*System Programming (C language) Add to the previous shell the following features: Input file redirection using < Output file redirection, both truncating (>) and appending (>>) #include #include #include #include #include #include #define MAX_INPUT_LENGTH 1024 #define MAX_ARG_LENGTH 128 void execute_command(char *args[]) { pid_t pid = fork(); if (pid == 0) { // Child process if (execvp(args[0], args) == -1) { perror("Shell"); } exit(EXIT_FAILURE); } else if (pid < 0) { // Forking failed perror("Shell"); } else { // Parent process waitpid(pid, NULL, 0); } } int main() { char input[MAX_INPUT_LENGTH]; char *args[MAX_ARG_LENGTH]; char *token; while (1) { printf("MyShell> "); fgets(input, sizeof(input), stdin); // Remove newline character input[strlen(input) - 1] = '\0'; // Tokenize the input token = strtok(input, " "); int i = 0; while (token != NULL) { args[i++] = token; token = strtok(NULL, " "); } args[i] = NULL; // Set the last element to NULL // Handling built-in commands if (args[0] != NULL) { if (strcmp(args[0], "cd") == 0) { if (args[1] != NULL) { if (chdir(args[1]) != 0) { perror("cd"); } } else { fprintf(stderr, "cd: missing argument\n"); } } else if (strcmp(args[0], "exit") == 0) { exit(0); } else { // Execute other commands execute_command(args); } }
*System Programming (C language)
Add to the previous shell the following features:
- Input file redirection using <
- Output file redirection, both truncating (>) and appending (>>)
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#define MAX_INPUT_LENGTH 1024
#define MAX_ARG_LENGTH 128
void execute_command(char *args[]) {
pid_t pid = fork();
if (pid == 0) {
// Child process
if (execvp(args[0], args) == -1) {
perror("Shell");
}
exit(EXIT_FAILURE);
} else if (pid < 0) {
// Forking failed
perror("Shell");
} else {
// Parent process
waitpid(pid, NULL, 0);
}
}
int main() {
char input[MAX_INPUT_LENGTH];
char *args[MAX_ARG_LENGTH];
char *token;
while (1) {
printf("MyShell> ");
fgets(input, sizeof(input), stdin);
// Remove newline character
input[strlen(input) - 1] = '\0';
// Tokenize the input
token = strtok(input, " ");
int i = 0;
while (token != NULL) {
args[i++] = token;
token = strtok(NULL, " ");
}
args[i] = NULL; // Set the last element to NULL
// Handling built-in commands
if (args[0] != NULL) {
if (strcmp(args[0], "cd") == 0) {
if (args[1] != NULL) {
if (chdir(args[1]) != 0) {
perror("cd");
}
} else {
fprintf(stderr, "cd: missing argument\n");
}
} else if (strcmp(args[0], "exit") == 0) {
exit(0);
} else {
// Execute other commands
execute_command(args);
}
}

Step by step
Solved in 4 steps with 3 images

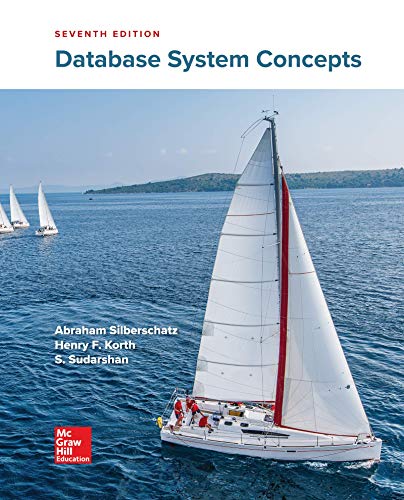
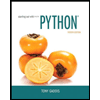
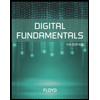
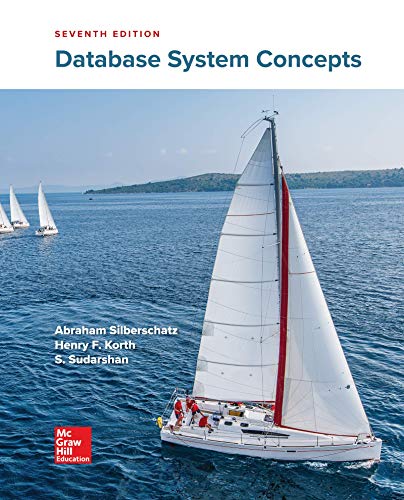
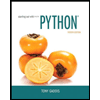
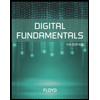
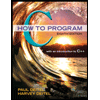
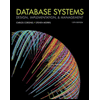
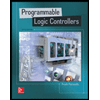