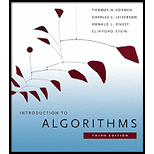
Introduction to Algorithms
3rd Edition
ISBN: 9780262033848
Author: Thomas H. Cormen, Ronald L. Rivest, Charles E. Leiserson, Clifford Stein
Publisher: MIT Press
expand_more
expand_more
format_list_bulleted
Question
Chapter 24.1, Problem 3E
Program Plan Intro
To suggest a simple change to the BELLMAN-FORD
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We recollect that Kruskal's Algorithm is used to find the minimum spanning tree in a weighted graph. Given a weighted undirected graph G = (V , E, W), with n vertices/nodes, the algorithm will first sort the edges in E according to their weights. It will then select (n-1) edges with smallest weights that do not form a cycle. (A cycle in a graph is a path along the edges of a graph that starts at a node and ends at the same node after visiting at least one other node and not traversing any of the edges more than once.)
Use Kruskal's Algorithm to nd the weight of the minimum spanning tree for the following graph.
You are given a weighted, undirected graph G = (V, E) which is guaranteed to be connected.
Design an algorithm which runs in O(V E + V 2 log V ) time and determines which of the edges appear in all minimum spanning trees of G.
Do not write the code, give steps and methods. Explain the steps of algorithm, and the logic behind these steps in plain English.Please give total time complexity.
test whether a particular edge appears in all MSTs
You are given a weighted, undirected graph G = (V, E) which is guaranteed to be connected.
Design an algorithm which runs in O(V E + V 2 log V ) time and determines which of the edges appear in all minimum spanning trees of G.
Do not write the code, give steps and methods. Explain the steps of algorithm, and the logic behind these steps in plain English
Chapter 24 Solutions
Introduction to Algorithms
Ch. 24.1 - Prob. 1ECh. 24.1 - Prob. 2ECh. 24.1 - Prob. 3ECh. 24.1 - Prob. 4ECh. 24.1 - Prob. 5ECh. 24.1 - Prob. 6ECh. 24.2 - Prob. 1ECh. 24.2 - Prob. 2ECh. 24.2 - Prob. 3ECh. 24.2 - Prob. 4E
Ch. 24.3 - Prob. 1ECh. 24.3 - Prob. 2ECh. 24.3 - Prob. 3ECh. 24.3 - Prob. 4ECh. 24.3 - Prob. 5ECh. 24.3 - Prob. 6ECh. 24.3 - Prob. 7ECh. 24.3 - Prob. 8ECh. 24.3 - Prob. 9ECh. 24.3 - Prob. 10ECh. 24.4 - Prob. 1ECh. 24.4 - Prob. 2ECh. 24.4 - Prob. 3ECh. 24.4 - Prob. 4ECh. 24.4 - Prob. 5ECh. 24.4 - Prob. 6ECh. 24.4 - Prob. 7ECh. 24.4 - Prob. 8ECh. 24.4 - Prob. 9ECh. 24.4 - Prob. 10ECh. 24.4 - Prob. 11ECh. 24.4 - Prob. 12ECh. 24.5 - Prob. 1ECh. 24.5 - Prob. 2ECh. 24.5 - Prob. 3ECh. 24.5 - Prob. 4ECh. 24.5 - Prob. 5ECh. 24.5 - Prob. 6ECh. 24.5 - Prob. 7ECh. 24.5 - Prob. 8ECh. 24 - Prob. 1PCh. 24 - Prob. 2PCh. 24 - Prob. 3PCh. 24 - Prob. 4PCh. 24 - Prob. 5PCh. 24 - Prob. 6P
Knowledge Booster
Similar questions
- Write a pseudocode to find all pairs shortest paths using the technique used in Bellman-Ford's algorithm so that it will produce the same matrices like Floyd-Warshall algorithm produces. Also provide the algorithm to print the paths for a source vertex and a destination vertex. For the pseudocode consider the following definition of the graph - Given a weighted directed graph, G = (V, E) with a weight function wthat maps edges to real-valued weights. w(u, v) denotes the weight of an edge (u, v). Assume vertices are labeled using numbers from1 to n if there are n vertices.arrow_forwardLet G be a directed acyclic graph. You would like to know if graph G contains directed path that goes through every vertex exactly once. Give an algorithm that tests this property. Provide justification of the correctness and analyze running time complexity of your algorithm. Your algorithm must have a running time in O(|V | + |E|). Detailed pseudocode is required.arrow_forwardI need the algorithm, proof of correctness and runtime analysis for the problem. No code necessary ONLY algorithm. And runtime should be O((n+m)*T).arrow_forward
- I need the algorithm, proof of correctness and runtime analysis for the problem. No code necessary ONLY algorithm. And runtime should be O(n + m) as stated in the question.arrow_forwardA directed graph G = (V, E) and two vertices, s and t, are supplied. Additionally, the graph's edges are blue or red. Finding a path from s to t with all red edges after all blue edges is your goal. If the route has red or blue borders, they should show after the blue edges. Develop and test an algorithm that solves this problem in O(n + m) time?arrow_forwardAlgorithmsarrow_forward
- Let G be a directed weighted graph with n vertices and m edges such that the edges in G have positive weights. Let (u, v) be an edge in G. By a shortest cycle containing edge (u, v) we mean a cycle containing (u, v) that is of minimum weight, among all cycles containing (u, v) (if such a cycle exists). Give an O(mlgn)-time algorithm that computes a shortest cycle in G containing the edge (u, v), or reports that no cycle containing edge (u, v) exists.arrow_forwardConsider the following graph. We are finding the lengths of the shortest paths from vertex a to all vertices by the Relaxation algorithm from the lecture: At the beginning, every vertex v is set as unmarked and h(v) = +∞. Then a is set as open and h(a) = 0. How many ways there are to select five (at that moment) open vertices so that after their relaxation (and closing) the value of h(v) will represent the shortest path length from a to every v in the graph? Specify the number of ways and one such way separated by commas and spaces, for example 25, b, а, с, а, d. d 3 1 a 1 -4 -4arrow_forwardProvide an eficient algorithm that given a directed graph G with n vertices and m edges as input, finds the outdegree of each vertex in G. Note that outdegree of a vertex u is the number of edges directed from u to some other vertex v. Discuss the running-time of your algorithm and Provide an algorithm that given a directed graph G with n vertices and m edges as input, nds the indegree of each vertex in G. Note that indegree of a vertex u is the number of edges directed into u from some other vertex v. Discuss the running-time of your algorithm.arrow_forward
- You are organizing a programming competition, where contestants implement Dijkstra's algorithm. Given adirected graph G = (V, E) with integer-weight edges and a starting vertex s ∈ V , their programs are supposedto output triplets (v, v.d, v.π) for each vertex v ∈ V . Design an O(V +E) time algorithm that takes as inputthe original graph G in both adjacency matrix (G.M) and adjacency list (G.Adj) representations, startingvertex s, and the output of a contestant's program (given as an array A of triplets), and returns whetherA is the correct output for G. Write down the pseudocode for your algorithm, explain why it correctlyveries the output, and analyze your algorithm's running time. You may assume that all edge weights of the input graph provided to the contestantsare nonnegative and A (the output of their programs) is in the valid format, i.e., you don't need to verifythat A is actually an array of triplets, with v and v.π being valid vertices and v.d being an integer.Can you…arrow_forwardWe are given an undirected graph, that is connected. We're also given that each edge is associated with a positive weight. Now, we want to make this graph acyclic by removing some edges. Find this set of edges by designing an algorithm. Note: this edge set should have the smallest total weight out of other potential edge set solutions. The algorithm should run in O((m + n)log n) time.arrow_forward........arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
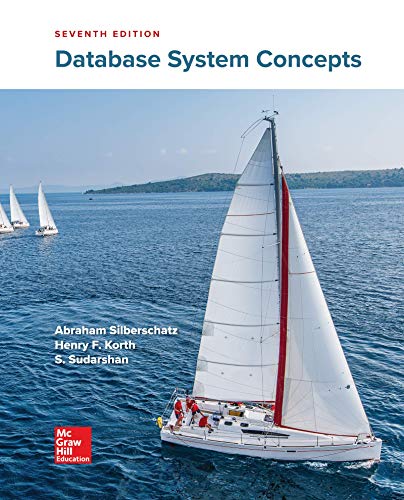
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
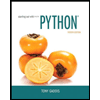
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
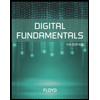
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
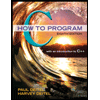
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
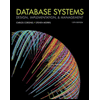
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
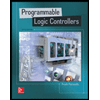
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education